Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial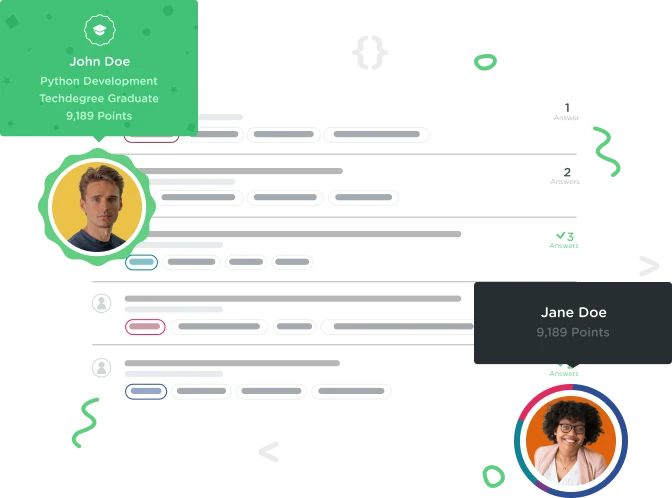
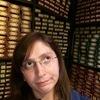
Linda Gomes
3,584 PointsWhy does the else overrides the if?
When I add the else to show an alert if the student does not exist, it just show the alert every time, even if the student does exist.
var message = '';
var student;
var search;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getReport(student) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
while (true) {
search = prompt("Type student name to load file. To exit type 'quit'.");
if (search === null || search.toLowerCase() === "quit") {
break;
} for (var i = 0; i < students.length; i += 1) {
student = students[i];
if (student.name.toLowerCase() === search.toLowerCase()) {
message = getReport(student);
print(message);
} else {
message = "<h3> No student named " + search + " was found.</h3>";
print(message);
}
}
}
2 Answers

andren
28,558 PointsThat happens because the code within the for
loop runs once for every student in the students
array. And the if
statement will only be true the one time it pulls out the student you searched for. All of the other times it will not be true and the else
will run.
So unless the student you search for is the last one the for
loop runs though you will end up with the else
statement being the last one to run.
You can fix this issue by adding the break
keyword to the if
statement like this:
var message = '';
var student;
var search;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getReport(student) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
while (true) {
search = prompt("Type student name to load file. To exit type 'quit'.");
if (search === null || search.toLowerCase() === "quit") {
break;
} for (var i = 0; i < students.length; i += 1) {
student = students[i];
if (student.name.toLowerCase() === search.toLowerCase()) {
message = getReport(student);
print(message);
break; // Added break
} else {
message = "<h3> No student named " + search + " was found.</h3>";
print(message);
}
}
}
That way the loop ends once it runs the if
statement, which means that you don't end up with the else
statement running after it.

Adrien Contee
4,875 PointsUnless I'm missing something, this code doesn't work as intended to complete the bonus:
var message = '';
var student;
var search;
var students = [
{
name: "Tom",
track: "iOS Development",
achievements: 43,
points: 73839
},
{
name: "Jerry",
track: "Web Design",
achievements: 23,
points: 89498
},
{
name: "Sam",
track: "Front End Development",
achievements: 86,
points: 23187
},
{
name: "Kim",
track: "Back End Development",
achievements: 24,
points: 90594
},
{
name: "Jane",
track: "Java Development",
achievements: 73,
points: 87328
}
];
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getReport(student) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
while (true) {
search = prompt("Type student name to load file. To exit type 'quit'.");
if (search === null || search.toLowerCase() === "quit") {
break;
} for (var i = 0; i < students.length; i += 1) {
student = students[i];
if (student.name.toLowerCase() === search.toLowerCase()) {
message = getReport(student);
print(message);
break; // Added break
} else {
message = "<h3> No student named " + search + " was found.</h3>";
print(message);
}
}
}
// The 'for loop' will always print out the else statement in cases where the name you type in the
// prompt is not the first item in the students array at index 0.
// If you type in 'Jerry' as it is in my students array, the loop will ask 'does student.name [which is position 0]
// at this point equal to 'Jerry'? No it doesn't , its 'Tom'... run the else block
// Even though 'Jerry' is the name of someone in the array, the code never has a chance to get there.
I haven't found a solution. I tried for like an hour before giving up. Not going to stackoverflow a treehouse project.
If anyone has a solution I would love to see it. Especially if it only involves the limited knowledge we've gained so far.
Linda Gomes
3,584 PointsLinda Gomes
3,584 PointsThank you... but now I have another problem. I had found a solution for the "two people with the same name" problem and now it doesn't work.
I had added a + to the message, like this:
message += getReport(student);
but now it doesn't work.