Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial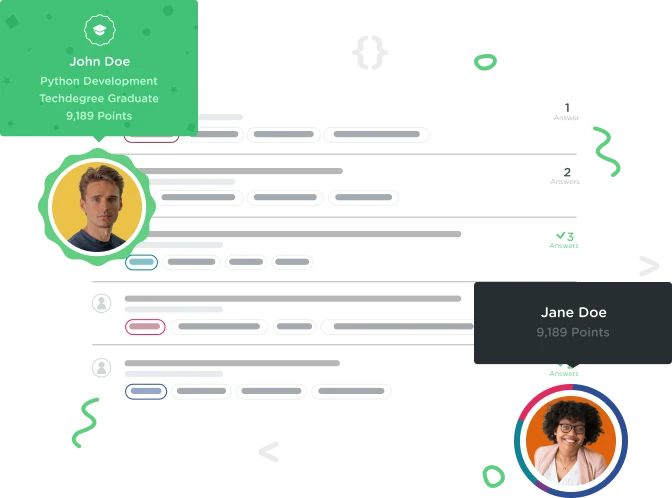

s speir
3,991 PointsWhy is this the correct Math?
Hello!
I don't fully understand part of the math in Dave's solution for the Random Number Challenge.
Here is (basically) his solution :
var input = prompt('Please enter any number.');
var topNumber = parseInt (input);
var input2 = prompt('Please enter another number.')
var bottomNumber = parseInt (input2);
var randomNumber = Math.floor ( Math.random () * (topNumber - bottomNumber + 1) ) + bottomNumber;
var msg = '<h1>' + randomNumber + ' is a number between ' + bottomNumber + ' and ' + topNumber + '.</h1>';
document.write(msg);
I can talk myself through each line of code, except for this one :
var randomNumber = Math.floor ( Math.random () * (topNumber - bottomNumber + 1) ) + bottomNumber;
First : why do you subtract topNumber from bottomNumber, add 1, and then add the bottomNumber again? I understand that it creates the value to multiply against Math.random() so that your random number is between the two user inputs ... I just don't understand how it accomplishes that? (apologies if that's incredibly simple, but I just keep getting lost in it).
Second : In the lesson, Dave creates the variable topNumber because that is supposed to be the highest value from user input. How does labeling it topNumber have anything to do with making sure it's the highest value? You can input 3 for the first prompt (topNumber) and 8905 for the second (bottomNumber), and nothing requires that topNumber is actually the highest number out of the two.
Thank you for any answers!
3 Answers
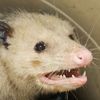
gregsmith5
32,615 Points"First : why do you subtract topNumber from bottomNumber, add 1, and then add the bottomNumber again? I understand that it creates the value to multiply against Math.random() so that your random number is between the two user inputs ... I just don't understand how it accomplishes that? (apologies if that's incredibly simple, but I just keep getting lost in it)."
var topNumber = 10;
var bottomNumber = 2;
With these two parameters, we get all numbers between 2 and 10 (inclusive).. That's 9 possible values, no less than 2. Keep in mind that Math.random()
produces a value between 0 and 1, but never 1.
Math.floor(Math.random() * (topNumber - bottomNumber)) // A value between 0 and 8, but never 8
Simply by multiplying Math.random()
by the sum of topNumber
and -bottomNumber
, and passing that into Math.floor()
, we get 7 possible integer values starting at 0. We want 8 values, so we add 1 to the sum. Because we want the smallest possible value to be 2, and not 0, we have to add bottomNumber
back into our product.
Math.floor(Math.random() * (topNumber - bottomNumber + 1)) + 2 // A whole number between 2 and 10, inclusive

Jason Anello
Courses Plus Student 94,610 PointsHi s speir,
It looks like your second question wasn't answered.
You're right, there is no guarantee that topNumber will be the higher number. We're relying on the user to enter them correctly.
But it needs to be for the formula to work right.
Dave was probably keeping the solution simple to illustrate the concepts.
In a real program, you could have some conditional logic to check if bottomNumber is greater than topNumber which means they were entered in the wrong order. Then you could switch the values if that condition is true.
Something like this:
if (bottomNumber > topNumber) {
var temp = bottomNumber;
bottomNumber = topNumber;
topNumber = temp;
}

AUTIF KAMAL
11,692 PointsI'm just chiming in cause I'm on this challenge right now.
3 (1), 4 (2), 5 (3), 6 (4), 7 (5), 8 (6), 9 (7)
So, that's 7 possible values if exclusive, but I was under the impression that it was supposed to be inclusive.
2 (1), 3 (2), 4 (3), 5 (4), 6 (5), 7 (6), 8 (7), 9 (8), 10 (9)
That's inclusive, so it looks like there are 9 possible values.
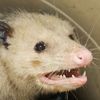
gregsmith5
32,615 PointsI agree. It would help if I paid attention while typing.
s speir
3,991 Pointss speir
3,991 PointsThank you for your answer!
How is between 2 and 10 (inclusive), 8 possible values? Isn't that 9 values counting from 2 - 10 and including both 2 and 10?
Ah, I think I understand : subtracting the Top number from the Bottom number gives you the # of values you want, but restricted by the Math.random command (0-x but never x ... x being 8 in this case). Therefore you just want to get back to the amount of values you want, make sure it's rounded proper (Math.floor), and you add the Bottom number back to make sure that your lowest number possible is indeed the lowest number you received.
It's still seems a mystery as to why exactly this works in terms of the nitty-gritty mathematical / theoretical reasons, but it seems like it's one of those things that just works this way because it does.
thanks!