Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial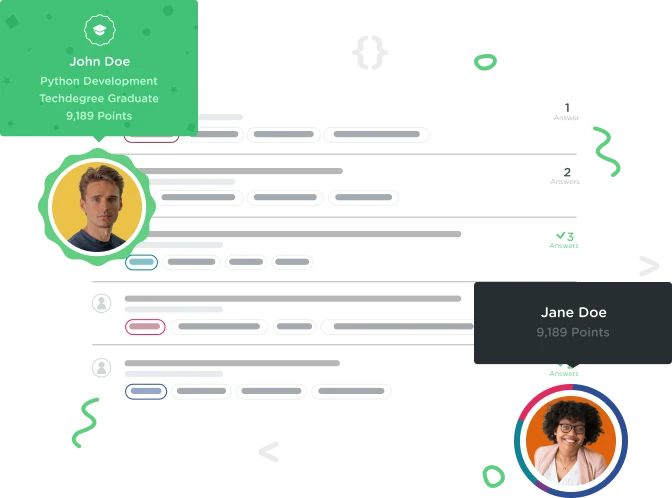

ammarkhan
Front End Web Development Techdegree Student 21,661 PointsWhy use [] around object literals
Just wondering why we should use
[
{
"name": "Ammar",
"inoffice": "no"
}
]
and why not
{
{
"name": "Ammar",
"inoffice": "no"
}
}
what is the difference?
2 Answers

andren
28,558 PointsSquare brackets [] are used to denote an array (list) of values, while curly braces {} are used to denote objects. This is the case both in JavaScript and JSON. They are by no means interchangeable. Arrays store single values separated by a comma, while objects store key-value pairs separated by commas.
Your first example is an array that contains one object, your second example is an object with the definition of another object inside, which is invalid syntax.
You don't need to wrap objects in an array if you only want a single object, but if you are grouping multiple objects like is done in the lecture then an array is needed.

Jeff Sanders
Courses Plus Student 9,503 PointsAnother key advantage of returning an array of objects is that you can iterate over said array of objects by referencing the array's indices, like someArray[0] or someArray[200]
ammarkhan
Front End Web Development Techdegree Student 21,661 Pointsammarkhan
Front End Web Development Techdegree Student 21,661 PointsThanks.
ammarkhan
Front End Web Development Techdegree Student 21,661 Pointsammarkhan
Front End Web Development Techdegree Student 21,661 Pointsandren I see two JSON, one is
and other
Is accessing them the same?
andren
28,558 Pointsandren
28,558 PointsIn the first example you have an object inside of an array, in the second example you have an object which has a property (
jobs
) which stores an array, which itself stores an object, and that object has a property (employer
) which also stores an object. It is a good example of how you can nest arrays and objects freely to create pretty complex structures in JavaScript and JSON .As far as accessing it, you access them the same way you would access an array or an object in JavaScript normally. That is by using bracket notation with an index to pull out items from arrays, and using either dot notation or bracket notation to pull property values out of objects. I can demonstrate it by giving some code examples, for all of these examples I'm assuming the JSON has been stored in a variable called
json
.Let's say I wanted to access the
name
property in your first example, the code would look like this:Let's say I wanted to access the
title
property in your second example that would look like this:Let's say I wanted to access the
name
property in your second example that would look like this:As you can see once you start nesting multiple arrays and objects inside of each other you end up making it more complicated to access them. It can be hard to wrap your mind around in the beginning, but once you have spend some time working with JSON it starts to become easier to conceptualize how it is structured and how you can access the various properties.