Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial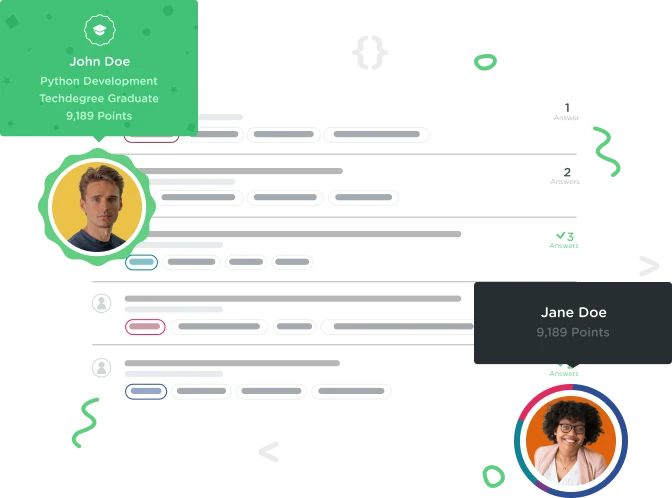

Michael Zarucki
803 PointsWhy is my loop incorrect, and how do I append my results to the var results?
Why is my loop incorrect, and how do I append my results to the var results?
// Enter your code below
var results: [Int] = []
for multiplier in 1...10 {
print("\(multiplier) times 6 is equal to \(Multiplier * 6)")
}
2 Answers
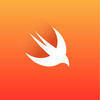
Steven Deutsch
21,046 PointsHey Michael Zarucki,
You can append items to an array by calling the append()
method on the array and passing in the value you want to add. Instead of printing a string for each iteration of a loop, append one of the values of multiplier * 6 to the results array.
// Enter your code below
var results: [Int] = []
for multiplier in 1...10 {
results.append(multiplier * 6)
}
/* This loop will run 10 times,
each time adding a multiple of 6
to the results array. */
Good Luck

andren
28,558 PointsThe loop itself is not incorrect but there is an error in your code. This is one of the situations where looking at the error message the swift compiler produces comes in handy, it is often far more informative than you might expect. You can do so by pressing the preview button when you recive and error. Here is the swift compiler error messge your code produces:
swift_lint.swift:11:44: error: use of unresolved identifier 'Multiplier'
print("\(multiplier) times 6 is equal to \(Multiplier * 6)")
^~~~~~~~~~
swift_lint.swift:9:5: note: did you mean 'multiplier'?
for multiplier in 1...10 {
^
It states that it cannot figure out what "Multiplier" is supposed to refer to, but also point out that you might have meant "multiplier" instead. Which is actually correct.
The error is in other words caused by a typo in the variable name you use in your second string interpolation.
Anyway as for how you append values to the results array that is actually quite simple, arrays has a method called append
that you can pass data into to append them to the array. So for example if I wanted to append the number 5 to the array I would write:
results.append(5)
If you fix the variable typo and add code to append multiplier * 6
to the results array within your loop then your code should pass.