Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial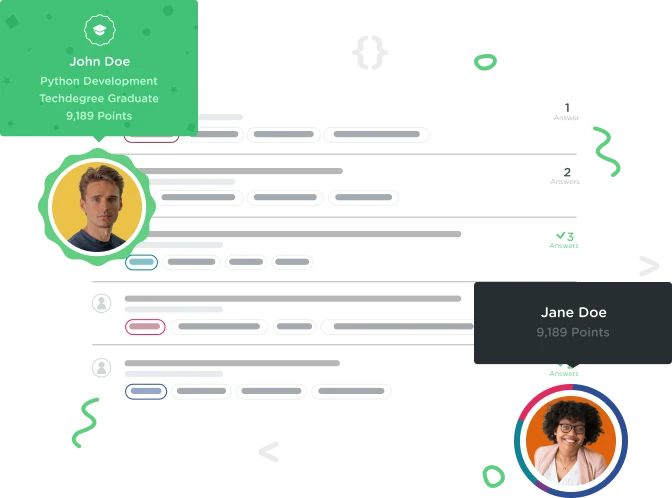
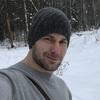
gennady
14,145 PointsWhy I can see the result of document.write only after all the prompts and alert messages disappear?
Hi! As I understand, the code in the js file executing string by string. If that is true why I can see the result of the first string only after the alert disappear, which is obviously should be executed after...
document.write('This string I would like to see first');
alert("But I wouldn't while this message dissapear");
5 Answers
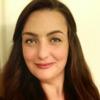
Jennifer Nordell
Treehouse TeacherHi there! Not necessarily. JavaScript is a little special in that it's an interpreted language and implements something called "function hoisting" by some. Essentially, all of your code is compiled and goes into the memory at the same time. Now, I could be wrong here, but I believe it interprets the alert method to be of higher priority because it is acting on the Window
object whereas the document.write is a method acting on the Window.document object.
Here is some relevant documentation:
Hope this helps!

andren
28,558 PointsI'm sorry but Jennifer Nordell's answer is actually wrong, "function hoisting" means that function and variable declarations (but not calls) are essentially moved to the top of the file at runtime, meaning that you can call a function before you actually define it, which would normally not be possible since JavaScript otherwise reads your code from top to bottom. But it has no bearing on what function gets run first, JavaScript runs though your commands from top to bottom, it does not run on any kind of priority system when it comes to what commands it executes first.
The reason why you see the alert pop-up before you see something written to the page is that modern browsers are set up such that they once they encounter a JavaScript file they will essentially pause the rendering of HTML and run though the entire JavaScript file before they resume the HTML rendering, this means that while the document.write does run first, you don't get to see the result of it before the rest of the file has finished executing.
This is part of the reason why it is no longer considered a good practice to place JavaScript links in the head element, but to instead place them at the bottom of the body element. Since placing it inside the head element will result in the page being completely blank until the JavaScript file has been run though.
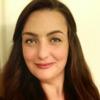
Jennifer Nordell
Treehouse TeacherThis quote of yours is interesting and actually helps to make my point:
"..modern browsers are set up such that they once they encounter a JavaScript file they will essentially pause the rendering of HTML and run though the entire JavaScript file before they resume the HTML rendering.. "
I believe it to be a restatement of what I said. That JavaScript acting on the Window will take precedence over the JavaScript acting on the rendering of the HTML or document object. So the JavaScipt acting on the document will be paused while the JavaScript acting on the Window is still fair game.
You might be correct about the function hoisting, but I'd point out that the Mozilla Developer Network disagrees with you as evidenced by this documentation
Here's the top paragraph from said documentation:
Hoisting is a term you will not find in the JavaScript docs. Hoisting was thought up as a general way of thinking about how execution context (specifically the creation and execution phases) work in JavaScript. But, hoisting can lead to misunderstandings. For example, hoisting teaches that variable and function declarations are physically moved to the top your coding, but this is not what happens at all. What does happen is the variable and function declarations are put into memory during the compile phase, but stays exactly where you typed it in your coding.
Also, note that when I run this code:
<!DOCTYPE html>
<html>
<head>
</head>
<body>
<script src="js/script.js"></script>
</body>
</html>
combined with this...
document.write('This string I would like to see first')
alert("But I wouldn't while this message dissapear");
... it results in the alert being displayed before the string is written to the document.

andren
28,558 PointsI believe it to be a restatement of what I said. That JavaScript acting on the Window will take precedence over the JavaScript acting on the rendering of the HTML or document object. So the JavaScipt acting on the document will be paused while the JavaScript acting on the Window is still fair game.
My main point was that it had nothing to do with precedence, JavaScript itself does not attribute any precedence to any command, it just executes it line by line (once it's done with hoisting). The browser does prioritize JavaScript over HTML, but not any specific part of it, it runs though the entire file before resuming HTML rendering regardless of whether the JavaScript file ends up doing anything to the DOM at all.
And I'd disagree that Mozilla's definition of hoisting defies my own, my definition was a simplification, I said it essentially moves the declarations to the top, as that's an easy simplified way of thinking about it and accurate enough that it helps beginners understand the implications of it. I never claimed it physically moved things around or anything of the sort. Also hoisting was not really the main topic that I wanted to discuss in the my comment in the first place, I only brought it up in response to your post.
As for your last paragraph with the code example, I think you might have misunderstood part of the point I was making, I was not saying that placing the script in the body would change the behavior I was describing as far as the document.write was concerned. As I said once the browser starts to run though a JavaScript file it stops rendering HTML until it is done processing the file, and the alert dialog pauses that processing. My point about placing the script at the end of the body element was that it enables the HTML elements that is actually in the HTML file beforehand to be rendered to the screen right away before the JavaScript file is run and the HTML rending gets paused. Meaning that you get to see the HTML page right away even if tons of Javascript code ends up executing right after it is rendered, if you placed the JS file in the head element you would have to wait for all of that JavaScript code to execute before any of the elements that was already on the page started rendering at all, leading the page to appear blank while the JS file was executing.
Edit: I am certainly not a JavaScript expert, and DOM manipulations is one of my weaker areas, so I'll admit that I might be mistaken on some details myself, but I do know enough about it that I found your explanation miselading, my main gripe was the fact that you made it sound like some commands was executed by the JS interpreter before others based on some priority system, which is just not the case. Even though you don't see the result of document.write the command is still executed first, the browser simply doesn't show the result of it being executed until after it has run though the script.
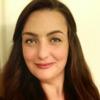
Jennifer Nordell
Treehouse TeacherI think that we actually agree on this. I think our big contention here is on the definition of priority/precedence. Yes, I understand that JavaScript does not give priority to any given command over the other. However, when we have object A that we can manipulate with JS and object B which is paused from being manipulated, this in my mind constitutes a sort of implicit priority. If code acting on the Window object is allowed to run fully, but not the document object (until all the JS is loaded) then I consider that to be an implied priority/precedence. At this point, we're arguing semantics.
My point with the "hoisting" part to the original poster of the question was to let them know that this exists and is a little special to JavaScript. The intended point was that things do not always need to be coded in a specific order to make it function properly. It was intended as a response to this quote:
As I understand, the code in the js file executing string by string.
But in this particular example, hoisting does not come into play.
And yes, I understand the point of loading in the body. My intention for including the code snippet was to make it clear to the original poster that the behavior would not be altered in any way.

andren
28,558 PointsYes to be honest after having had a bit of time to think about it, and having this conversation, I have started to think that we might not actually be in that much of a disagreement. You are right in that we seem to have slightly different opinions on what precedent actually means in this context, and that is the main reason why I wrote my comment, which in hindsight might have come across a bit more aggressively than I intended.
As I edited in to my previous post, my main issue was that I thought you were implying that JavaScript actually executed code based on some precedent system, but since that was mostly down to a misunderstanding I will take back my claim that your answer is "actually wrong". I think I went a bit to far in my original comment.
Since this "argument" was based on a misunderstanding that has now been cleared up I don't actual have much to argue back against, we seem to disagree slightly on the definition of precedence/priority but other than that we do indeed seem to agree with each other. So I guess I'll concede this argument to you. I don't actually think I disagree with your overall message. I have now up voted your response, I'll leave my own response mainly so that people can continue to see our discussion. Hope you have a nice day :) and sorry for being so aggressive in the beginning.

Tornike Bestavashvili
4,275 PointsIf you want that document.write appeared after alert, you must write first alert() and then document.write():
alert("But I wouldn't while this message dissapear"); document.write('This string I would like to see first')
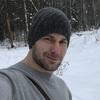
gennady
14,145 PointsThanks! That makes sense. I'll do this homework! =)
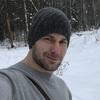
gennady
14,145 PointsThank you Anden and Jennifer for your detailed response. What about console.log()?
console.log('This string I would like to see first');
alert("But I wouldn't while this message dissapear");
It doesn't write to html body, but behaves identically. And what technics could be used to change this priority. How cam I see 'This string I would like to see first' first? Thanks!