Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial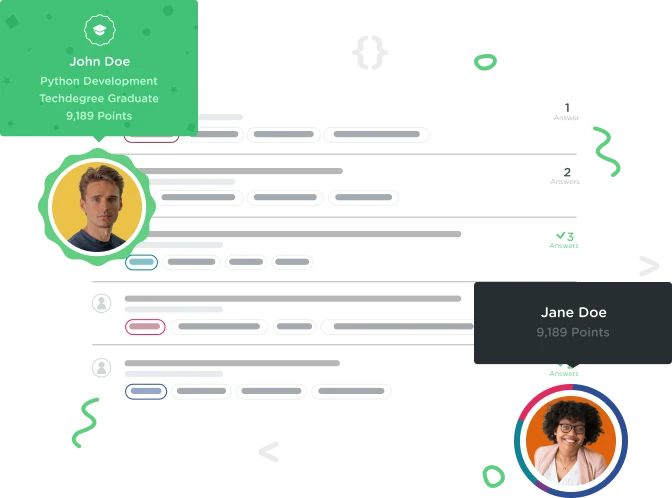
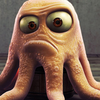
Laurence Foley
16,695 PointsWhy is the following code not passing this treehouse challenge test?
The code works fine when I run it in my own browser but the challenge test doesn't pass it because it doesn't like me using a variable inside the while loop. Why?
var password = "sesame";
while(password !== secret) {
var secret = prompt("What is the secret password?");
if (secret === password) {
document.write("You know the secret password. Welcome");
}
}
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="app.js"></script>
</body>
</html>
1 Answer
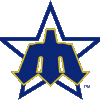
Bryan Tidwell
3,473 Pointsvar password = "sesame";
secret = prompt("What is the secret password?");
while(password !== secret) {
secret = prompt("What is the secret password?");
if (secret === password) {
}
}
document.write("You know the secret password. Welcome");
This is all pretty new to me, but I managed to get it to work by duplicating the variable outside of the loop. I don't think you have to use the "var" to declare inside of a loop?
Additionally, you'll need to move your document.write command outside of the loop for it to display.
Bryan Tidwell
3,473 PointsBryan Tidwell
3,473 PointsBut I'd love a comment from someone more experienced so we can both learn the "why" behind it. Thanks!
Oh, and I probably should have killed the "if" if I was going to move document.write. But it works!! I've got a lot to learn!
Bryan Tidwell
3,473 PointsBryan Tidwell
3,473 PointsThere... cleaned it up a bit. That makes a little more sense to me, too. It declares the variable outside of the loop and kills the unnecessary "If". No reason for it. All we have to do is get the user to knock it out of the loop and we've already defined the string they have to type in.
William Li
Courses Plus Student 26,868 PointsWilliam Li
Courses Plus Student 26,868 Pointshi, Bryan, the if conditional inside the while loop
isn't doing anything here, you can just go ahead and delete it; other than that, the code looks pretty good to me.
Bryan Tidwell
3,473 PointsBryan Tidwell
3,473 PointsThanks, William! I just figured that one out. So the only other question I've got is the variable. Can we not declare a new variable inside of a loop?
Oh, and many thanks to Laurence for letting me hijack his question and use it for own learning experience!
William Li
Courses Plus Student 26,868 PointsWilliam Li
Courses Plus Student 26,868 Pointswhat you wrote is correct, don't put the var keyword, because inside the while loop, you aren't declaring the variable here, you're simply re-assigning a new value to it, the variable was previously declared outside of the loop.
Laurence Foley
16,695 PointsLaurence Foley
16,695 PointsThanks Bryan,
That looks alot better alright, but I'd still like to know why my orginal code was not passing.
Bryan Tidwell
3,473 PointsBryan Tidwell
3,473 PointsAbsolutely, William. Thanks. But just to clarify, if I hadn't declared it before the loop, I couldn't do so inside it, correct?
And Laurence, I think the challenge was mainly upset with the "var" inside the loop and using the "if" as part of the solution. I've found with some of these challenges there are other ways to beat them, but sometimes only one or two solutions will work.
William Li
Courses Plus Student 26,868 PointsWilliam Li
Courses Plus Student 26,868 Pointstechnically speaking, you could, because in Javascript, variable declared with var keyword doesn't not have block scope, and while loop is block scoped.
but the problem here is the while condition
while(password !== secret)
, if you haven't declared the secret variable previously, when it gets to this line, JavaScript will raise an Exception error for undefined variable because it has no idea where secret comes from.Bryan Tidwell
3,473 PointsBryan Tidwell
3,473 PointsThanks! So much clearer now.