Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial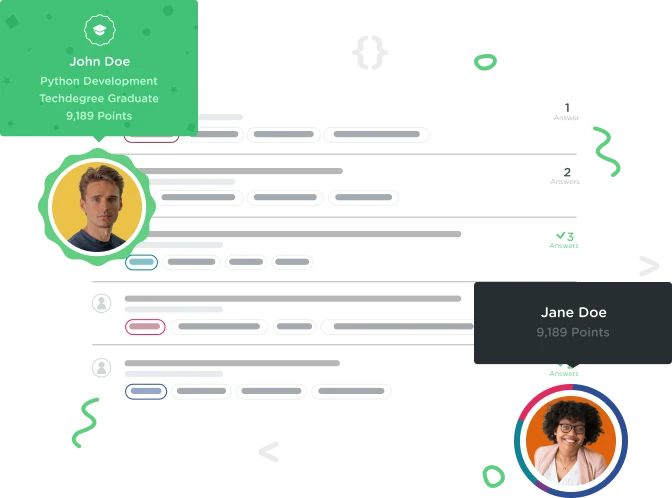
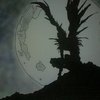
Lucas Santos
19,315 PointsWhy isn't my onSaveInstanceState saving my EditText Value properly when going from landscape back to portrait
So I have seen multiple examples of this done and for some reason I cannot get onSaveInstanceState to save the value of my EditText properly.
I have a very simple example first a TextView, then an EditText followed by a Button.
When clicking the Button it saves the value in EditText and then applies it to the TextView.
So the problem is it only works once, meaning I start in Portrait mode then turn my phone to Landscape and it saves then back to Portrait and it does not so the TextView appears blank.
My Example code is below:
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="50dp"
android:paddingLeft="50dp"
android:paddingRight="50dp"
android:paddingTop="50dp"
tools:context="mydomain.com.savetest.MainActivity">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Default Text"
android:textSize="48sp"
android:id="@+id/textView"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"/>
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/editText"
android:layout_centerVertical="true"
android:layout_centerHorizontal="true"
android:hint="choose a word"/>
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="SAVE WORD"
android:id="@+id/bu"
android:layout_alignParentBottom="true"
android:layout_centerHorizontal="true"/>
</RelativeLayout>
MainActivity.java
package mydomain.com.savetest;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
public class MainActivity extends AppCompatActivity {
private TextView mTextView;
private EditText mEditText;
private Button mButton;
private String editTextValue;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mTextView = (TextView) findViewById(R.id.textView);
mEditText = (EditText) findViewById(R.id.editText);
mButton = (Button) findViewById(R.id.button);
editTextValue = mEditText.getText().toString();
mTextView.setText(editTextValue);
if(savedInstanceState != null){
mTextView.setText( savedInstanceState.getString("text") ); //setting the saved value to the TextView
}
mButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
editTextValue = mEditText.getText().toString();
mTextView.setText(editTextValue);
}
});
}
@Override
protected void onSaveInstanceState(Bundle outState) {
super.onSaveInstanceState(outState);
outState.putString("text", editTextValue); //saving EditText value
}
}
So the whole goal of this is to get the TextView to save the word that was int the EditText and maintain it switching from portrait to landscape. As of now if I type lets say "Car" it will update the TextView with "Car" and will also have the value of Car even turning to Landscape mode, BUT when turning back to portrait the TextView value is gone and has nothing. I Cant seem to figure out whats going on here because I have set the value of EditText to TextView in the beginning on onCreate() so when the app starts again it should take the saved value just fine.
1 Answer
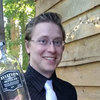
Evan Demaris
64,262 PointsHi Lucas,
I played with your code a bit, and found that deleting all references to onSaveInstanceState and using the below code instead worked well.
@Override
protected void onRestoreInstanceState(Bundle savedInstanceState) {
super.onRestoreInstanceState(savedInstanceState);
editTextValue = mEditText.getText().toString();
mTextView.setText(editTextValue);
}
Because the edittext has a unique value once text has been entered, it will be saved by onSaveInstanceState, and you just need to reassign that unique value to the textview on restore.
Lucas Santos
19,315 PointsLucas Santos
19,315 PointsOk that worked but made absolutely no sense how would it work without "onSaveInstanceState" if "onSaveInstanceState" is what will save the value of EditText? "onRestoreInstanceState" is only for retrieving the saved value from "onSaveInstanceState" which would be a replacement for,
so how would that work if you are not even saving a value? please explain