Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial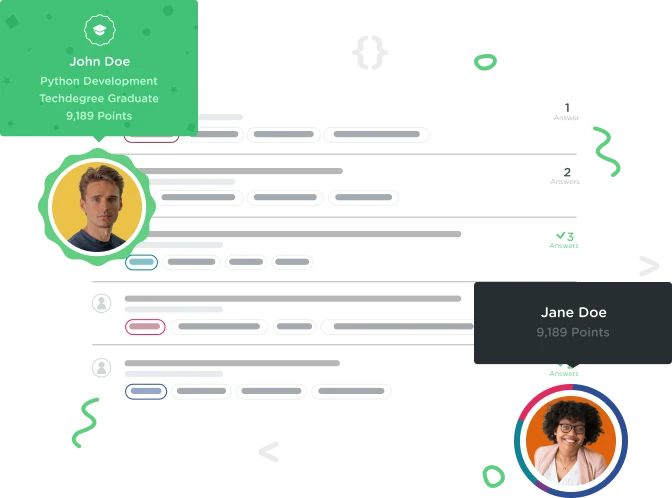

Oğulcan Girginc
24,848 PointsWhy do we need self in the code provided?
Can someone explain me the reason of using self
in the make_mates
instance method? (Code is taken from The Well-Grounded Rubyist book, p143)
class Car
@@makes = []
@@cars = {}
attr_reader :make
def self.total_count
@total_count ||= 0
end
def self.total_count=(n)
@total_count = n
end
def self.add_make(make)
unless @@makes.include?(make)
@@makes << make
@@cars[make] = 0
end
end
def initialize(make)
if @@makes.include?(make)
puts "Creating a new #{make}!"
@make = make
@@cars[make] += 1
self.class.total_count += 1
else
raise "No such make: #{make}."
end
end
def make_mates
@@cars[self.make] #Why do we need self? (Works without it!)
end
end
class Hybrid < Car
end
Car.add_make("Honda")
Car.add_make("Ford")
h = Car.new("Honda")
f = Car.new("Ford")
h2 = Car.new("Honda")
puts "Counting cars of same make as h2..."
puts "There are #{h2.make_mates}."
puts
puts "Counting total cars..."
puts "There are #{Car.total_count}."
puts
h3 = Hybrid.new("Honda")
f2 = Hybrid.new("Ford")
puts "There are #{Hybrid.total_count} hybrids on the road!"
Output:
Creating a new Honda!
Creating a new Ford!
Creating a new Honda!
Counting cars of same make as h2...
There are 2.
Counting total cars...
There are 3.
6 Answers

Oğulcan Girginc
24,848 PointsAs it turns out, self
is not needed in this specific case.
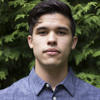
David Cisneros
10,472 Pointshttps://www.jimmycuadra.com/posts/self-in-ruby/ this guy gives a very good explanation for it.

Oğulcan Girginc
24,848 PointsAgreed. Probably read that one tens of times!
However, it didn't help in this case because both @@cars[self.make]
and @@cars[make]
(both class variables are clickable) points to the Car object "Honda". So, it raises the question of why self is needed in this specific case, if both of them points to the same object?
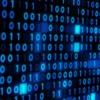
Alexander Davison
65,469 PointsMethods with "self" means that ONLY that class that method is in an access the method.

Oğulcan Girginc
24,848 PointsI think, you tried to define a class method...
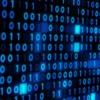
Alexander Davison
65,469 PointsOh sorry, I was wrong XD
Those "self" methods in the class allow you to call the method WITHOUT making an instance.
Example:
class Hello
def initialize
@something = "Hello!"
end
def self.greetings
return @something
end
end
# You can run the "self.greetings" method like this:
Hello.greetings
# If the method was called "greetings" and not "self.greetings", you would have to do this:
hello = Hello.new
hello.greetings
Hope that helps!

Oğulcan Girginc
24,848 PointsThanks for trying xela888 but, I think, I know how class methods work. My question is more specific, compared to your answer because both @@cars[self.make]
and @@cars[make]
(both class variables are clickable) points to the Car object "Honda". So, what I am having hard time to understand is; why self is needed in this specific case, if both of them points to the same object?
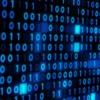
Alexander Davison
65,469 PointsI'll say it is just time-saving in that case, although I never use it.
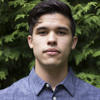
David Cisneros
10,472 PointsHey Oğulcan Girginc,
Thats a good question. I misread and thought you were just talking about that topic in general. I'll take a gander at it myself because I'm not too familiar with this either. Hows the book? Do you find it a useful resource for ruby knowledge?

Oğulcan Girginc
24,848 PointsI would highly recommended it, but it's not definitely not for beginners. If you think you have covered basics, it would be a great next step!