Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial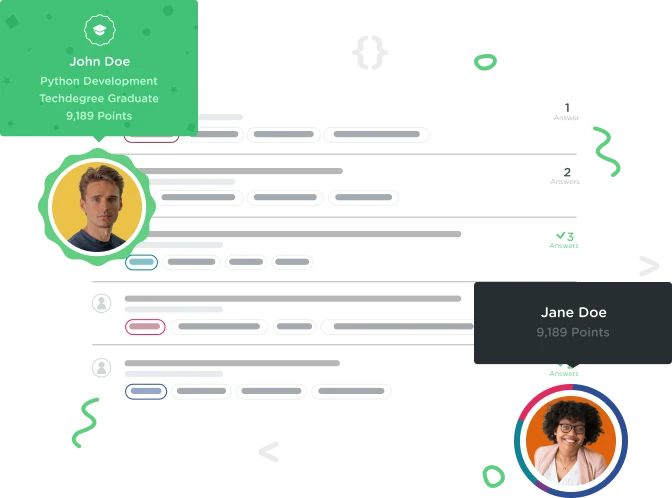

Artur Ferfecki
5,950 PointsWhy can't I use or to not repeat if statement?
Hello pythonists!
I know how to solve the assignment (or at least I think I know) but I was trying to reduce the amount of code lines by checking all the vowels in one line using or logical operator and still only the first condition is checked ('a' in my case). Can someone please give me a hand how to adjust the code so I don't have to repeat if statement multiple times?
def disemvowel(word):
new_list = []
word = word.lower()
for i in word:
if i != 'a' or 'e' or 'i' or 'o' or 'u':
new_list.append(i)
word = ''.join(new_list)
return word
1 Answer
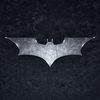
Logan R
22,989 PointsHello!
So you have 3 issues with your code:
The first issue is that the final output is case sensitive. That means that you cannot just simply call word = word.lower()
before the while loop. This will make your final output all lowercase.
The second issue is the comparisons.
if i != 'a' or 'e':
The !=
does not distribute across multiple comparisons. This means the above statement says "1. if the current letter is not 'a' or 2. if 'e', then follow through with if statement. if 'e'
will always be true, so the if block will always run. You need to compare the current letter against each vowel.
IE:
if i != 'a' or i != 'e':
The final problem is the use of OR. By having all of your statements ORs, you are saying if ANY of those are true, go ahead and run the if block.
IE:
current_letter = 'a'
if current_letter != 'a' or current_letter != 'o':
# While the current letter is an 'a', it's not an 'o', so this program will print out "HELLO".
print ("HELLO")
What you want to do is print out only if ALL of the comparisons are true.
Hopefully, this is helpful. It's not one of my best explanations (sorry about that). Feel free to leave a comment about anything that isn't clear and I can try to clear it up!
Thanks!
Artur Ferfecki
5,950 PointsArtur Ferfecki
5,950 PointsHi Logan,
Thanks for your explanation!
1) yeah I discovered when submitting the code, I misunderstood a bit the assignment. 2) ok, understand 3) yes got it, in this case should be used AND right?
All the best, Artur
Logan R
22,989 PointsLogan R
22,989 PointsYep! You should use the
and
keyword.