Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial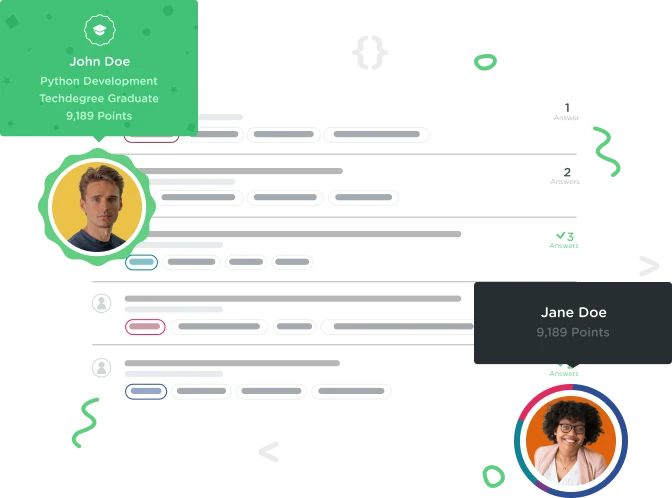

Jesus Bayona
4,937 PointsWhy won't this work?
//Intro
var score = 0;
alert("Hello! Welcome to the quiz! Five questions will be asked. Please answer the best you can and your score will be tracked.");
//concatenation of correct answer
var goodJob = "Good job! Your score is " + score + ".";
//concatenation of wrong answer
var badJob = "Sorry..., wrong answer. Your score remains at " + score + ".";
//concatenation of conclusion
var conclusion = "<p>Your final score is " + score + ". " + crown + "</p>";
//crown is defaulted to no crown
var crown = "You received no crown.";
//define crowns
var bronzeCrown = "You received the bronze crown.";
var silverCrown = "Good job! You received the silver crown.";
var goldCrown = "Excellent job! You received the gold crown!!";
//first question
var asterisk = prompt("Shift 8 on the keyboard produces what symbol?");
if (asterisk === "*") {
score = 1;
alert(goodJob);
} else {
score=0;
alert(badJob);
}
//second question
var tallahassee = prompt("What is the capital of Florida?");
if (tallahassee.toUpperCase() === "TALLAHASSEE") {
score += 1;
alert(goodJob);
} else {
alert(badJob);
}
//third question
var seconds = prompt("How many seconds are in a minute?");
if (seconds === "60" || seconds.toUpperCase() === "SIXTY") {
score += 1;
alert(goodJob);
} else {
alert(badJob);
}
//fourth question
var days = prompt("How many days are there in a week?");
if (days === "7" || days.toUpperCase() === "SEVEN") {
score += 1;
alert(goodJob);
} else {
alert(badJob);
}
//fifth question
var bible = prompt("How many books are there in the Bible?");
if (bible === "66" || bible.toUpperCase() === "SIXTY SIX" || bible.toUpperCase() === "SIXTY-SIX") {
score += 1;
alert(goodJob);
} else {
alert(badJob);
}
//final
if (score === 5) {
crown = goldCrown;
document.write(conclusion);
} else if (score === 3 || score === 4){
crown = silverCrown;
document.write(conclusion);
} else if (score === 2) {
crown = bronzeCrown;
document.write(conclusion);
} else {
document.write(conclusion);
}
3 Answers

Jesus Bayona
4,937 PointsNOW! It's working...
//Intro
var score = 0;
alert("Hello! Welcome to the quiz! Five questions will be asked. Please answer the best you can and your score will be tracked.");
//concatenation of correct answer
var goodJob = "Good job! Your score is ";
//concatenation of wrong answer
var badJob = "Sorry..., wrong answer. Your score remains at ";
//concatenation of conclusion
var conclusion = "Your final score is ";
//crown is defaulted to no crown
var crown = ". You received no crown.";
//define crowns
var bronzeCrown = ". You received the bronze crown.";
var silverCrown = ". Good job! You received the silver crown.";
var goldCrown = ". Excellent job! You received the gold crown!!";
//first question
var asterisk = prompt("Shift 8 on the keyboard produces what symbol?");
if (asterisk === "*") {
score = 1;
alert(goodJob + score);
} else {
score=0;
alert(badJob + score);
}
//second question
var tallahassee = prompt("What is the capital of Florida?");
if (tallahassee.toUpperCase() === "TALLAHASSEE") {
score += 1;
alert(goodJob + score);
} else {
alert(badJob + score);
}
//third question
var seconds = prompt("How many seconds are in a minute?");
if (seconds === "60" || seconds.toUpperCase() === "SIXTY") {
score += 1;
alert(goodJob + score);
} else {
alert(badJob + score);
}
//fourth question
var days = prompt("How many days are there in a week?");
if (days === "7" || days.toUpperCase() === "SEVEN") {
score += 1;
alert(goodJob + score);
} else {
alert(badJob + score);
}
//fifth question
var bible = prompt("How many books are there in the Bible?");
if (bible === "66" || bible.toUpperCase() === "SIXTY SIX" || bible.toUpperCase() === "SIXTY-SIX") {
score += 1;
alert(goodJob + score);
} else {
alert(badJob + score);
}
//final
if (score === 5) {
crown = goldCrown;
} else if (score === 3 || score === 4){
crown = silverCrown;
} else if (score === 2) {
crown = bronzeCrown;
}
document.write("<p>" + conclusion + score + crown + "</p>");
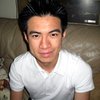
jason chan
31,009 PointsIt's perfectly fine and working.
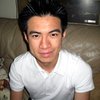
jason chan
31,009 PointsI've edited the post for better reading of source.