Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial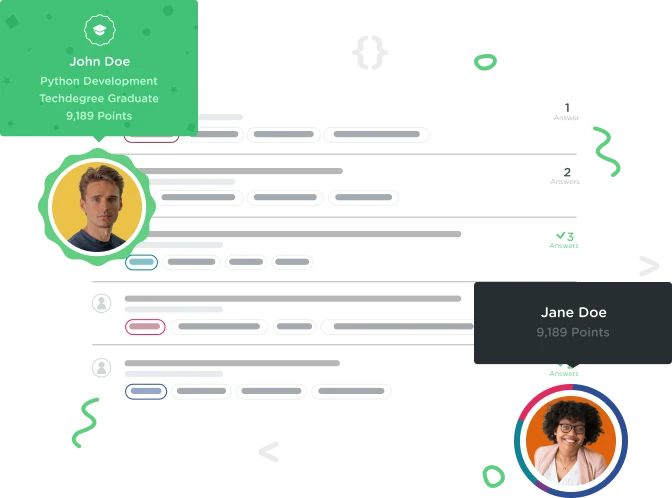

Austin Parisi
3,952 PointsWrite a function named string_factory that accepts a list of dictionaries as an argument. Return a new list of strings m
I'm getting an error that says my output should be a list. I don't understand what I need to change to make it so.
# Example:
# values = [{"name": "Michelangelo", "food": "PIZZA"}, {"name": "Garfield", "food": "lasagna"}]
# string_factory(values)
# ["Hi, I'm Michelangelo and I love to eat PIZZA!", "Hi, I'm Garfield and I love to eat lasagna!"]
template = "Hi, I'm {name} and I love to eat {food}!"
def string_factory(name=None, food=None):
if name and food:
print("Hi, I'm {} and I love to eat {}!".format(name, food))
string_factory(**{"name": "Austin", "food": ["burritos", "tacos", "pizza"]})
4 Answers

Keith Ostertag
16,619 PointsThe challenge asks to return a new list, not print it.
values = [{"name": "Michelangelo", "food": "PIZZA"}, {"name": "Garfield", "food": "lasagna"}]
template = "Hi, I'm {name} and I love to eat {food}!"
def string_factory(args):
new_list = []
for dict in args:
new_list.append(template.format(**dict))
return new_list
string_factory(values)
If you want to print out the answer in your local python environment so you can see if it works,
just substitute print(new_list)
for return new_list
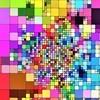
james south
Front End Web Development Techdegree Graduate 33,271 Pointsyour function does not return anything, it just prints a string. change it to return whatever strings it is asking for instead of printing a string.

Austin Parisi
3,952 PointsCan you write out the correct code? I'm so fucking lost on this stuff. I've tried 4 or 5 different things based on the suggestions I've found online, but I'm not understanding it at all.

Sherwin Shann
3,206 PointsTruly took me a lot of time to figure it out. Hope this helps:
def string_factory(dic_list):
template = "Hi, I'm {name} and I love to eat {food}!"
return_list = []
for dic in dic_list:
return_list.append("".join(template.format(**dic)))
return return_list