Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial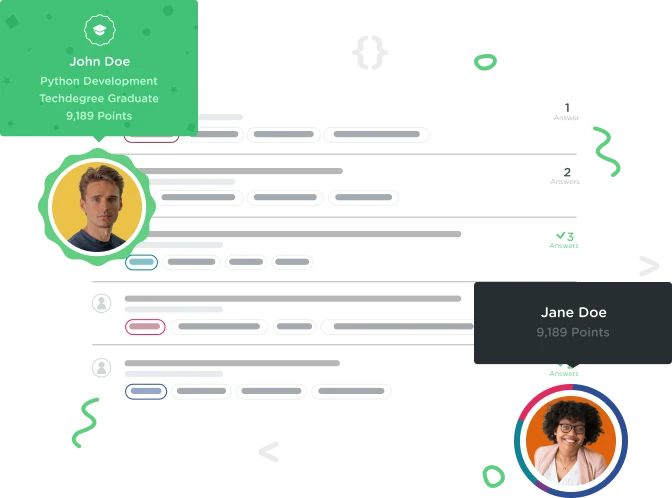

Gary Gibson
5,011 PointsWhy does adding arguments ruin my method? (Ruby)
When I write my code without arguments like this...
def add
puts "Enter two numbers and I'll add them. "
a = gets.to_i
b = gets.to_i
puts "Here is the sum of #{a} and #{b}."
puts a + b
end
add
It works.
But when I add arguments...
def add(a,b)
puts "Enter two numbers and I'll add them. "
a = gets.to_i
b = gets.to_i
puts "Here is the sum of #{a} and #{b}."
puts a + b
end
add(a,b)
I get
9:in <main>': undefined local variable or method
a' for main:Object (NameError)
I'm clearly not understanding something about arguments.
2 Answers

Jaro Schwab
8,957 PointsYou need to declare the variables you want to pass in the method first. On Line 9, you're calling your method and you're passing in the variables a and b. The problem here is that you haven't declared those two variables. Ruby now looks for the variable a and b, does not found them and throw an error. Hope this helps..
Happy Coding :)

Jaro Schwab
8,957 PointsSo, yeah, in this case you don't really need the arguments. But imagine, this function would be a lot more complex. One time, you want to call it with values you entered in, and the other time you just need to execute the same function with hardcoded values. You see, this would not work anymore. So there would be two options now. 1st, just copy and paste the function and add some arguments, delete the "a = gets.to_i" statements. But this is not good and not DRY. The point of a method is that you can reuse it over your application. So, 2nd option, rewrite the method so you can reuse it!
Gary Gibson
5,011 PointsGary Gibson
5,011 PointsOkay, I think I get it. It works when I do this:
def add(a,b) .... add(2,3)
When I pass in values at the bottom, the code runs. I was confused by this and thought that would prevent me from getting the sum of the variables I inputted. It didn't.
The code works fine without me adding any arguments. So I'd rather write it as:
def add ... add
Is there something wrong with doing it this way?
Jaro Schwab
8,957 PointsJaro Schwab
8,957 PointsI do not fully understand what you mean by this:
When I pass in values at the bottom, the code runs. I was confused by this and thought that would prevent me from getting the sum of the variables I inputted. It didn't.
I'm assuming that you're confused that you didnt get the output of 5 (2 + 3). Thats happens because of following reason:
in you method, you're redeclareing your parameters a and b with the input the user entered.. So, if you delete the two lines
You should get the output of 5 - by calling "add(2,3)".
So, before you're calling your method, you could do something like this:
And now, you will get the result of the numbers you entered, but you passed them in as arguments..
Could I help you with this?
Gary Gibson
5,011 PointsGary Gibson
5,011 PointsI am saying that this works:
And this works:
The second version where I have (a, b) in the definition and (2, 3) passed in the bottom allows me to enter my own numbers and have them calculated when I run the program. The (2, 3) that are passed in don't get added; the numbers I input when prompted get added. Which is what I want.
But it makes more sense to me the way it's written at top without arguments.