Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial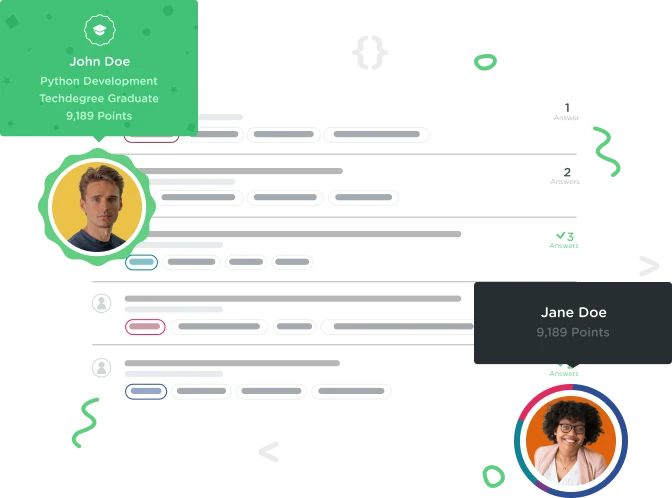

Jackson Monk
4,527 PointsWhy does innerHTML attribute skip its first assigned value?
I am writing a program in which I will have a header that communicates with you by fading in, showing its text of course, fading out, changing its text, then fading in again to let you see the new text. First let me show you the code. Disregard the millis variable, and this is also assuming the h1 variable holds the header:
function changeText(newText) {
body.removeChild(h1);
h1 = undefined;
h1 = document.createElement('h1');
h1.innerHTML = newText;
h1.style.fontSize = '60px';
body.appendChild(h1);
h1.style.display = 'none';
}
h1.innerHTML = "First text";
$(h1).hide().delay(millis = millis + 2000).fadeIn(400, () => {$(h1).delay(3500).fadeOut(400);});
changeText("Second text");
$(h1).delay(millis = millis + 1500).fadeIn(400, () => {$(h1).delay(3500).fadeOut(400);});
I thought this would work, but when the header fades in for the first time, it has the value of "Second Text". Why does it not have its intended "First text" value? Is it something I am doing or that can be worked around? Or is it just a limitation of the innerHTML attribute?
1 Answer

Nathan Thomas
13,477 PointsHi there,
Cool piece of functionality, I couldn’t get it to run as is though, kinda curious about that but here’s what I found nevertheless.
I believe you need to grab the h1 element and assign it to the variable h1 before calling a method on it. Something like:
var h1 = document.querySelector("h1");
I believe you’re using this h1 variable inside the changeText function, but you don’t include it as an argument in the method call or define it as a parameter in the method definition. Maybe add something like:
changeText("Second text", h1);
function changeText(newText, h1) {}
I believe you’ll need to grab the body element before calling the remove method on it. Something like:
var body = document.querySelector('body');
I don’t believe you need to set the h1 variable to undefined. This can probably be removed: ‘h1 = undefined;’ I believe by using the var keyword, redeclaring a variable inside the changeText function will also redeclare the variable outside the function. Which I believe is what you want to do.
With these changes the h1 variables seemed to be the correct values at their respective places in the program.
Hope that helps