Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial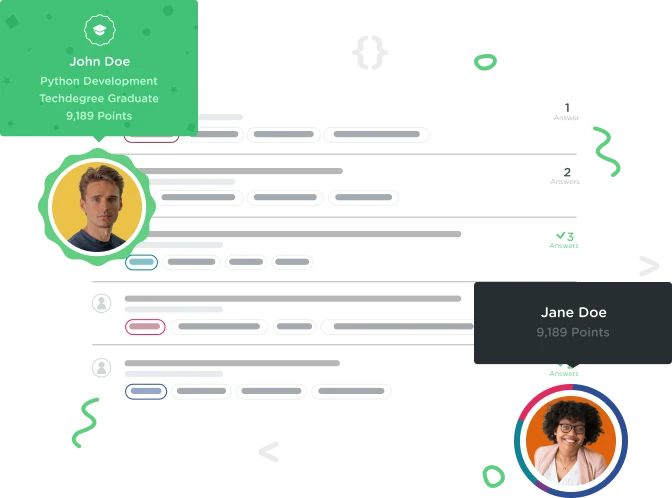

Daniel Kol Adam
3,285 PointsWhy does prop do anything if it was not assigned to anything?
It was just created in the loop, so there was nothing in it yet.. why does it show the country.. or even all variable values?
I'm a little confused at this point.
Thanks.
2 Answers
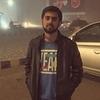
zainsra7
3,237 PointsThat's the beauty of for-in loop,
Let me write it down first so that it becomes easier to explain:
for (var prop in person) {
console.log(prop, ' : ', person[prop]);
}
person is an object containing 'key' and 'value' pairs
Prop is declared and initialized inside the loop with each key from person object, all of this happens behind the curtains. Let's look at this in a sequential way:
1. First Iteration :
prop = person[0] //prop = name;
2. Second Iteration:
prop = person[1] //prop = country;
3. Third Iteration
prop = person[2] //prop = age;
As you can see above , prop always get the 'key' part of object and then to reference to the value part we simply use person[prop] as in console.log().
Let's try to see it in traditional for loop way (it doesn't follow any syntax but just to get the idea of what's happening behind the scenes:
for ( var i = 0; i < (total keys in person); i ++){
var prop = person.key[ i ]; //key#1, Key#2 and so on
console.log( prop , ":" , person.value[prop]) //Value#1 , Value#2 and so on
}
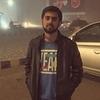
zainsra7
3,237 PointsBrad McKinney exactly prop and key are just mere names, and you can use anything to replace them e.g
for (var hey in person) {
console.log (hey, ":", person[hey]);
}
will produce the same result as with the above code.
What you need to remember is that an object store stuff in a "key" , "value" pair, like
var person = {
name : zain,
age : 22
};
and when traversing using for ... in loop , our counter variable(hey or prop) gets initialized with "key" (name/age) and to access the value stored in key (zain and 22) we use person[hey].
I hope it clears the concept :)
Brad McKinney
14,677 PointsBrad McKinney
14,677 PointsZain Sra im trying to understand this as well. So for clarification's sake, basically the loop accesses the correct data based on SYNTAX, not based on what you choose to name the variables? (If i recall, the instructor was saying that you can name them other things besides "key" or "prop") So basically, where the "prop" variable is in the iteration tells the program to access the keys and the square brackets tell the program to access the values. Is this correct?