Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial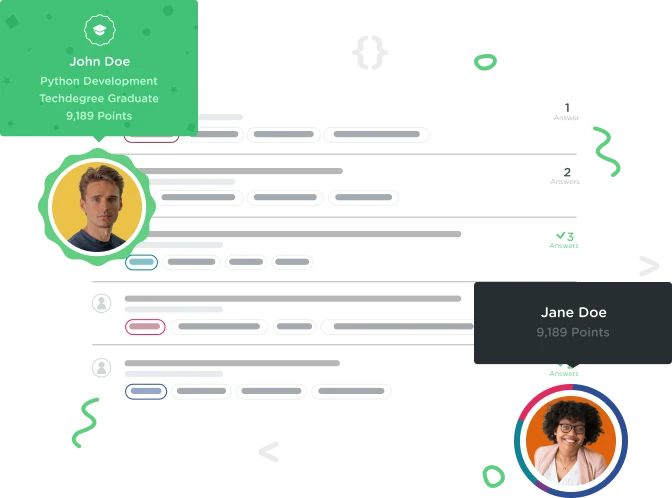

Susan Rusie
10,671 PointsWhy is this code not passing the Super Conditional Challenge?
I have made the changes necessary to get the message "It's Friday, but I don't have enough money to go out." However, it isn't passing. What am I missing? Here is the code I typed in:
var money = 9; var today = 'Friday'
if ( money >= 100 && today === 'Friday' ) {
alert("Time to go to the theater");
} else if ( money >= 50 && today === 'Friday' ) {
alert("Time for a movie and dinner");
} else if ( money > 10 && today === 'Friday' ) {
alert("Time for a movie");
} else if ( today !== 'Friday'|| money === 9) {
alert("It's Friday, but I don't have enough money to go out");
} else {
alert("This isn't Friday. I need to stay home.");
}
var money = 9;
var today = 'Friday'
if ( money >= 100 && today === 'Friday' ) {
alert("Time to go to the theater");
} else if ( money >= 50 && today === 'Friday' ) {
alert("Time for a movie and dinner");
} else if ( money > 10 && today === 'Friday' ) {
alert("Time for a movie");
} else if ( today !== 'Friday'|| money === 9) {
alert("It's Friday, but I don't have enough money to go out");
} else {
alert("This isn't Friday. I need to stay home.");
}
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JavaScript Basics</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
2 Answers
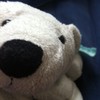
bothxp
16,510 PointsHi Susan,
Take a close look at your line:
else if ( today !== 'Friday'|| money === 9)
hint: You don't need to test the money again at that stage and you want to make sure that it is Friday rather than not.
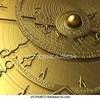
AFIR MOURAD TAHAR
3,766 PointsHi,
the mistake is here: else if ( today !== 'Friday'|| money === 9) { alert("It's Friday, but I don't have enough money to go out"); you are testing if it is not Friday. the correct thing to do is: else if ( today == 'Friday'|| money === 9) { alert("It's Friday, but I don't have enough money to go out");
change !== to ==
A little advice: When you see you have many else if. change to another much powerful method. use switch/case statement:
Example in php. example: <?php
$money=9;
$today = 'Friday';
switch ($money,$today) {
case ($money >= 100 && $today = 'Friday) :
echo "Time for a movie and dinner";
break;
case ($money == 10 && $today = 'Friday):
echo "It's Friday, but I don't have enough money to go out";
break;
}
?>
Susan Rusie
10,671 PointsSusan Rusie
10,671 PointsThanks for your help. I figured out that I needed to add a closing parenthesis and eliminate the part about the money and it passed.