Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial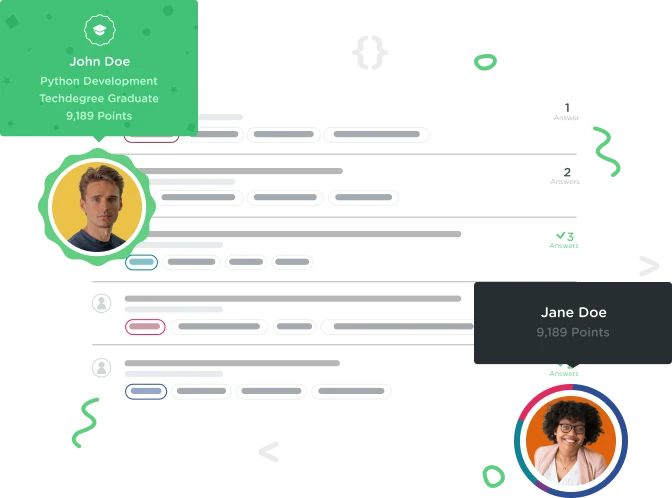

emhes
6,934 PointsWhy doesn't ( guess !== randomNumber) work?
This is what I wrote as the solution before watching Dave's solution.
I don't understand why this wouldn't work:
var randomNumber = getRandomNumber(10); var guess; var guessCount = 0;
function getRandomNumber( upper ) { var num = Math.floor(Math.random() * upper) + 1; return num; }
do { guess = prompt("I am thinking of a number between 1 and 10. What is it?"); guessCount += 1; } } while ( guess !== randomNumber)
document.write("It took you " + guessCount + " tries to guess the number " + randomNumber);
Isn't that saying: DO
- prompt the user for their answer and store their answer in the 'guess' variable
- increase the guess count by 1 WHILE
- if the user's answer is not equal to the Random Number then continue to run the DO loop
What am I missing?
2 Answers
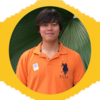
Erick Kusnadi
21,615 Points1) there's an extra } 2) there's an infinite loop. randomNumber is an Integer, while guess is a String. When you use the prompt function the input from the user will be a string, so you have to parse guess into a number, you can use the parseInt() function for this. http://www.w3schools.com/jsref/jsref_parseint.asp
Or you can even just change: ( guess !== randomNumber) to ( guess != randomNumber). The second version automatically tries to change the string to a number, while the first version doesnt
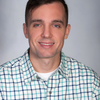
Jared Hensley
8,106 PointsI used the following solution to solve, slightly different comparison in the while. Seems to be simpler to me but I guess there are multiple ways to do the same thing!
var randomNumber = getRandomNumber(10);
var guess;
var guessCount = 0;
var correctGuess = false;
function getRandomNumber( upper ) {
var num = Math.floor(Math.random() * upper) + 1;
return num;
}
do {
guess = prompt("Guess a number between 1 and 10?");
guessCount++;
if (parseInt(guess) === randomNumber) {
correctGuess = true;
}
} while (correctGuess == false)
document.write("<h1>You guessed the number!</h1>");
document.write("It took you " + guessCount + " attempt(s) to guess the number " + randomNumber);
emhes
6,934 Pointsemhes
6,934 Pointsah... that's right. Prompt inputs are considered strings even if a number is entered... That's what I was forgetting. Thank you!