Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial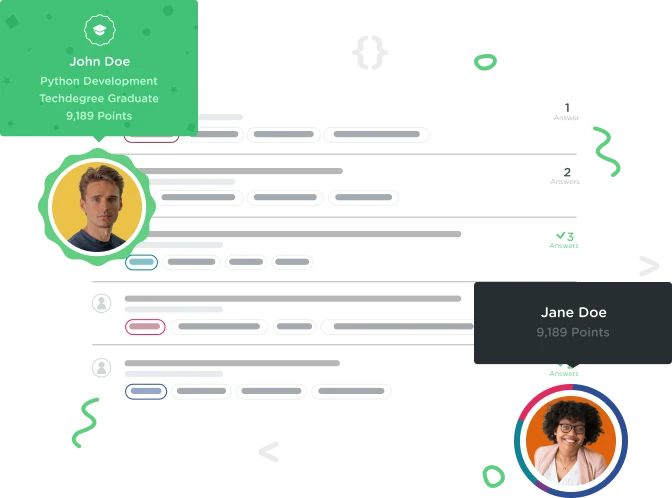
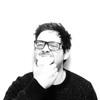
Adrien Dubois
4,862 PointsWhy do I have to put the "document.write(html);" outside the for loop and not inside?
The correct way to display it 100 times, for instance is the following:
for ( var i = 1; i <= 100; i += 1 ){
red = Math.floor(Math.random() * 256 );
green = Math.floor(Math.random() * 256 );
blue = Math.floor(Math.random() * 256 );
rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
html += '<div style="background-color:' + rgbColor + '"></div>';
}
document.write(html);
If I write it like as below, which for me should be correct, it is displayed to many times:
for ( var i = 1; i <= 100; i += 1 ){
red = Math.floor(Math.random() * 256 );
green = Math.floor(Math.random() * 256 );
blue = Math.floor(Math.random() * 256 );
rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
html += '<div style="background-color:' + rgbColor + '"></div>';
document.write(html);
}
Edited for better readability - Dane E. Parchment Jr. (Moderator)
3 Answers

Anjali Pasupathy
28,883 PointsThe for loop adds a div element to the variable html in every iteration. When the for loop has run 100 times, there are 100 div elements stored in html. Thus, after the for loop, when you use the line "document.write(html)", you're writing 100 div elements to the document.
If you put "document.write(html)" inside the for loop, then you're writing html to the document after adding a div element to html in each iteration. Thus, on the 1st iteration, you're adding 1 div element to the document; on the 2nd iteration, you're adding 2 div elements; on the 3rd, 3 div elements. This continues until you get to the 100th iteration, where you're adding 100 div elements to the doc. This means that, after the for loop has run its course, you've added 1+2+3+4+...+98+99+100 div elements to the document. This gives you 5050 div elements rather than the 100 div elements you wanted. If you look closely at those div elements, you'll see that they're all the same 100 colors: the 1st color is repeated 100 times, the 2nd color 99 times, the 3rd 98 times, etc.
If you want to put "document.write(html)" inside the for loop without printing 5050 divs, you need to remove the "+" symbol from the line immediately before it:
html = '<div style="background-color:' + rgbColor + '"></div>';
I hope this helps!
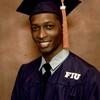
Dane Parchment
Treehouse Moderator 11,077 PointsOk, you are not wrong to believe that placing the document.write in the loop would be correct. However, you need to take a look at context. The value being written to the document.write, is a concatenated string with the values being appended to it. This string forms a div, with a random background color.
If you were to place the document.write within the loop you would loop 100 times that is true, but you would also be displaying the previous iterations with it, making it unorganized:
Loop 1: <div style="background-color: rgb(0, 200, 0)"></div>
Loop 2: <div style="background-color: rgb(0, 200, 0)"></div>
<div style="background-color: rgb(1, 47, 230)"></div>
Loop 3: <div style="background-color: rgb(0, 200, 0)"></div>
<div style="background-color: rgb(1, 47, 230)"></div>
<div style="background-color: rgb(86,33, 72)"></div>
...100 times
See the problem now we have now created much more divs than necessary and all of them are just repeats of the previous n - 1 (n being the number of loops) iterations.
By placing the document.write after we would get:
Loop 1: result appended to html
Loop 2: result appended to html
Loop 3: result appended to html
document.write: <div style="background-color: rgb(0, 200, 0)"></div>
<div style="background-color: rgb(1, 47, 230)"></div>
<div style="background-color: rgb(86,33, 72)"></div>
Hopefully this answers your question.
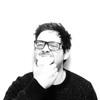
Adrien Dubois
4,862 PointsMany thanks for your answers! Actually, as part of the cod e was already written I didn't really check or see the HTML +=... line. Feel a bit stupid now :p
Thank you again guys!

Anjali Pasupathy
28,883 PointsYou're welcome! And you're not stupid - it's a pretty easy mistake to make and miss, especially if you've been staring at the same code for a while. (: