Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial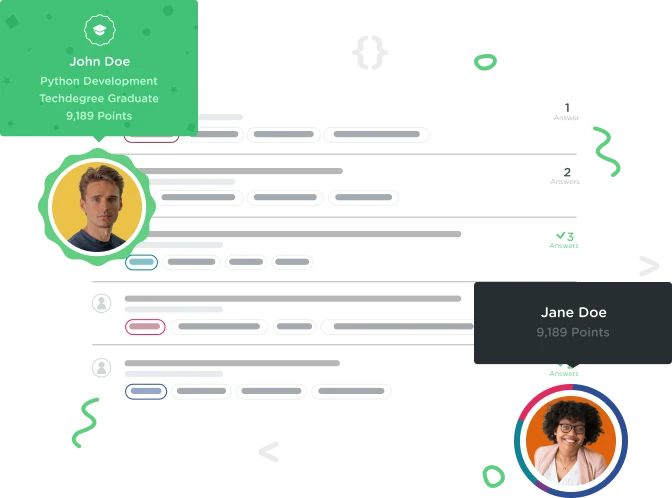

Gerard Gaspard
2,473 PointsWhy is this code not working, it works in OS X terminal with no errors
combo = (['swallow', 'snake', 'parrot'], 'abc')
first_list = list(combo[1])
second_list = combo[0]
new_list= list()
def combo():
count = 0
for x in enumerate(second_list):
f = x[1],first_list[count]
count+=1
new_list.append(f)
return new_list
combo()
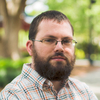
Kenneth Love
Treehouse Guest TeacherNo, because combo
has to take two lists as arguments. It then has to combine those two lists into tuples inside of a list. Yours is using these lists you've created outside of the function.
def combo(list1, list2):
output = []
for index, value in enumerate(list1):
This is how I start it.
5 Answers
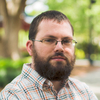
Kenneth Love
Treehouse Guest TeacherYou don't need to define any of the inputs, only the function that'll be called. Because of the way your code is structured, you're affecting new_list
and always returning it. That'd be fine, but you also call your own function with your own inputs later on. That causes the new_list
that gets returned to contain more than just the information it should.
If you're going to use a list like new_list
and append onto it (which is fine), don't put new_list
outside of the function.

Gerard Gaspard
2,473 PointsThanks, That did the trick but I still had to define the inputs within my function.
I am confused with the what "return" does, could you please explain it a bit and also let me know what would be better solution
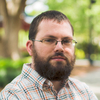
Kenneth Love
Treehouse Guest TeacherHey Gerard Gaspard. return
sends data back out from a function. Consider the following function:
def add_butt(some_string):
some_string += " butt"
This function takes a string and appends the string " butt"
to it. But it only changes the string inside of the function. So doing joke = add_butt("I feel like a")
wouldn't make joke
equal to anything. It would actually be None
. Now let's look at another example:
def add_butt(some_string):
some_string += " butt"
return some_string
Now, if I do joke = add_butt("I feel like a")
, joke
will be "I feel like a butt"
because we returned the changed string out of the function.
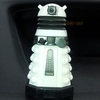
Kevin Franks
12,933 PointsTry changing your function declaration to: definition combo (first_list, second_list): To pass the list to your function. :

Gerard Gaspard
2,473 PointsThank you for your reply. No luck still. Got this error instead from my code challenge. The exact code runs perfect on my computer and I got required list.
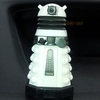
Kevin Franks
12,933 PointsI believe your output is in the wrong order. The test is looking for (first_list, second_list). Also you can eliminate the count by using x[0] for the index.

Gerard Gaspard
2,473 PointsThanks for the index hint, but still no luck, I still get errors, here is my code and screenshot from the code challenge.
combo = (['swallow', 'snake', 'parrot'], 'abc')
first_list = combo [0]
second_list = list(combo[1])
new_list= list()
def combo():
for x in enumerate(first_list):
f = x[1],second_list[x[0]]
new_list.append(f)
return new_list
combo()
Thanks.
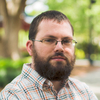
Kenneth Love
Treehouse Guest TeacherYeah, you need to get the first_list
item into the tuple's 0
index.

Gerard Gaspard
2,473 PointsStill no luck, I get this error

Wilfredo Reyes
1,073 PointsHere is how I did it:
def combo(a, b):
output = []
for index in enumerate(a):
output.append((index[1], b[index[0]]))
return output
Gerard Gaspard
2,473 PointsGerard Gaspard
2,473 PointsThanks Kenneth for that explanation for the return function.
so my code for combo() can be modified to: