Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial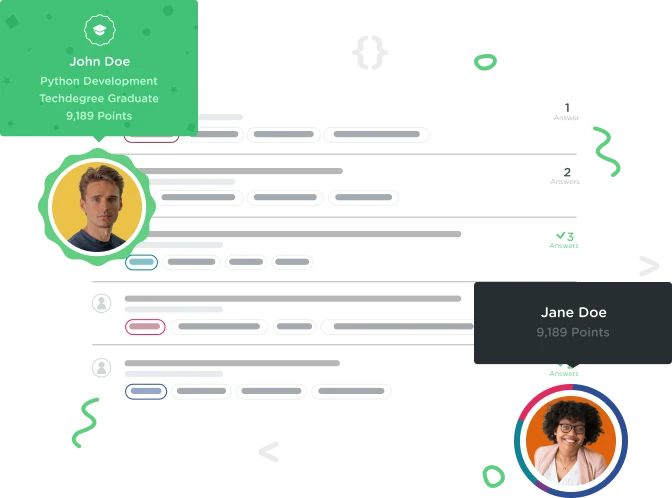

Michel Ortega
2,279 PointsWhy says im using the memberwise initializer?
Im trying to fully understand the methods and objects. What am I doing wrong?
struct RGBColor {
let red: Double
let green: Double
let blue: Double
let alpha: Double
let description: String
// Add your code below
init(red: Double, green: Double, blue: Double, alpha: Double, description: String ){
self.red = red
self.green = green
self.blue = blue
self.alpha = alpha
self.description = "red: \(red), green:\(green), blue:\(blue), alpha:\(alpha)"
}
}
let RGB = RGBColor(red: 33.3, green: 77.7, blue: 13.13, alpha: 23.23, description: "")
2 Answers
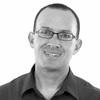
David Papandrew
8,386 PointsHi Michael,
To answer your questions: 1) You could setup the RGB initializer to take a description argument and setup the initializer to set the passed value to the description property, BUT you already have all the strings necessary to construct the description string from the color values. So why not let the code do the "heavy lifting" and generate the description string using the other parameters? It streamlines the code and you only need to capture the essential inputs via a limited set of function parameters.
2) You can call the new RGB object whatever you want. I sometimes call things "my<Whatever>" when I muck around with a Swift playground.
Hope that helps.
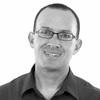
David Papandrew
8,386 PointsHi Michael,
Since the description property will be derived from the other 4 properties, you won't need the description text to be part of the init parameters.
struct RGBColor {
let red: Double
let green: Double
let blue: Double
let alpha: Double
let description: String
// Add your code below
init(red: Double, green: Double, blue: Double, alpha: Double) {
self.red = red
self.green = green
self.blue = blue
self.alpha = alpha
self.description = "red: \(red), green: \(green), blue: \(blue), alpha: \(alpha)"
}
}
Now if you want to obtain see description you could do something like this:
let myRGB = RGBColor(red: 33.3, green: 77.7, blue: 13.13, alpha: 23.23)
print(myRGB.description)

Michel Ortega
2,279 PointsThanks a lot. Two more questions: Why I don't need to pass the description argument when I create the myRGB object, along with the colors? Is it just a convention to call the first object "my.." in this case "myRGB?
xoxo
Michel Ortega
2,279 PointsMichel Ortega
2,279 Pointsthanks a lot