Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial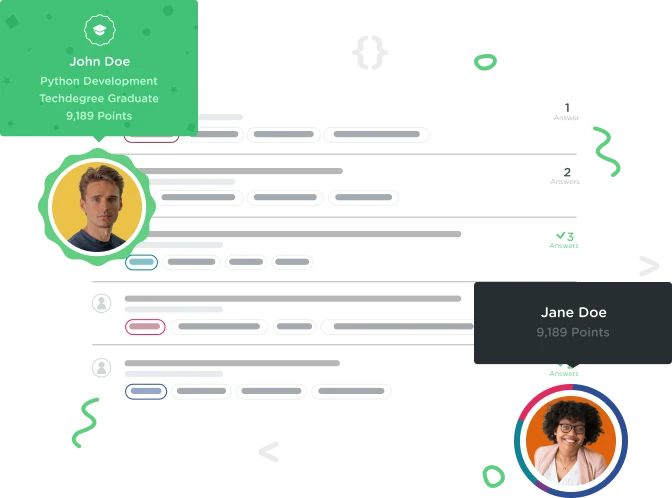

Peter Dommermuth
2,509 Pointswhy does my code work when "taskListItem" and "checkboxEventHandler" were never explicitly defined?
would it still work if I typed literally anything else? why even type anything? how does the browser know what to do when not given that information?
3 Answers

Damien Watson
27,419 PointsHi Peter,
The two elements you are referring to are 'arguments' that get passed into a function. They are not defined because they are passed into the function from the caller.
If you have nothing here then you can still access the arguments passed in by using the 'arguments' object, but defining your arguments is cleaner and the preferred way.
To answer your other question, you can call arguments what ever you like, but it makes sense for them to represent what they actually contain, so:
var bindTaskEvents = function(taskListItem, checkboxEventHandler) {}
// could be :
var bindTaskEvents2 = function(doSomethingWithThisList, whenYouClickCheckboxCallMe) {}
// if you really wanted to.

Peter Dommermuth
2,509 Pointsok, I think I get it. If the argument can be anything though, what's the point of even passing one in at all? would it work if it was just "bindTaskEvent = function() {}?"

Damien Watson
27,419 PointsThat would work, but the point of passing in an argument is that the function does something with this argument and in most cases returns a result. A good example of this is with the random number example they use:
var randomNumber = function(maxNum) {
return Math.ceil(Math.random() * maxNum) + 1;
}
// then call it using the maximum number in your random...
alert(randomNumber(10); // this will return a number between 1 and 10 inclusively
// You could do this with no arguments, but its a little ugly...
var otherRandom = function() {
var maxNum = arguments[0]; // arguments is a JS array, so '0' gets the first argument passed in
return Math.ceil(Math.random() * maxNum) + 1;
}

Damien Watson
27,419 PointsWhen creating your functions, it is best case to add the arguments you expect to use in the function. You may know what argument[0] / argument[1] means, but for someone else picking up your code or trying to use your function, they are going to have to do more work to find what these mean.
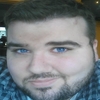
Marcus Parsons
15,719 PointsThe rule of thumb when defining functions is that if you know exactly what is going to go into the function, use defined arguments. If you don't know what is going to go into the function, you can use the arguments
array to get values from the function.
One instance where I used arguments
is in my Extramath.js library. I created a "mean" function that calculates the mean (average) of a series of numbers. It can take any amount of numbers/strings or any amount of numbers/strings inside of an array because it utilizes the arguments
array:
Math.mean = function () {
var total = 0;
var len;
try {
//if the first argument is a string or number
if (typeof arguments[0] === "string" || typeof arguments[0] === "number") {
len = arguments.length;
for (var i in arguments) {
total += parseFloat(arguments[i]);
}
return round(total / len, defPrec);
}
else {
//Otherwise, treat as an array
len = arguments[0].length;
for (var i in arguments[0]) {
total += parseFloat(arguments[0][i]);
}
return round(total / len, defPrec);
}
}
catch (err) {
showError(errorMsg + err);
}
}

Peter Dommermuth
2,509 Pointsawesome, thanks for the detailed responses!