Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial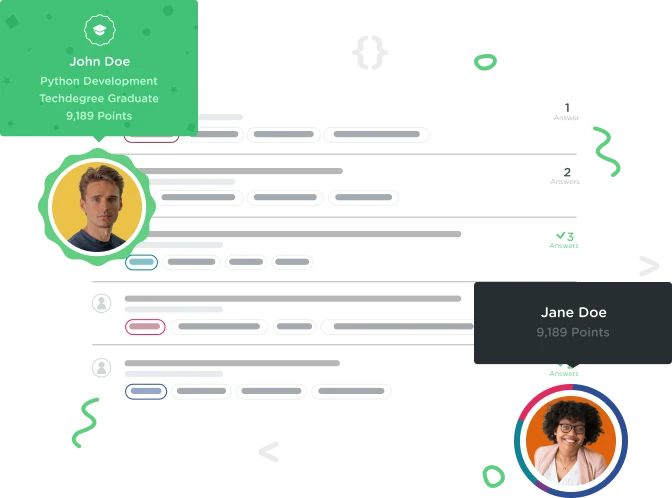

Yewon Stephanie Cho
2,220 Pointswhy isnt this going through?
not sure why this code is not accepted .. it gets the results as specified in the comments
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(s):
results = {}
s = s.lower().split(' ')
for item in s:
results[item] = s.count(item)
print(results)
5 Answers
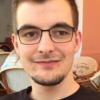
tobiaskrause
9,160 PointsDiffference between split() and split(' ')
split(' ')
def word_count(s):
results = {}
s = s.lower().split(' ')
for item in s:
results[item] = s.count(item)
print(results)
word_count("This is \na \ntest");
OUTPUT: {'\ntest': 1, '\na': 1, 'is': 1, 'this': 1}
split()
def word_count(s):
results = {}
s = s.lower().split()
for item in s:
results[item] = s.count(item)
print(results)
word_count("This is \na \ntest");
OUTPUT: {'this': 1, 'a': 1, 'is': 1, 'test': 1}
And thats why the challanged said you should NOT only split the whitespaces -> Bummer! Hmm, didn't get the expected output. Be sure you're not splitting only on spaces! BTW: if you are not common with \n -> this is a build in String escape character for a new line like
==> https://docs.python.org/2/reference/lexical_analysis.html#string-literals
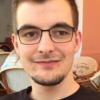
tobiaskrause
9,160 Points# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(str1):
str2 = str1.lower()
new_string = str2.split()
result = {}
for s in new_string:
if s in result:
result[s] += 1
else:
result[s] = 1
return result
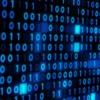
Alexander Davison
65,469 PointsFirst, you shouldn't print out anything. The challenge didn't ask you to print anything
Second, your program only splits on spaces.
If you don't pass in any arguments into the
split
function, it will by default split on all whitespace (the challenge expects you to do this). Whitespace includes: spaces, tabs, newlines, etc.
def word_count(s):
results = {}
s = s.lower().split()
for item in s:
results[item] = s.count(item)
return results
I hope this helps. ~Alex
If this answered your question, Please mark is as a "Best Answer". Thank you!

Yewon Stephanie Cho
2,220 Pointsthank you Tobias for providing the solution but im genuinely curious to see why my code is not accepted as the answer !

Yewon Stephanie Cho
2,220 PointsThanks Alexander and Tobias for the helpful feedback !! :)
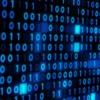
Alexander Davison
65,469 PointsNo problem :)