Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial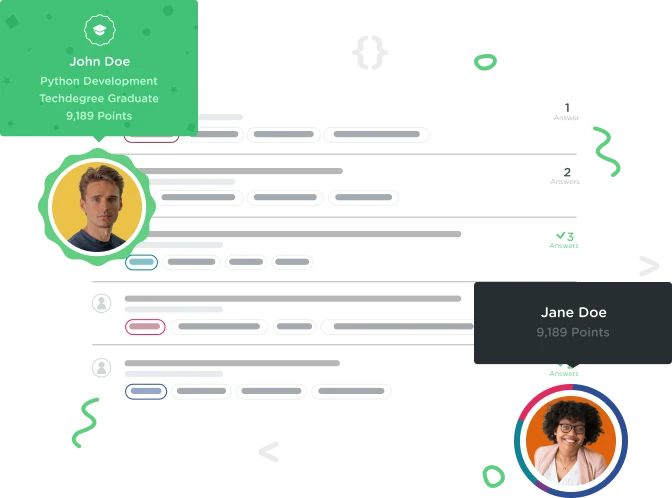

dlpuxdzztg
8,243 PointsWith this code, how do I add an item to a list activity from a fragment?
Hello.
I'm having trouble trying to add something to my list activity from my fragment. I believe that you create a mListView member field in your fragment, but I'm clueless as where to go from there. I thought sharing most of the code for my fragment and activity would help:
Fragment:
@Nullable
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
View view = inflater.inflate(R.layout.fragment_new_note, container, false);
mNoteTitleEdit = (EditText) view.findViewById(R.id.NoteTitleEdit);
mNoteDescriptionEdit = (EditText) view.findViewById(R.id.NoteDescriptionEdit);
mConfirmImageNN = (ImageView) view.findViewById(R.id.ConfirmImageNN);
mConfirmImageNN.setOnClickListener(this);
mCancelImageNN = (ImageView) view.findViewById(R.id.CancelImageNN);
mCancelImageNN.setOnClickListener(this);
mListView = (ListView) view.findViewById(android.R.id.list);
return view;
}
@Override
public void onClick(View v) {
if (v == mConfirmImageNN) {
String titleText = mNoteTitleEdit.getText().toString();
String descriptionText = mNoteDescriptionEdit.getText().toString();
if (v == mConfirmImageNN && titleText.equals("")) {
noteError();
} else if (v == mConfirmImageNN && descriptionText.equals("")){
noteError();
} else {
Note note = new Note(titleText, descriptionText);
goBack();
}
}
if (v == mCancelImageNN) {
goBack();
}
}
Activity:
private ListView mListView;
private TextView mEmpty;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mListView = (ListView) findViewById(android.R.id.list);
mEmpty = (TextView) findViewById(android.R.id.empty);
ListAdapter adapter = new ListAdapter(this, mList);
mListView.setAdapter(adapter);
mListView.setEmptyView(mEmpty);
}
Thanks!

dlpuxdzztg
8,243 PointsThere is no ListView in my fragment layout, I only initialized it because I thought I needed to do so in order to achieve what I wanted. Also, the fragment belongs to a different activity. I want the info from the fragment to be displayed in my main activity's ListView.
2 Answers
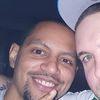
Joe Brown
21,465 PointsYou can pass any sort of data as an Intent extra. Even custom objects you created yourself, you would just need to take an extra step inside that class to being able to do that , but any sort of primitive type(int(or Integer), boolean(or Boolean), etc, is already built in. It is explained in one of the courses here and you can google passing intent extras and read about it on the android dev site

dlpuxdzztg
8,243 PointsAlright, so I think it's working but I'm getting an error concerning the ListAdapter now. But for now, thanks for everything! In case you do want to help, I'll leave the text from the logcat:
FATAL EXCEPTION: main
Process: com.x.x, PID: 560
java.lang.RuntimeException: Unable to start activity ComponentInfo{com.x.x/com.x.x.activities.MainActivity}: java.lang.NullPointerException: Attempt to invoke virtual method 'int java.util.ArrayList.size()' on a null object reference
at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2331)
at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2393)
at android.app.ActivityThread.access$800(ActivityThread.java:151)
at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1309)
at android.os.Handler.dispatchMessage(Handler.java:102)
at android.os.Looper.loop(Looper.java:135)
at android.app.ActivityThread.main(ActivityThread.java:5351)
at java.lang.reflect.Method.invoke(Native Method)
at java.lang.reflect.Method.invoke(Method.java:372)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:908)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:703)
Caused by: java.lang.NullPointerException: Attempt to invoke virtual method 'int java.util.ArrayList.size()' on a null object reference
at com.diego.smartnotes.adapters.ListAdapter.getCount(ListAdapter.java:32)
at android.widget.ListView.setAdapter(ListView.java:487)
at com.diego.smartnotes.activities.MainActivity.onCreate(MainActivity.java:48)
at android.app.Activity.performCreate(Activity.java:6020)
at android.app.Instrumentation.callActivityOnCreate(Instrumentation.java:1105)
at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2284)
at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2393)
at android.app.ActivityThread.access$800(ActivityThread.java:151)
at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1309)
at android.os.Handler.dispatchMessage(Handler.java:102)
at android.os.Looper.loop(Looper.java:135)
at android.app.ActivityThread.main(ActivityThread.java:5351)
at java.lang.reflect.Method.invoke(Native Method)
at java.lang.reflect.Method.invoke(Method.java:372)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:908)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:703)
ListAdapter:
public class ListAdapter extends BaseAdapter {
private Context mContext;
private ArrayList<Object> mList;
private Note[] mNotes;
public ListAdapter(Context context, ArrayList<Object> list) {
mContext = context;
mList = list;
}
@Override
public int getCount() {
return mList.size();
}
@Override
public Object getItem(int position) {
return mList.indexOf(position);
}
@Override
public long getItemId(int position) {
return 0;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
NoteViewHolder noteHolder;
if (convertView == null) {
// Brand new
convertView = LayoutInflater.from(mContext).inflate(R.layout.note_list_item, null);
noteHolder = new NoteViewHolder();
noteHolder.NoteListItemImage = (ImageView) convertView.findViewById(R.id.NoteListItemImage);
noteHolder.NoteListItemText = (TextView) convertView.findViewById(R.id.NoteListItemText);
noteHolder.DeleteNoteImage = (ImageView) convertView.findViewById(R.id.DeleteNoteImage);
convertView.setTag(noteHolder);
} else {
noteHolder = (NoteViewHolder) convertView.getTag();
}
Note note = mNotes[position];
noteHolder.NoteListItemText.setText(note.getTitle());
return convertView;
}
private static class NoteViewHolder {
ImageView NoteListItemImage; // Public by default.
TextView NoteListItemText;
ImageView DeleteNoteImage;
}
}
Thanks once again!
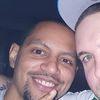
Joe Brown
21,465 PointsI think I am correctly visualizing what you're doing then. You dont need to get a reference to the Activity ListView in the other Activity's fragment then. So you have, say, your MainActivity with a ListView, then you start the other Activity that has the fragment, and you want to be able to get data from that fragment over into MainActivity for use in the ListView there, correct? If thats the case you could just pass data from the fragment as an Intent extra back to MainActivity. You would typically do this through an interface you create between that fragment and its own containing Activity, that Activity would then start your MainActivity and pass the Intent data. Then in MainActivity just call getIntent(), check for null, and pull out your data and add it to the adapter data and update the List. Worst case post a link to a github repo if you have one so I can see the full picture. What you're trying to do should be pretty straight forward.

dlpuxdzztg
8,243 PointsThanks! But what if the data I want to pass from the fragment to the activity isn't a string?
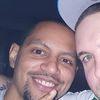
Joe Brown
21,465 PointsYou are crashing because your ArrayList object is null. You are passing one into your constructor. Where are you initializing your List?
Joe Brown
21,465 PointsJoe Brown
21,465 PointsYou have a ListView in your fragment layout and your Activity layout? Are you adding that fragment to the activity you showed there somewhere? You would typically add the fragment to the activity and the fragments layout file would have the ListView, and in its onCreateView you would set the adapter and the like.