Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial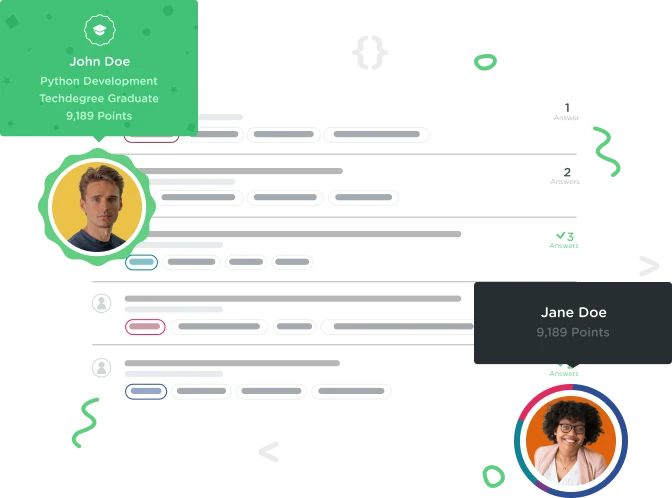

Terrell Stewart
Courses Plus Student 4,228 PointsWhy doesn't my program break once I have guess the secret word?
The program continues until I run out of tries even when I correctly guess my secret word
import random
#make a list of words
words = [
'apple',
'orange',
'banana',
'coconut',
'strawberry',
'lime',
'grapefruit',
'lemon',
'kumquat',
'blueberry',
'melon'
]
while True:
start = input("Press enter/return to start, or enter Q to quit: ")
if start.lower() == 'q':
break
#pick a random word
secret_word = random.choice(words)
bad_guesses = []
good_guesses = []
while len(bad_guesses) <7 and len(good_guesses) != len(list(secret_word)):
#draw guessed letters and strikes
for letter in secret_word:
if letter in good_guesses:
print(letter,end='')
else:
print('_',end='')
print('')
print('Strikes: {}/7'.format(len(bad_guesses)))
print('')
#take guess
guess = input("Guess a letter: ").lower()
if len(guess) !=1:
print("You can only guess a single letter!")
continue
elif guess in bad_guesses or guess in good_guesses:
print("You've already guessed that number")
continue
elif not guess.isalpha():
print("You can only guess a letter")
continue
if guess in secret_word:
good_guesses.append(guess)
if len(good_guesses) == len(list(secret_word)):
print("You win! The word was {}".format(secret_word))
break
else:
bad_guesses.append(guess)
else:
print("You didnt guess it! My secret word was {} ".format(secret_word))
#print out win/loose
3 Answers
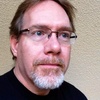
Chris Freeman
Treehouse Moderator 68,426 PointsIf the secret _word has a repeated letter the length of good_guesses can never equal the length of secret_word. In the comparisons change list
to set
:
while len(bad_guesses) <7 and len(good_guesses) != len(set(secret_word)):
# ...
if len(good_guesses) == len(set(secret_word)):
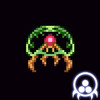
Adam Young
15,791 PointsThe script only counts one addition to the good_guesses list, even if there are duplicate letters.
For example, if the word is strawberry, when a user guesses "r", good_guesses only increments 1, when it should increment 3.
This means that on words with duplicate letters, the condition to trigger your break will never be met.
Using strawberry again as an example, len(good_guesses) would equal 8, while len(list(secret_word)) is 10.
There are a lot of ways to do this, but here's what worked for me:
#take guess
guess = input("Guess a letter: ").lower()
if len(guess) !=1:
print("You can only guess a single letter!")
continue
elif guess in bad_guesses or guess in good_guesses:
print("You've already guessed that number")
continue
elif not guess.isalpha():
print("You can only guess a letter")
continue
for letter in secret_word:
if guess == letter:
good_guesses.append(guess)
if guess not in secret_word:
bad_guesses.append(guess)
if len(good_guesses) == len(list(secret_word)):
print("You win! The word was {}".format(secret_word))
break
else:
print("You didnt guess it! My secret word was {} ".format(secret_word))

Jason Roseberry
1,080 PointsSomeone should really put this in teacher notes or on the video. I just spent an hour trying to figure out what I was doing wrong. ):
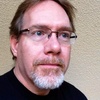
Chris Freeman
Treehouse Moderator 68,426 PointsDebugging code is great experience. Hopefully it wasn't too frustrating. Some of the best learning comes from answer the "What's wrong with my code" type questions.
There is an acknowledgement of the issue in the Teacher's Notes for the associated video that mentions:
Did you notice?
There's a tricky condition (on purpose) in the final version of our code from this video. Words that have repeated characters won't be marked as correct once they're all correctly guessed due to our len()
comparisons. See if you can find a way to fix that yourself!
We'll write a version without that bug in the next video. link
Adam Young
15,791 PointsAdam Young
15,791 PointsI knew someone would find a more concise way to do this! Way more readable than mine.
Terrell Stewart
Courses Plus Student 4,228 PointsTerrell Stewart
Courses Plus Student 4,228 PointsThanks I learned alot