Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial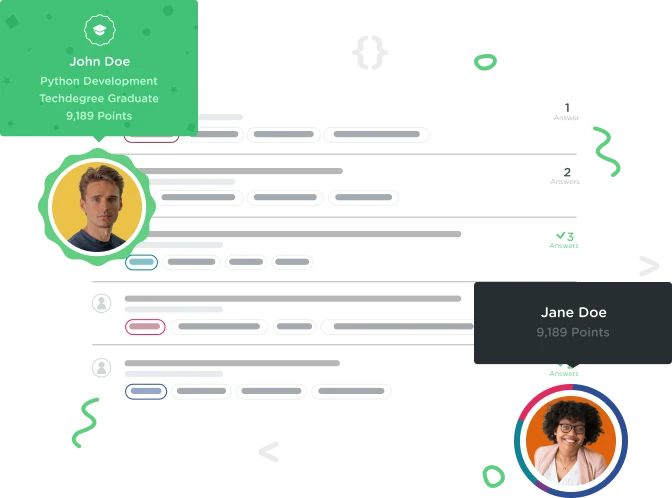

Emily Coltman
Courses Plus Student 9,623 PointsWhy does this say that the method does not return a boolean value when I have put "return true"?
Hi there - not sure why this doesn't work and grateful for help? Thank you!
class TodoList
attr_reader :name, :todo_items
def initialize(name)
@name = name
@todo_items = []
end
def add_item(name)
todo_items.push(TodoItem.new(name))
end
def contains?(name)
if todo_items.include?(name)
return true
end
end
def find_index(name)
index = 0
found = false
todo_items.each do |item|
found = true if item.name == name
break if found
index += 1
end
if found
return index
else
return nil
end
end
end

Myers Carpenter
6,421 PointsYou are only returning true if it's true
, but you'll return nil
if it's false
. Do you even need an if
? What does todo_items.include?(name)
return?
2 Answers
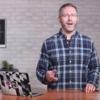
Jay McGavren
Treehouse TeacherHi, Emily Coltman! There's two issues here.
First: your contains?
method is set up to return true
when it finds a match. But it also needs to be set up to return false
when it doesn't find a match. The hints provided by the challenge didn't make this totally clear, so I've updated the challenge to communicate that more effectively.
Second: your contains?
method won't find any matches as it is right now. I would suggest calling the find_index
method within contains?
. If find_index
returns nil
, then contains?
should return false
. But if find_index
returns a number, then contains?
should return true
.

Emily Coltman
Courses Plus Student 9,623 PointsThanks Jay - and thanks Myles!
I've tried putting both your suggestions into practice but I'm still stuck...
My code now reads:
def contains?(name)
if todo_items.find_index(name) != nil
return true
else
return false
end
end
This still gives me an error message though! What am I doing wrong?! Please help :)
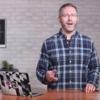
Jay McGavren
Treehouse TeacherEmily Coltman you're getting close! But todo_items.find_index
attempts to call a method named find_index
on the array in todo_items
, which doesn't have a method named find_index
defined. Instead, you want to call the find_index
method on the current TodoList
object.
You can call another method on the current object (self
) either with self.other_method
or just other_method
(the self.
is added implicitly):
class MyClass
def method_1
puts "In method_1"
# Call another method on this object
self.method_2
# Same as above
method_2
end
def method_2
puts "In method_2"
end
end
object = MyClass.new
object.method_1
This outputs:
In method_1
In method_2
In method_2
So if you take todo_items.
off of your call to find_index
, your code should pass the challenge.

Emily Coltman
Courses Plus Student 9,623 PointsIt worked!! Yay!!
Thanks so much Jay, most grateful for your help!
Emily

Ken jones
5,394 PointsAs a point of interest you can refactor that entire find_index
method into one line by using Array#index
.
like so ->
class TodoList
attr_reader :name, :todo_items
def initialize(name)
@name = name
@todo_items = []
end
def add_item(name)
todo_items.push(TodoItem.new(name))
end
def contains?(name)
find_index(name) != nil
end
def find_index(name)
todo_items.index { |item| item.name == name }
end
end
This will do exactly the same thing and pass the challenge :-)
Emily Coltman
Courses Plus Student 9,623 PointsEmily Coltman
Courses Plus Student 9,623 PointsPlease can I have some help with this?! Thank you :)