Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial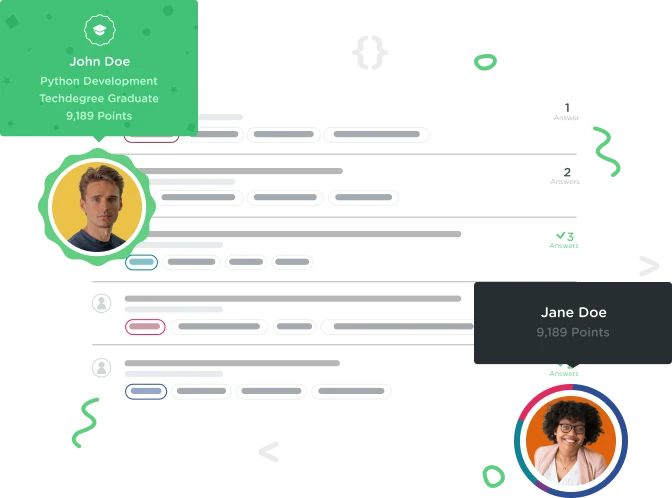

jp28
3,413 PointsWhy is this wrong?
i did everything treehouse told me but it's telling me that task number 1 is no longer passing
import random
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
start = 5
while True:
sat = random.randit(1, 99)
even_odd(sat)
if sat % 2 == 0:
print("{} is even".format(sat))
else:
print ("{} is odd".format(sat))
start = start -1
1 Answer

Daniel Granlund
3,359 PointsHi Avendano,
I can spot a few problems with your code above, however it's a little bit confusing the error message that task number 1 is no longer passing. My pet theory here is that since you have some syntax errors here when you're submitting your results it will start by testing the original challenges -- which will fail.
Anyhow! Here's some pointers:
import random
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
start = 5
while start: # This will enter an infinite loop if you use True, replacing it with the start variable instead.
sat = random.randint(1, 99) # You misspelled it random.randit()
even_odd(sat) # This function call has no purpose as you're not using the result by storing it as a variable and using it in your if statement.
if sat % 2 == 0:
print("{} is even".format(sat))
else:
print ("{} is odd".format(sat))
start = start -1
Adding the changes should make it work for you, but do let me know if you have any questions. Otherwise here's more 'pythonic' way of writing the same thing just for reference:
import random
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
start = 5
while start:
random_int = random.randint(1, 99)
if even_odd(random_int):
print("{} is even".format(random_int))
else:
print("{} is odd".format(random_int))
start -= 1