Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial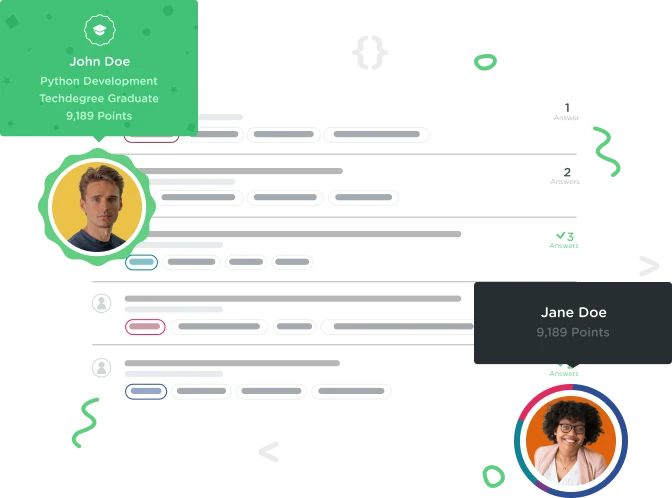
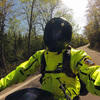
Brian Hartley
3,115 PointsWrong counts in challenge (code works locally)
I am consistently getting a 'Bummer" Didn't get the right counts for some of the words' error message. My code works as it should when I run it on my local machine (i.e. pass any string and it returns a dict with each word as a key, and their values as the number of times they appear in the string).
The problem seems to be in the challenge workspace, and not my code. Can anybody help? One example of my code is below:
def word_count(x):
d = {}
x = x.lower()
for item in x:
l = list(x.split())
for word in l:
if word not in d:
d[word] = 1
elif word in d:
d[word] = x.count(word)
return d
And another example of code that works locally, but not within the challenge workspace:
def word_count(x):
d = {}
x = x.lower()
for item in x:
l = list(x.split())
for word in l:
d[word] = x.count(word)
return d
3 Answers
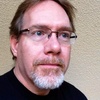
Chris Freeman
Treehouse Moderator 68,426 PointsThere are two parts I would correct:
for item in x:
l = list(x.split())
Repeatedly assigns x.split()
to l
. It can be replaced with just the assignment. split()
returns a list.
l = x.split()
The second issue is the use of x.count(word)
. For each word
it is scanning the original string and returning the count. when a duplicate word is present, it will over count those words again effective ending up with the squared count value: 2 => 4; 3 => 9.
Increment the count can be:
d[word] += 1
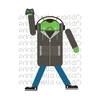
Stephen Bone
12,359 PointsHi Brian
I think the issue is with the use of the count method as it seems count to every occurrence of the letter 'i' in the string. So for example if I change the test string to "I am that I am I'm in" (I know it's nonsensical) the result I receive from your first function is {'in': 1, "i'm": 1, 'that': 1, 'am': 2, 'i': 4}.
If you just swap that line out to d[word] += 1 that fixes it.
Hope it helps!
Stephen
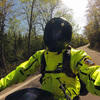
Brian Hartley
3,115 PointsChris and Steven, thanks so much for taking the time to review my question. You are absolutely right, I was miscalculating the increment. I also moved the split() outside of the for loop. Everything worked as expected.
I still wonder why the original code worked on my local machine, but that I'll have to dive into some other time.