Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial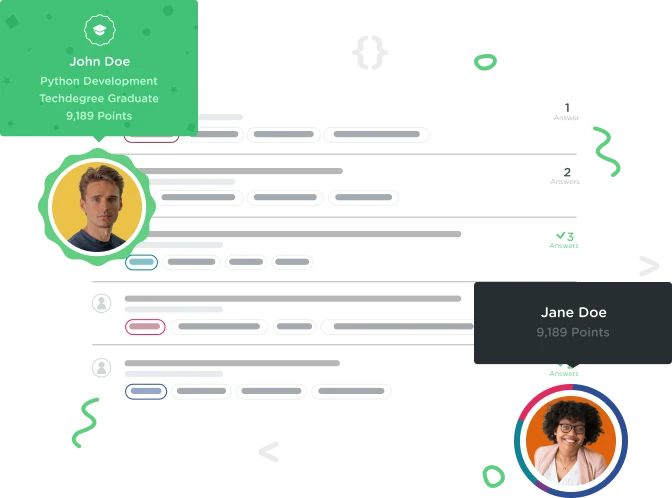

Hussein Amr
2,461 Pointswhy is my code not working
def disemvowel(word):
vowels = ['a','e','i','o','u','A','E','I','O','U']
for letter in vowels:
if letter in word:
word.remove(letter)
else:
continue
return word
def disemvowel(word):
vowels = ['a','e','i','o','u','A','E','I','O','U']
for letter in vowels:
if letter in word:
word.remove(letter)
else:
continue
return word

Hussein Amr
2,461 PointsEric Boxer Didn't work
3 Answers
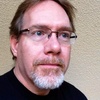
Chris Freeman
Treehouse Moderator 68,460 PointsRemember the word
argument is a string. To break word
up into a list use list(word)
. This can be reassign back to word
:
word = list(word)
Loop over the new extracted letters now in word
:
for letter in word[:]:
The extra [:] means the for
loop should iterate on a copy of word
. A copy keeps the index references safe from deletions of letters from original extract word
.
Now, if letter
is in vowels
it can be remove from word
using word.remove(letter)
When the for
loop completes, rejoin the letters of word
back into a string. Use the join()
method for this:
return "".join(word)
Good Luck!!

Hussein Amr
2,461 Pointsthanks man i got it now :D
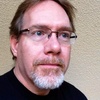
Chris Freeman
Treehouse Moderator 68,460 PointsYour current approach will only find the first occurrence of each vowel in the word. Try reversing the comparison: check if each letter in the word is in the vowels list.
Post back if you need more hints. Good Luck!!

Hussein Amr
2,461 Pointsstill not working :/ i tried your method but maybe i did something wrong, I just flipped "vowels" and "word"
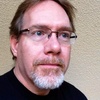
Chris Freeman
Treehouse Moderator 68,460 PointsBe careful not to modify the for
loop iterable. If you're using word
, then modifying word
will throw off the indexes using by the for loop. Instead, use a copy of word
by adding slice copy notation: word[:]
Repost your code if you're still stuck! Keep at it!
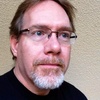
Chris Freeman
Treehouse Moderator 68,460 PointsAlso, remember to convert the word in to a mutable list where letters can be removed. You can't remove letters from a string.

Hussein Amr
2,461 PointsChris Freeman man i tried everything and im still stuck, also i don't know how to convert a word into a mutable
def disemvowel(word):
word[:]
vowels = ['a','e','i','o','u','A','E','I','O','U']
for letter in vowels:
if letter in word:
word.remove(letter)
return word
Eric Boxer
9,026 PointsEric Boxer
9,026 PointsThe argument
word
is a string, and strings have a method ofremove
. Try usingreplace
instead.