Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial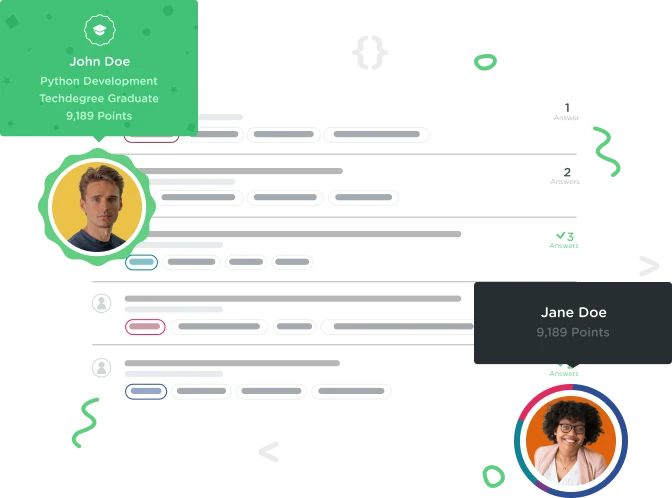
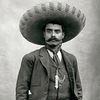
Keith Corona
9,553 PointsWhy do we add the else statement in the while loop?
Hello all,
I have one question regarding the "else" portion of my code to print a message when there is no student to be found.
For a long while I had added my "else" statement in the for loop and continued to get the no student found message even when entering a listed student's name. I moved the "else" statement to where it is in the code below (in the while statement) and now the code works great. Why?
I thought that if the search didn't find the student's name, it would be best to return the no student found message. I don't get why the "else" statement works only in the while loop.
while (true) {
search = prompt('Search student records: type a name [Jody] (or type "quit" to end)');
if (search === null || search.toLowerCase() === 'quit') {
break;
} else {
print(noStudent);
}
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if ( search.toLowerCase() === student.name.toLowerCase() ) {
message = getStudentReport( student );
print(message);
}
}
Full code below:
var message = '';
var noStudent = 'No student found by that name';
var student;
var search;
function print (message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport( student ) {
var report = '<h2>Name: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
report += '<p>Points: ' + student.points + '</p>';
return report;
}
while (true) {
search = prompt('Search student records: type a name [Jody] (or type "quit" to end)');
if (search === null || search.toLowerCase() === 'quit') {
break;
} else {
print(noStudent);
}
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if ( search.toLowerCase() === student.name.toLowerCase() ) {
message = getStudentReport( student );
print(message);
}
}
}
3 Answers
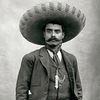
Keith Corona
9,553 PointsUpdate:
For some reason, this morning the code works fine in both ways. Thank you to all who chimed in, I don't know what it malfunctioned last night, but it is all good now.

chrisverra
3,760 PointsThat would work just fine, just add a String value to noStudent :)
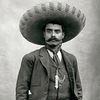
Keith Corona
9,553 PointsIn my original question post I had made the var noStudent = 'No student found by that name';
, wouldn't this call it?
Each time I have tried putting the else
keyword in the second if condition (inside the for loop), whatever name I enter in the prompt return the 'No student found by that name' result instead of posting the data for a student who should be found.
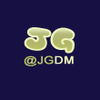
Jonathan Grieve
Treehouse Moderator 91,254 PointsThe else
is actually part of the condition statement inside.
Else
is a keyword designed to match all other possible conditions and works best with the elseif
keyword. If all other conditions in an else statement are not met the code in the else
keyword is run. :-)
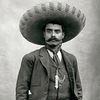
Keith Corona
9,553 PointsThank you for your response. So in the code here, why would adding the else
keyword not run the code correctly?
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if ( search.toLowerCase() === student.name.toLowerCase() ) {
message = getStudentReport( student );
print(message);
}
}
Example of code that doesn't work which I am referring to:
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if ( search.toLowerCase() === student.name.toLowerCase() ) {
message = getStudentReport( student );
print(message);
} else {
print(noStudent);
}
}
Mindaugas Kunevičius
12,029 PointsMindaugas Kunevičius
12,029 PointsHow? I have the same problem, I believe it should work both ways, but it doesn't. My code is exactly the same...
Keith Corona
9,553 PointsKeith Corona
9,553 PointsHello Mindaugas,
If you are using the Chrome browser, you might need to clear the cache. I don't know what happened for me, but when I tried my code both ways the day after posting this question, it worked as it should've.