Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial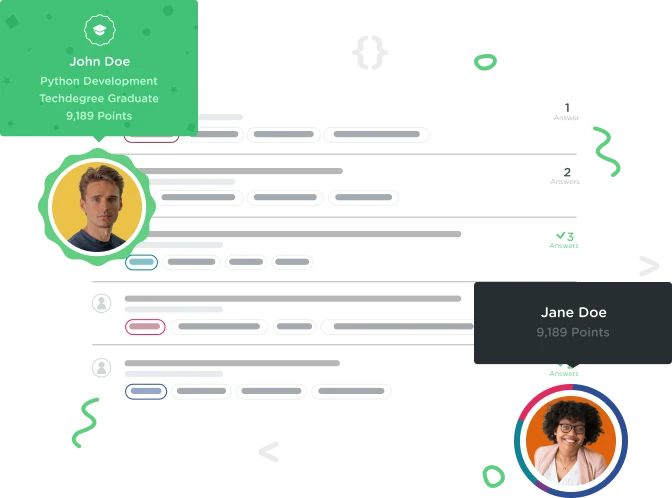

Jordan Gent
10,002 PointsWhy is my developer tools in chrome saying "uncaught Syntax error" (line for my version of the ultimate quiz challenge?
This is how I solved The Ultimate Quiz Challenge:
var correct = 0;
var question1 = prompt("2 + 2=");
if (question1 === "4") {
correct += 1;
}
var question2 = prompt("2-2=");
if (question2 === "0") {
correct += 1;
}
var question3 = prompt("2*2=");
if (question3 === "4") {
correct += 1;
}
var question4 = prompt("2/2=");
if (question4 === "1") {
correct += 1;
}
var question5 = prompt("2*5=");
if (question5 === "10") {
correct += 1;
}
if (correct === 5) {
document.write("<p>Congratulations, you got 5 out of 5 correct! You've earned the golden crown!</p>");
} else if
(correct === 4) {
document.write("<p>Congratulations, you got 4 out of 5 correct! You've earned the silver crown!</p>");}
else if
(correct === 3) {
document.write("<p>Congratulations, you got 3 out of 5 correct! You've earned the silver crown!</p>");}
else if
(correct === 2) {
document.write("<p>I'm sorry, you only got 2 out of 5 correct. You've earned the bronze crown.</p>");}
else if
(correct === 1) {
document.write("<p>I'm sorry, you only got 1 out of 5 correct. You've earned the bronze crown.</p>");}
else
(correct === 0) {
document.write("<p>I'm sorry, you got 0 out of 5 correct. You didn't earn a crown.</p>");
}
My chrome developer tools is telling me that my opening bracket (In the last 3 lines) has an: "Uncaught SyntaxError: Unexpected Token {" . If I remove that final bracket set everything works but it writes out that final document.write. I don't understand why it's saying this.
2 Answers

Jason Anello
Courses Plus Student 94,610 PointsHi Jordan,
The else
block can't have a condition on it.
If you remove the (correct === 0)
then it should work.
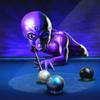
Robert Andrade
7,554 PointsRemember that syntax is the 'grammer' of coding. Too many punctuations, too many conditions as was mentioned earlier, not enough punctuations. Also remember that when the console throws an error, on the far right side on the same line as the error there will be a link to the line of code that ran into trouble. Good Luck