Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial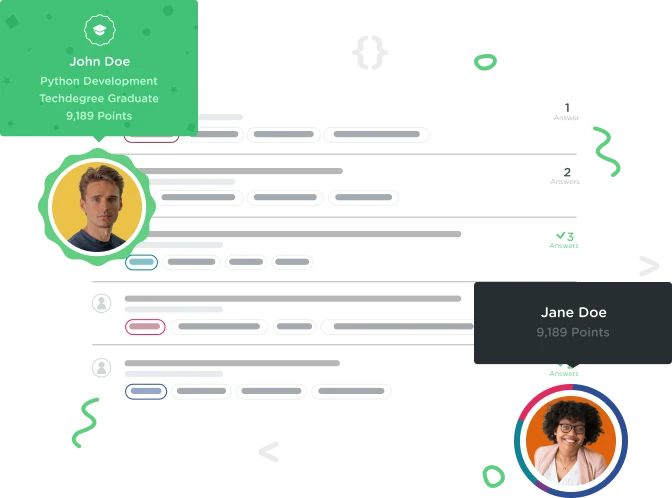

Rachel Heneault
Courses Plus Student 766 PointsYour code took too long is error I keep getting for this code: while (secret !=="seasame") {var secret = prompt("Wha");}
while (secret !=="seasame") { var secret = prompt("What is the secret password?");
}
document.write("You know the secret password. Welcome.");
while (secret !=="seasame") {
var secret = prompt("What is the secret password?");
}
document.write("You know the secret password. Welcome.");
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="app.js"></script>
</body>
</html>
3 Answers
Aaron Kaye
10,948 PointsI believe you are getting an infinite while loop because you are declaring the variable in the loop body. Try defining the variable outside the loop and just using secret = prompt .... inside the loop
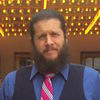
Adam Moore
21,956 PointsAaron is right. You have to define it first outside the loop, then create the loop, and inside set "secret" back to the prompt, or simply define the variable outside of the loop and set it to nothing, such as: "var secret;". This is because declaring it outside the loop is the initial creation, making the variable "secret" actually exist as a global variable (i.e., being able to be used all over your script).
If you declare it inside your while loop, then it is a local variable, and only able to be used inside it, and the while loop will begin to use the local variable as the prompt, leaving your global variable being tested in the while loop a different, global, "secret" variable than your local "secret" variable. So, the while loop checks the global variable "secret" to see if the answer matches, but since you define a local variable "secret" inside the while loop, then it is the local variable that keeps popping up a prompt. This makes it to where you are never given a second chance to type in the correct password into your global "secret" prompt variable, so the loop continues to run and run and run forever, as the initial "secret" variable that you started out testing in your while loop is set only to your very first password given.
So, if you were to type "dog" instead of "sesame" into the first prompt, then the while loop runs (because "dog" is not equal to "sesame"). So then the loop pops up a new prompt with a local variable declared and assigned to it also called "secret", and then whatever you type in that prompt gets assigned to the local variable "secret". So, if you type in "sesame" into the prompt that gets popped up inside the while loop, then that means your global variable "secret" is set to "dog", and your local variable "secret" is set to "sesame".
Well, the while loop is set up to check only the global variable, so the while loop runs again to see what your global variable "secret" is set to, and it's still equal to "dog", because you haven't changed it, so the loop runs again, popping up another prompt to set a local variable of "secret" again, all the while never changing your global variable "secret" to anything other than what you initially set it to be, so the loop will run forever, prompt after prompt after prompt.
If you declared the variable OUTSIDE the loop, then set the global variable to the prompt INSIDE the loop, WITHOUT the "var" keyword (as that's what creates a local variable), then the while loop will then set the global variable "secret" to the prompt, and then when you type another password into the prompt, you are changing the global variable "secret", which is what the while loop is attempting to test. THEN, if you type "sesame" into the prompt, you are setting the global variable "secret" to "sesame", and the while loop runs again, testing if the global variable "secret" is equal to "sesame" (which, if it ISN'T equal, the while loop runs), and since it IS equal to "sesame", the while loop does NOT run, and the next line of code runs, which is your "document.write" code.
Also, I would suggest NOT setting the global variable "secret" outside of the while loop to anything, but simply declare it like "var secret;" because of not having to repeat yourself, which is very important rule of programming shorthanded as DRY (Don't Repeat Yourself).
Hope this explanation helps. I know it's kind of long-winded, but a greater understanding of what is going on under the hood can never hurt :)
Aaron Kaye
10,948 PointsSolid Answer!

Jason Anello
Courses Plus Student 94,610 PointsHi Rachel,
The reason that the code timed out is because you have a typo. You spelled "sesame" as "seasame". The tester is trying to set secret
to "sesame" in order to stop the loop but the condition continues to remain true.
Once you fix that problem your code is fine. However, you will get a new error message.
" Bummer! Don't use the var
keyword inside the loop. You only need to declare the secret
variable once, at the beginning of the script."
It's not that anything is wrong but the challenge is trying to get you to follow the recommended practice of declaring variables at the top of their scope.
Javascript has something called hoisting. When you write your code like this:
while (secret !=="sesame") {
var secret = prompt("What is the secret password?");
}
document.write("You know the secret password. Welcome.");
It's treated as if you wrote it like this:
var secret;
while (secret !=="sesame") {
secret = prompt("What is the secret password?");
}
document.write("You know the secret password. Welcome.");
Notice that the prompt assignment still remains inside the loop but the variable declaration was hoisted to the top of the code.
This new error message is simply saying that instead of relying on this implicit hoisting you should explicitly declare your variables at the top. Either at the top of a function or at the top of the global code.
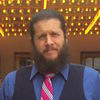
Adam Moore
21,956 PointsI didn't know about hoisting, so thanks, Jason! Also, I thought I had tried it that way when I was piddling around with this bit of code, and it didn't work for me, so I must have had some spelling or syntax error somewhere in my own code.