Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial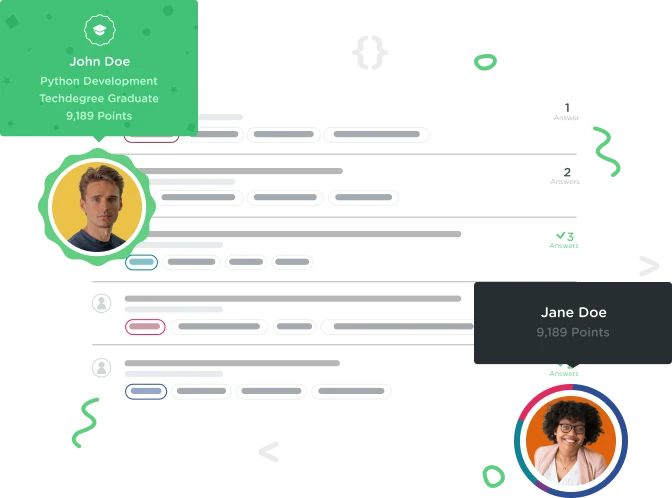
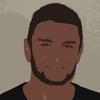
jsdevtom
16,963 PointsWhy, when I store employeeList as a variable, does it not want to change the inner HTML of the list later in the code?
My code works perfectly when I copy his code. I just want to be able to select the div with the id of employeeList in javascript, store it in a variable and call innerHTML on it.
var list = document.getElementById('employeeList');
///code inbetween
list.innerHTML = statusHTML;
JavaScript console returns with:
"Uncaught TypeError: Cannot set property 'innerHTML' of nullxhr.onreadystatechange"
Please tell me for what reason this doesn't work and I will learn it (again).

Emmanuel Plouvier
11,919 PointsYour error are not in this lines of code. Check any misspelling with the id you use.
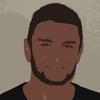
jsdevtom
16,963 PointsCode that works:
var xhr = new XMLHttpRequest();
xhr.onreadystatechange = function () {
if(xhr.readyState === 4) {
var employees = JSON.parse(xhr.responseText);
var statusHTML = "<ul class='bulleted'>";
for (i=0; i<employees.length; i++) {
if (employees[i].inoffice === true) {
statusHTML += "<li class='in'>";
} else {
statusHTML += "<li class='out'>";
}
statusHTML += employees[i].name;
statusHTML += "</li>";
}
statusHTML += "</ul>";
document.getElementById('employeeList').innerHTML = statusHTML;
}
};
xhr.open('GET', 'data/employees.json');
xhr.send();
Code that doesn't works:
var xhr = new XMLHttpRequest();
var list = document.getElementById('employeeList');
xhr.onreadystatechange = function () {
if(xhr.readyState === 4) {
var employees = JSON.parse(xhr.responseText);
var statusHTML = "<ul class='bulleted'>";
for (i=0; i<employees.length; i++) {
if (employees[i].inoffice === true) {
statusHTML += "<li class='in'>";
} else {
statusHTML += "<li class='out'>";
}
statusHTML += employees[i].name;
statusHTML += "</li>";
}
statusHTML += "</ul>";
list.innerHTML = statusHTML;
}
};
xhr.open('GET', 'data/employees.json');
xhr.send();
1 Answer

Erik Nuber
20,629 PointsLooks like you can do this if you move where you are declaring the variable. I played around trying to find out what list was defined as and, if I call your variable within the onreadystatechange area, you can do this properly. Likely has to do with scope so this will work based on your code.
var xhr = new XMLHttpRequest();
xhr.onreadystatechange = function () {
if(xhr.readyState === 4) {
var list = document.getElementById('employeeList');
var employees = JSON.parse(xhr.responseText);
var statusHTML = "<ul class='bulleted'>";
for (i=0; i<employees.length; i++) {
if (employees[i].inoffice === true) {
statusHTML += "<li class='in'>";
} else {
statusHTML += "<li class='out'>";
}
statusHTML += employees[i].name;
statusHTML += "</li>";
}
statusHTML += "</ul>";
list.innerHTML = statusHTML;
}
};
xhr.open('GET', 'data/employees.json');
xhr.send();
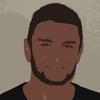
jsdevtom
16,963 PointsThanks
Samuel Webb
25,370 PointsSamuel Webb
25,370 PointsWhere are you running this code and is there an element with the id
employeeList
on that page?