Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial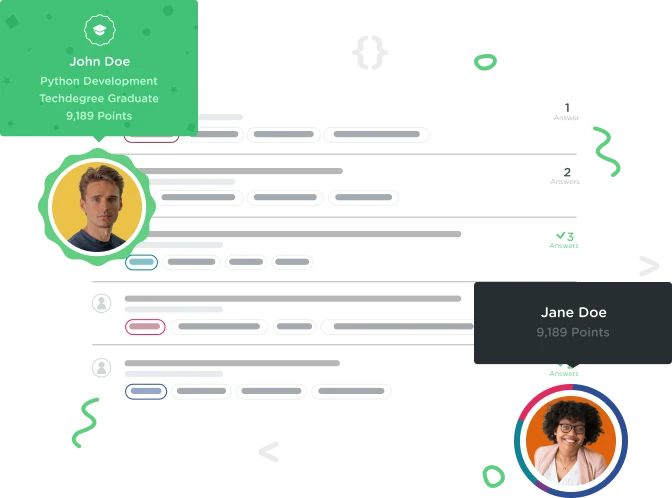
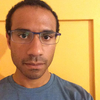
Joe Williams
16,945 PointsWhy would I not split only on white spaces?
In the Word Count code challenge in Python Collections; My code below works on my local console to produce the desired dictionary output but it doesn't pass the challenge. It tells me it's not the output it's looking for and to make sure I'm not splitting only on white spaces. Why would I not split on white spaces in this example? Given than spaces denote words, which is what I'm, trying to separate and count.
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(string):
string.lower()
word_list = string.split()
counter = 0
occurances = []
for word in word_list:
occurances.append(word_list.count(word_list[counter]))
counter += 1
output = dict(zip(word_list, occurances))
return output
3 Answers
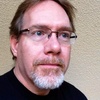
Chris Freeman
Treehouse Moderator 68,441 PointsJoe, you are VERY close! strings are immutable (can't be changed) meaning the string.lower()
does not change the string "in place" but rather returns the lowercased string. Fix by assigning the return value back to string:
string = string.lower()
Edit: typo on Joe, sorry
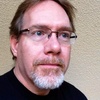
Chris Freeman
Treehouse Moderator 68,441 PointsThinking more on this, if lowercasing the input string is the difference between pass and fail, then "Lowercase the string to make it easier." Isn't a soft suggestion, it's a requirement. Perhaps the task instructions should explicitly state something like "The keys in the dictionary will be each of the words in the string in lowercase"
Tagging Kenneth Love for review
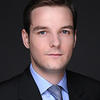
Bogdan Lalu
6,419 PointsI am also having an issue with this challenge. You need to separate on non spaces if the string is "Hello! I like to say hello to everyone I meet." If you only separate on spaces, you'll have "hello!" and "hello" as two separate words
I've even used regular expressions to solve this and create a list of words (word = a sequence of characters a-z) from a string that has, for example two sentences. My solution works great on my local console but the challenge won't accept it.
here's my code below (not accepted by the challenge)
import re
def word_count(mystring):
result = {}
lower_mystring = mystring.lower()
initial_word_list = re.findall(r'\w+', lower_mystring)
unique_word_list = list(set(initial_word_list))
for word in unique_word_list:
result[word] = initial_word_list.count(word)
return result
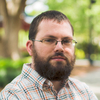
Kenneth Love
Treehouse Guest TeacherYours isn't passing because you're not following the instructions. By searching for \w+
, you'll miss words that contain punctuation, for example. You brought the "word = a sequence of characters a-z" definition into this all by yourself :)
Your code sample did lead to me improving the checking of the output, though, so thanks for that!
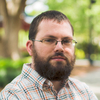
Kenneth Love
Treehouse Guest TeacherI'm just going to highlight part of Joe's code:
counter = 0
occurances = []
for word in word_list:
occurances.append(word_list.count(word_list[counter]))
counter += 1
Unless I'm reading this wrong (and it is the end of the day), won't this increase the counter for every word that comes through? So with a sentence like "hello my name is Hello"
, I'd end up with {"hello": 4, "my": 1, "name": 2, "is", 3}
, right?
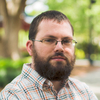
Kenneth Love
Treehouse Guest TeacherActually, yeah, it is the end of the day and I read that completely wrongly.
Joe's code is fine once he correctly lowercases the string. It's an interesting way of solving this, and not how I'd do it, but it passes the grader.
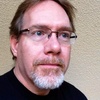
Chris Freeman
Treehouse Moderator 68,441 PointsIt is late: {'my': 1, 'name': 1, 'is': 1, 'hello': 2}
While not the most efficient, the code recounts the word occurrence each time a word reappears.
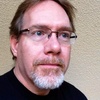
Chris Freeman
Treehouse Moderator 68,441 PointsSo about the "requirement" for lowercase keys.... Does that need to be more explicit?
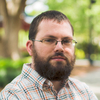
Kenneth Love
Treehouse Guest TeacherI've added a bit of a firmer note re: the lowercased keys. They've been displayed in provided code hint the entire time but a bit more explanation never hurts.
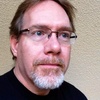
Chris Freeman
Treehouse Moderator 68,441 PointsThanks! You're the best!!
Joe Williams
16,945 PointsJoe Williams
16,945 PointsThanks to Kenneth, Chris, Bogdan and everyone who looked at this for me.
I'm in England so I posted this just before I went for bed and woke up to a really great wealth of discussion and tips.
The support is much appreciated.