Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial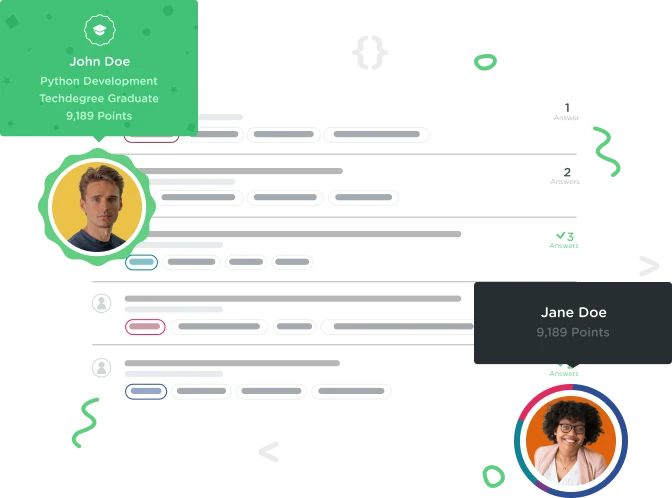
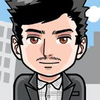
Abdillah Hasny
Courses Plus Student 3,886 Pointswhy this code isn't work
this code work properly as the question command
def loopy(items):
for x in range(len(items)):
if items[0] == "a":
continue
else:
print(items[x])
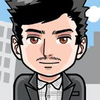
Abdillah Hasny
Courses Plus Student 3,886 Pointsif you make like
def loopy(items):
for item in items:
if 'a' in item:
continue
else:
print(item)
when 'a' is not at index 0 the string still skipped, i just think to check just the index 0 because the question , but thanks for your answer
1 Answer
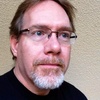
Chris Freeman
Treehouse Moderator 68,441 PointsSince you are using an index as your for loop variable you need to look up the individual item before looking at its index[0] element.
items[0]
is the first element of the items list
items[0][0]
is the first character of the first element of the items list
To correct your code, add the correct indexing:
def loopy(items):
for x in range(len(items)):
if items[x][0] == "a": # <-- added [x]
continue
else:
print(items[x])
A more direct solution would be to operate on the elements of items
directly:
def loopy(items):
for item in items:
if item[0] == "a":
continue
else:
print(item)
This can be simplified by inverting the if
condition:
def loopy(items):
for item in items:
if item[0] 1= "a": # print if NOT starts with 'a'
print(item)
Luke Mason
1,596 PointsLuke Mason
1,596 PointsThere's not one right way to do it, but here's how I did mine:
''' python <p> def loopy(items): for item in items: if 'a' in item: continue else: print(item) </p> '''
What this does is takes a list as a parameter for the function "loopy". Then sets a temporary iterator named item. Each value in the list "items" will be assigned one at a time to the iterator "item". Then in the if statement each "item" will be checked to see if it contains the value 'a'. If it does, the loop continues. If it does not contain 'a' then we print out the value of the item.
I'm no python expert, so you're code looks like it'd work to me. But you're calling methods that are a bit overboard. You don't need to check values in a range or even pass the length of the list of items.
the "for x in items" would iterate through the list items until the end by default. No need to spell it out further in the code. :-) And your final print statement is too specific in the wrong way. You don't need the "x" index of list "items". You just need to print "x". X is not an index value, it is temporarily holding a list item from the list "items".