Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial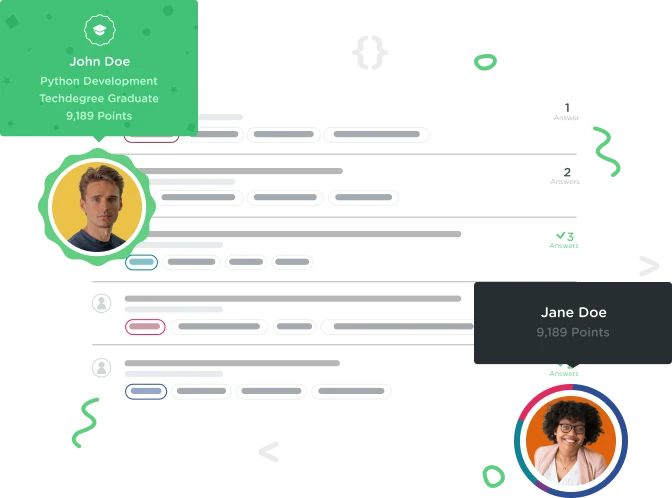

Jordan Olson
6,155 PointsWhy use 'use' when we can just pass the variable into the actual function?
<?php
$name = 'Mike';
$greet = function($name){ echo "Hello, $name!"; };
$greet();
VS
<?php
$name = 'Mike';
$greet = function() use($name){ echo "Hello, $name!"; };
$greet();
1 Answer
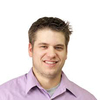
Kevin Korte
28,149 PointsBecause you can't always pass in all the variables you need into a function.
First, there is a small error to correct.
It should be
$name = 'Mike';
$greet = function($name){ echo "Hello, $name!"; };
$greet($name);
Otherwise, $greet
doesn't know what you're passing to it.
With that side, this is using the concept of closure. The second example, is passing a copy of the variable from outside it's scope into the variable.
Take for instance, you wanted to use array_walk()
. http://php.net/manual/en/function.array-walk.php The callback typically only allows 2 parameters. If you need more than two parameters passed into the function, you could use the use closure to pass a copy of those variables in so you could do whatever you needed to do.
I think this is helpful: http://stackoverflow.com/questions/10692817/whats-the-difference-between-closure-parameters-and-the-use-keyword