Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial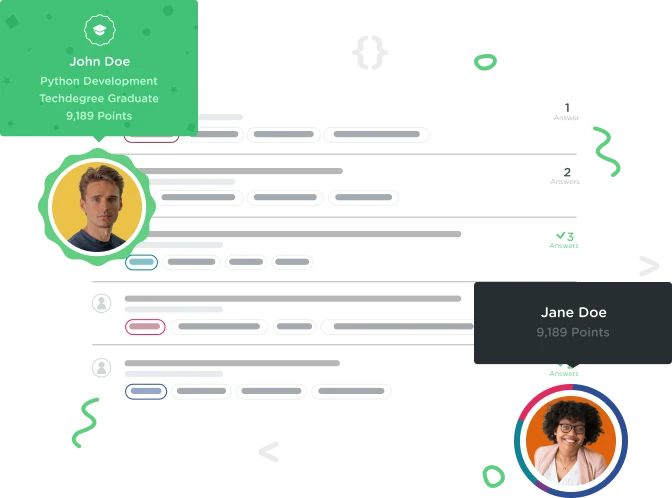
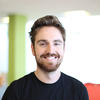
Kristian Woods
23,414 PointsWhy would you want to change the name of the parameter?
I can follow along with the video, that is, until she changes the parameter name...
The function that was created to display HTML to the page:
<?php
function get_item_html($id, $item) {
$output = "<li><a href='#'><img src='"
. $item["img"] . "' alt='"
. $item["title"] . "' />"
. "<p>View Details</p>"
. "</a></li>";
return $output;
}
?>
This function is then used with a foreach loop:
<?php
$random = array_rand($catalog, 4);
foreach($random as $id) {
echo get_item_html($id, $catalog[$id]);
}
?>
Then the output becomes:
$output = "<li><a href='details.php?id="204"'><img src='"
. $catalog[204]["img"] . "' alt='"
. $catalog[204]["title"] . "' />"
. "<p>View Details</p>"
. "</a></li>";
How can you get away with using different parameter names?
Can someone explain what is happening?
Thank you
1 Answer
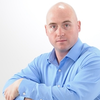
Benjamin Payne
8,142 PointsHey Kristian,
Have a look at this Stack Overflow question. Basically, the function definition defines the parameters to be used inside the function. So where you defined get_item_html($id, $item)
anything inside this function has to refer to $id
or $item
. When you are actually calling that function and passing arguments to it, you can call the arguments whatever you want or just pass the appropriate type directly into the function. For example:
<?php
function foo($hello, $world)
{
echo $hello . ' ' . $world;
}
foo ("hello", "world");
// or
$bar = "hello"; // argument 1
$baz = "world"; // argument 2
foo ($bar, $baz);
Hopefully that helps.
Thanks,
Ben