Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial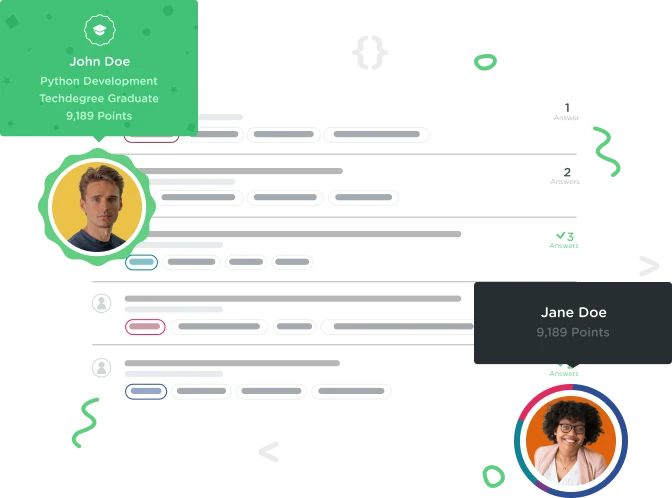
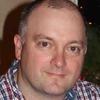
jonlunsford
15,480 PointsWordcount.py not passing even though I get the same answer as the example provided when running this in a Python IDE.
Here is my code:
def word_count(value):
newdict = {} # create an empty dict to store values
value_lower = value.lower() # lower the words in the value string
words_list = value_lower.split(' ') # split the words in the value string
for word in words_list:
if word in newdict: # if the word is already in the dict, increment it's count
newdict[word] += 1
else: # set its initial value to 1
newdict[word] = 1
return newdict # return the new dictionary
If I run this in my local instance of Python, and pass in the following string "I do not like it Sam I Am". I get the same result as the example.
1 Answer

andren
28,558 PointsThe issue is that the challenge asks you to split on all whitespace. While a space is an example of whitespace it is not the only example: tabs, line breaks, multiple spaces and other things like that are also classified as whitespace.
The example argument the task show you only contains single spaces which is why that works as intended, but a string containing different whitespace, like a line break for example would fail.
Conveniently enough the split
method will actually split on all whitespace by default if you don't pass it any arguments, so if you remove your argument to it like this:
def word_count(value):
newdict = {} # create an empty dict to store values
value_lower = value.lower() # lower the words in the value string
words_list = value_lower.split() # split the words in the value string on ALL whitespace
for word in words_list:
if word in newdict: # if the word is already in the dict, increment it's count
newdict[word] += 1
else: # set its initial value to 1
newdict[word] = 1
return newdict # return the new dictionary
Then your code will work.
jonlunsford
15,480 Pointsjonlunsford
15,480 PointsThanks Andren. I guess the devil's in the details.