Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial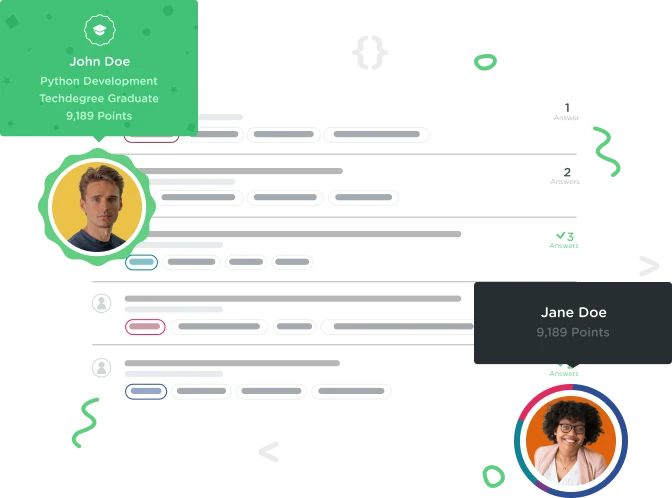

sebastien penot
3,292 PointsWhy do you need to declare the empty string "name" at the beginning of the function?
In the video tutorial, Jason declares an empty String name at the beginning of the function. Why is this required? I just returned the variable answer before breaking the loop and the method worked.
def get_name
puts " Please enter your name (it must contain at least 5 characters): "
loop do
answer = gets.chomp
if answer.length >= 5
puts "Hi #{answer}, nice to meet you"
return answer
break
else
puts "Your name requires at least 5 characters, please try again"
end
end
end
2 Answers
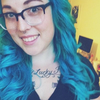
Meagan Waller
19,382 PointsHi Sebastien,
The reason that name
is set to an empty string before the loop is due to scoping.
If you were to run this code without declaring name outside of the loop you'd get this error:
NameError: undefined local variable or method 'name' for main:Object
The reason for that is because variables declared inside of a loop are available to that loop only. So, if name was only declared inside the loop as name = gets.chomp
, that name
variable and assignment is lost once you exit the loop, and the return name
statement at the end of the function would not know what name means.
By declaring name as an empty string outside of the loop, the variable name
is now available to the entire function. The loop inside of the function can modify it; and it does by reassigning the value from an empty string to gets.chomp
.
Try running this code without declaring the name = ""
before the loop and you'll see what I mean. Make sense?
Also, this blog post might be helpful to you!
William Li
Courses Plus Student 26,868 PointsI just took a quick look at your code, it seems that you're right; there's no need to declare name
as empty string here.
William Li
Courses Plus Student 26,868 PointsWilliam Li
Courses Plus Student 26,868 PointsMeagan, yeah, in Jason's version of the code during lecture,
name
has to be set to an empty string; but in the code posted here, Sebastien actually made a change by moving the return statement inside the loop, this new version can in fact works without first settingname
as empty string outside of the loop. So yeah, both versions are correct.Unsubscribed User
11,042 PointsUnsubscribed User
11,042 PointsThanks for the explanation! ...but I couldn' t get why it doesn't work in this way too - I've placed the name variable outside the method declaration, so I think that it would be visible even inside the method definition, but It throws this error: undefined local variable or method `name' for main:Object (NameError)