Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial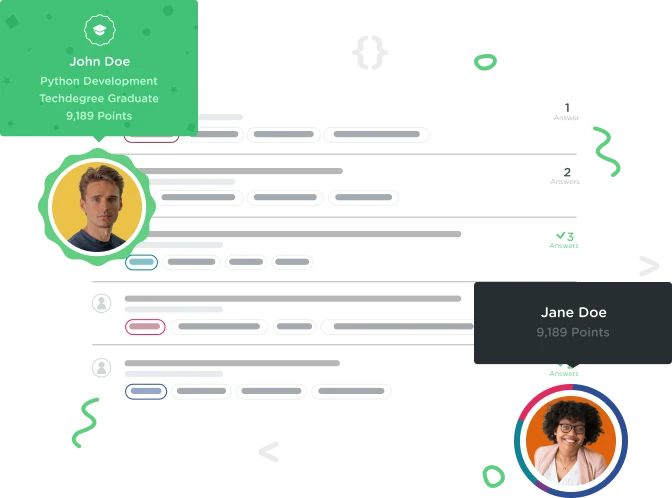

Christina Campbell
12,098 PointsWhy do the transitions no longer work?
When I initially entered the transitions they worked fine. When more code was added the lyrics no longer show. Only the animations.
Here is my code:
public class AlbumDetailActivity extends Activity {
public static final String EXTRA_ALBUM_ART_RESID = "EXTRA_ALBUM_ART_RESID";
@Bind(R.id.album_art) ImageView albumArtView;
@Bind(R.id.fab) ImageButton fab;
@Bind(R.id.title_panel) ViewGroup titlePanel;
@Bind(R.id.track_panel) ViewGroup trackPanel;
@Bind(R.id.detail_container) ViewGroup detailContainer;
private TransitionManager mTransitionManager;
private Scene mExpandedScene;
private Scene mCollapsedScene;
private Scene mCurrentScene;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_album_detail);
ButterKnife.bind(this);
populate();
setupTransitions();
}
private void animate() {
// ObjectAnimator scalex = ObjectAnimator.ofFloat(fab, "scaleX", 0, 1); // ObjectAnimator scaley = ObjectAnimator.ofFloat(fab, "scaleY", 0, 1); // AnimatorSet scaleFab = new AnimatorSet(); // scaleFab.playTogether(scalex, scaley); Animator scaleFab = AnimatorInflater.loadAnimator(this, R.animator.scale); scaleFab.setTarget(fab);
int titleStartValue = titlePanel.getTop();
int titleEndValue = titlePanel.getBottom();
ObjectAnimator animatorTitle = ObjectAnimator.ofInt(titlePanel, "bottom", titleStartValue, titleEndValue);
animatorTitle.setInterpolator(new AccelerateInterpolator());
int trackStartValue = trackPanel.getTop();
int trackEndValue = trackPanel.getBottom();
ObjectAnimator animatorTrack = ObjectAnimator.ofInt(trackPanel, "bottom", trackStartValue, trackEndValue);
animatorTrack.setInterpolator(new DecelerateInterpolator());
titlePanel.setBottom(titleStartValue);
trackPanel.setBottom(titleStartValue);
fab.setScaleX(0);
fab.setScaleY(0);
// animatorTitle.setDuration(1000); // animatorTrack.setDuration(1000); // animatorTitle.setStartDelay(1000);
AnimatorSet set = new AnimatorSet();
set.playSequentially(animatorTitle, animatorTrack, scaleFab);
set.start();
}
@OnClick(R.id.album_art)
public void onAlbumArtClick(View view) { animate(); }
@OnClick(R.id.track_panel)
public void onTrackPanelClicked(View view) {
if (mCurrentScene == mExpandedScene) {
mCurrentScene = mCollapsedScene;
}
else {
mCurrentScene = mExpandedScene;
}
mTransitionManager.transitionTo(mCurrentScene);
}
private void setupTransitions() {
mTransitionManager = new TransitionManager();
ViewGroup transitionRoot = detailContainer;
//Expanded scene
mExpandedScene = Scene.getSceneForLayout(transitionRoot,
R.layout.activity_album_detail_expanded, this);
mExpandedScene.setEnterAction(new Runnable() {
@Override
public void run() {
ButterKnife.bind(AlbumDetailActivity.this);
populate();
mCurrentScene = mExpandedScene;
}
});
TransitionSet expandTransitionSet = new TransitionSet();
expandTransitionSet.setOrdering(TransitionSet.ORDERING_SEQUENTIAL);
ChangeBounds changeBounds = new ChangeBounds();
changeBounds.setDuration(200);
expandTransitionSet.addTransition(changeBounds);
Fade fadeLyrics = new Fade();
fadeLyrics.addTarget(R.id.lyrics);
fadeLyrics.setDuration(150);
expandTransitionSet.addTransition(fadeLyrics);
//Collapsed scene
mCollapsedScene = Scene.getSceneForLayout(transitionRoot,
R.layout.activity_album_detail, this);
mCollapsedScene.setEnterAction(new Runnable() {
@Override
public void run() {
ButterKnife.bind(AlbumDetailActivity.this);
populate();
mCurrentScene = mCollapsedScene;
}
});
TransitionSet collapseTransitionSet = new TransitionSet();
collapseTransitionSet.setOrdering(TransitionSet.ORDERING_SEQUENTIAL);
Fade fadeOutLyrics = new Fade();
fadeOutLyrics.addTarget(R.id.lyrics);
fadeOutLyrics.setDuration(150);
collapseTransitionSet.addTransition(fadeOutLyrics);
ChangeBounds resetBounds = new ChangeBounds();
resetBounds.setDuration(200);
collapseTransitionSet.addTransition(resetBounds);
mTransitionManager.setTransition(mExpandedScene, mCollapsedScene, collapseTransitionSet);
mTransitionManager.setTransition(mCollapsedScene, mExpandedScene, expandTransitionSet);
mCollapsedScene.enter();
}
private void populate() {
int albumArtResId = getIntent().getIntExtra(EXTRA_ALBUM_ART_RESID, R.drawable.mean_something_kinder_than_wolves);
albumArtView.setImageResource(albumArtResId);
Bitmap albumBitmap = getReducedBitmap(albumArtResId);
colorizeFromImage(albumBitmap);
}
private Bitmap getReducedBitmap(int albumArtResId) {
// reduce image size in memory to avoid memory errors
final BitmapFactory.Options options = new BitmapFactory.Options();
options.inJustDecodeBounds = false;
options.inSampleSize = 8;
return BitmapFactory.decodeResource(getResources(), albumArtResId, options);
}
private void colorizeFromImage(Bitmap image) {
Palette palette = Palette.from(image).generate();
// set panel colors
int defaultPanelColor = 0xFF808080;
int defaultFabColor = 0xFFEEEEEE;
titlePanel.setBackgroundColor(palette.getDarkVibrantColor(defaultPanelColor));
trackPanel.setBackgroundColor(palette.getLightMutedColor(defaultPanelColor));
// set fab colors
int[][] states = new int[][]{
new int[]{android.R.attr.state_enabled},
new int[]{android.R.attr.state_pressed}
};
int[] colors = new int[]{
palette.getVibrantColor(defaultFabColor),
palette.getLightVibrantColor(defaultFabColor)
};
fab.setBackgroundTintList(new ColorStateList(states, colors));
}
}