Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial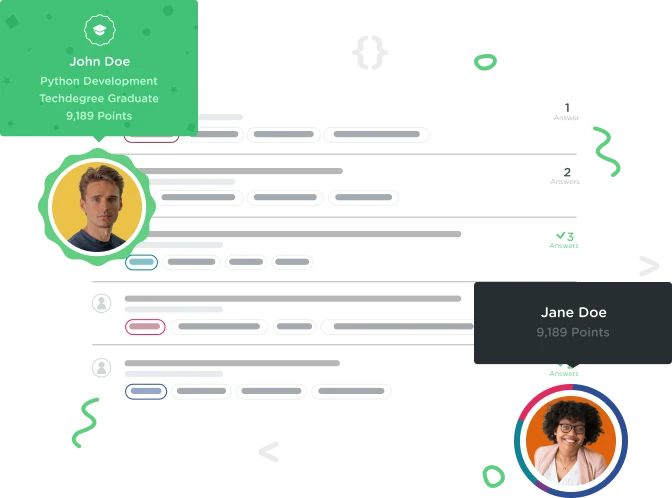

bpietravalle
16,718 PointsWrite a function named courses that takes the dictionary of teachers. It should return a list of all of the courses...
Can anyone provide a hint regarding part 4 of challenge? I'm having trouble looping through dict to separate courses from the teachers.
At first I tried:
def courses(teachers):
total_courses = []
total_courses.append(teachers.values())
return total_courses
This returns an error (paraphrased): "only find 1 item in list, should be 18"
Since the values (ie the courses) are another list, I assume you need loop through and use pop() or unpack the dict from the beginning. Here's what I've tried, but still no luck:
def courses():
total_courses = []
final_list = []
count = 0
for x in teachers:
total_courses.append(teachers[x])
while count <= len(total_courses:
print(total_courses)
z = total_courses.pop(0)
final_list.append(z)
count += 1
else:
final_list.append(total_courses.pop(0))
break
print(final_list)
courses()
Anyways, thanks for your help.
14 Answers

boog690
8,987 PointsHello:
The problem asks you to return a list of of all courses. Your function is not returning anything, it's printing. It should be returning the list with the list of all courses.
To get a list of all the courses, we'll need a nested for loop that'll (1st for loop) iterate through the dictionary and THEN (2nd for loop) iterate through the values for each key. These values will be the courses. Append these courses to an empty list (that you should initialize at the beginning) and RETURN that list (with the course names now in them).
I hope this helped. Best of luck and enjoy Python!
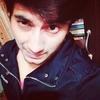
Ham Hamey
5,712 Pointsdef courses(teachers):
output = []
for courses in teachers.values():
output +=courses
return output

Jake Kobs
9,215 PointsI was so close lol. I tried appending the courses to a list and returning that, but I was returning a lists within a list. Thanks for this!
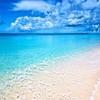
eodell
26,386 PointsMr. Ham, Thank you for this!! You saved a lot of my time.... Thank you so much!

dogancan
3,701 PointsWhy do I get always 'It should be 18' error? I gave the dict myself. It is:
dct = {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
'Kenneth Love': ['Python Basics', 'Python Collections']}
and my solution is this
def courses(d):
return list(d.values())
So, what is wrong with this code?
Thanks.
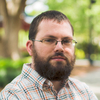
Kenneth Love
Treehouse Guest TeacherYou aren't actually using the dictionary you provide when the function runs. It uses the dictionary I give it as part of the validation test.
The reason you're getting the wrong answer, though, is that you're only combining the values as they are. For example:
>>> my_dict = {'a': [1, 2], 'b': [3, 4], 'c': [5, 6]}
>>> my_list = list(my_dict.values())
>>> my_list
[[1, 2], [3, 4], [5, 6]]
How many members does my_list
have? It only has 3. But, for the purpose of this function, I should be getting back [1, 2, 3, 4, 5, 6]
. You have to combine the contents of each key's value into a new list.

Akshay Kumar
1,098 Pointsdef num_courses (teachers): x=0 for courses in teachers.values(): for co in courses: x+=1 return x

bpietravalle
16,718 PointsThanks guys!
I finally got this to work (with some much needed outside help):
def courses(teachers):
total_courses = []
end_result = []
for key in teachers.items():
total_courses.append(teachers.values())
for courses_of_one_teacher in teachers.values():
for course in courses_of_one_teacher:
end_result.append(course)
return end_result
It seems too long for a simple task. If you have a more elegant solution, pls let me know!
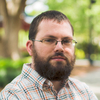
Kenneth Love
Treehouse Guest TeacherIn the teachers
dictionary, each key is a teacher and each value is a list of courses. You can get all of the values with .values()
, as you've done. So why not just extend a new list with each of the .values()
lists?
def courses(teachers):
output = []
for courses in teachers.values():
# something!
return output
I'll leave the "something" to you.

Madhu Badrikalli
Courses Plus Student 357 PointsI don't think that the first for loop is necessary

J llama
12,631 Pointsterrible style. should delete this so ppl don't get the wrong idea bro

dogancan
3,701 PointsBy saying I was giving the dict myself, I meant to say that I am actually giving a dict myself. It could have been any other dictionaries besides the ones that you gave for validation. But I chose to work with this dict, because it came handy to me.
But, when I run this code it is actually saying that 'this function returned 5 instead of 18'.I am looking for 5 as well! So where is that 18 came from?
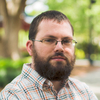
Kenneth Love
Treehouse Guest TeacherThe 18 is what the code challenge validator is looking for. When the CC is 'judged', your function is called with the dictionary I provide, and I check the contents against what I expect to get back. If the contents don't match what's expected, the task is failed.
The dictionary that your function is called with for validation has 5 keys with values. Your solution will always produce a list with as many members as there are keys in the dictionary. You just happened to test with a dictionary that has 5 items in its values. Try your code on your computer or in Workspaces. Call len(courses(dct))
and see what you get. It should give you back 2, instead of the 5 that you're expecting.

dogancan
3,701 PointsFinal question :)
What I did not understand is where this dictionary came from? Clearly the one I give (with two teachers and 5 courses) is not executed by my function, right? It is not in the code?
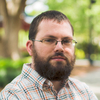
Kenneth Love
Treehouse Guest TeacherRight. Your function is called by some code that you can't see and given a dictionary that you can't see. The one at the top of the file is just an example.

dogancan
3,701 PointsOK thanks! :)
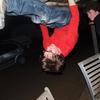
Daire Ferguson
1,592 Pointsyeah i's stuck with it saying should have returned 18 but only returned 5.... everything has worked, but its just saying i need 18 courses when i only have 5???
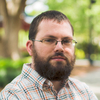
Kenneth Love
Treehouse Guest TeacherHey Daire! Sorry you're having trouble with the challenge. Can you share your code so we can work together to find the problem?

Grant Savage
960 Pointsyou were most likely using newlist.append(value). which returns results ([class 1, class 2, class 3], [class 4, class 5], ...) when you really want (class 1, class 2, class 3, class 4, class 5). Use extend(value) instead of append(value) to achieve this.
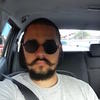
Demetrius Albuquerque
Python Web Development Techdegree Student 5,071 PointsI did that and it's working.
def courses(d):
out = []
for teacher in d:
out.extend(d[teacher])
return out
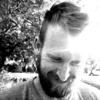
Jerehme Gayle
5,206 PointsIf anyone is still having issues with this, one thing you might try if you are using .append() to add the values to your list. Change .append() to .extend(). This website http://thomas-cokelaer.info/blog/2011/03/post-2/ is pretty black and white on why.

Vishay Bhatia
10,185 PointsCan someone please explain why this doesn't work!
def courses(teachers): course_list = [] for value in teachers.values: course_list.append(value) return course_list

ericwidman3
4,679 PointsYou're missing the () after the values in your for loop.

Surya Chandramouli
1,327 PointsHi, can someone explain how I can improve on this code? I know I'm very close but I can't seem to complete the missing point. INPUT:
def courses(something): my_list = [] for courses in something: my_list.extend(something[courses]) print( my_list)
OUTPUT:
['Python', 'Java', 'J', 'a', 'v', 'a']

Surya Chandramouli
1,327 PointsI meant to say return(my_list) in my INPUT. Sorry about that.

rd23
17,269 PointsI went with this which worked for me. Wouldn't account for redundant classes though.
def courses(dict_teachers): courseList = [] for teacher in dict_teachers: for course in dict_teachers[teacher]: courseList.append(course) return courseList

Miracle Mutoti
2,759 PointsHie rd23 i tried your code and its not working on my computer, what am i doing wrong?
Paulo Aguiar
14,120 PointsPaulo Aguiar
14,120 Pointsnot sure if this is the issue, but on this line i noticed:
while count <= len(total_courses:
it should be :
while count <= len(total_courses):