Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial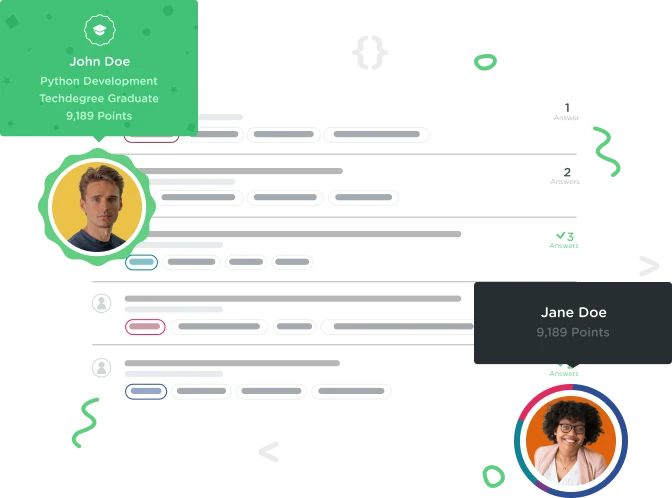

Dan Nguyen
4,665 PointsWorking code for Extra Challenge at the End - What do you think of my solution?
Hello, this was my code to find out if there was more than 1 student with the same names. I replaced Jordan with Judy. So, if you test it out... make sure you have students with the same name.
Basically, my solution was to push found students to an array. Similar to a previous exercise. Once this array was built, then run a for loop to print out the results.
For some reason the search === null doesn't work in safari...
var message = '';
var student;
var search;
var flag = true;
var foundStudents = [];
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport ( student ) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
while(flag){
search = prompt('Search student records or type quit to quit');
if(search === null || search === 'quit') {
break;
}
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if(student.name.toLowerCase() === search.toLowerCase()) {
foundStudents.push(student);
}
}
flag = false;
}
for(var j = 0; j < foundStudents.length; j++) {
message += getStudentReport(foundStudents[j]);
}
print(message);
3 Answers
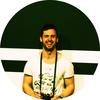
Malte Niepel
19,212 PointsJust one thing: If there is no student with this name, you could add this else statement.
But nice job!
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if(student.name.toLowerCase() === search.toLowerCase()) {
foundStudents.push(student);
}
else {
message = "This student does not exist.";
}

Braden Boaglio
9,375 PointsNice job on this.

Kevin Jenkins
12,630 PointsAgree - nice job. Part of the reason this works is that you moved print(message) outside the for loop. The print function we're using, with outputDiv.innerHTML replaces the entire div. The computer actually shows both results, but one after another so quickly we can't see it. When you build the message from all students with the same name, then print, you get to see them all at once.
I realized this when looking at the while statement as written by Dave. Since it's already looping through the full array, it should already have found all the students with the same name, right? It's just that the print and getStudentReport functions effectively hide all but one.
Dan Nguyen
4,665 PointsDan Nguyen
4,665 PointsThanks! Completely forgot about that part in this reiteration.