Heads up! To view this whole video, sign in with your Courses account or enroll in your free 7-day trial. Sign In Enroll
- Contact Class: Part 1 6:05
- Create a Contact Class 1 objective
- Contact Class: Part 2 4:04
- Write a Method 1 objective
- Phone Number Class 6:15
- Write a to_s Method 1 objective
- Address Class: Part 1 5:00
- Address Class: Part 2 3:44
- Initializing and Calling Methods 2 objectives
- Address Book Class 4:25
- Instance variable instantiation 2 objectives
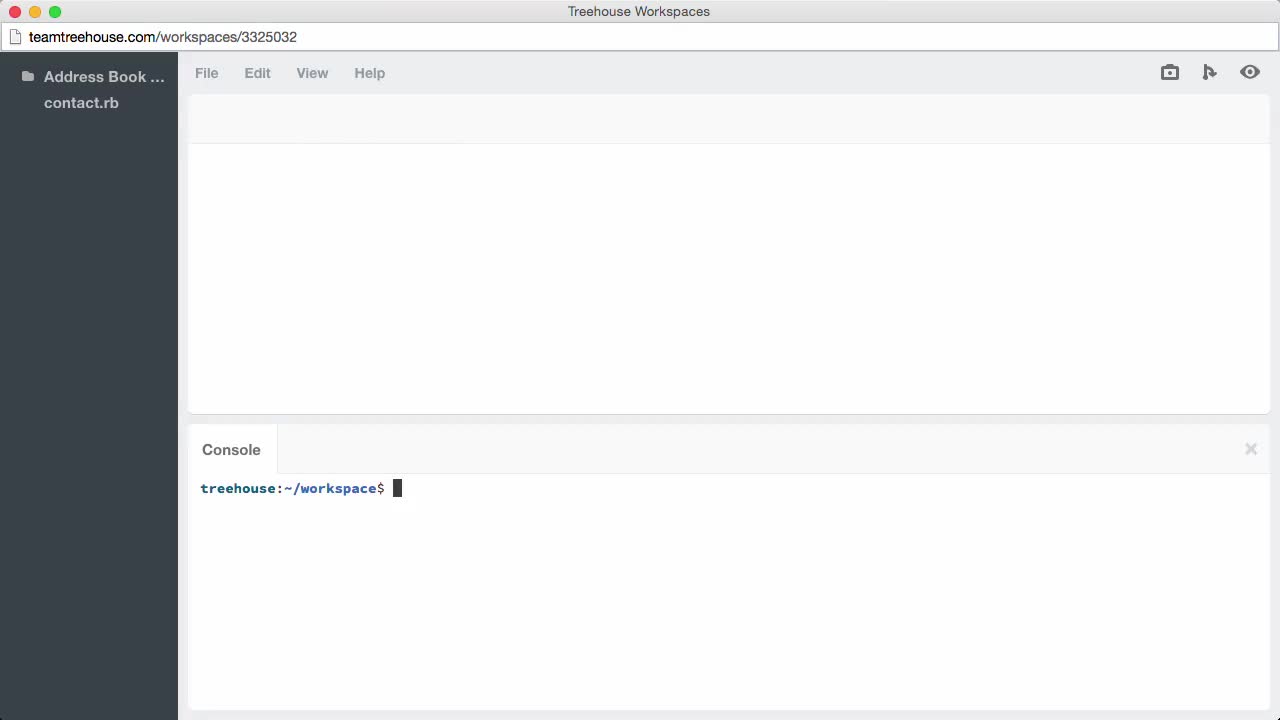
- 2x 2x
- 1.75x 1.75x
- 1.5x 1.5x
- 1.25x 1.25x
- 1.1x 1.1x
- 1x 1x
- 0.75x 0.75x
- 0.5x 0.5x
In this video, we're going to create a class that will store our phone numbers.
Code Samples
Phone Number Class (phone_number.rb):
class PhoneNumber
attr_accessor :kind, :number
def to_s
"#{kind}: #{number}"
end
end
Contact Class
require "./phone_number"
class Contact
attr_writer :first_name, :middle_name, :last_name
attr_reader :phone_numbers
def initialize
@phone_numbers = []
end
def add_phone_number(kind, number)
phone_number = PhoneNumber.new
phone_number.kind = kind
phone_number.number = number
phone_numbers.push(phone_number)
end
def first_name
@first_name
end
def middle_name
@middle_name
end
def last_name
@last_name
end
def first_last
first_name + " " + last_name
end
def last_first
last_first = last_name
last_first += ", "
last_first += first_name
if !@middle_name.nil?
last_first += " "
last_first += middle_name.slice(0, 1)
last_first += "."
end
last_first
end
def full_name
full_name = first_name
if !@middle_name.nil?
full_name += " "
full_name += middle_name
end
full_name += ' '
full_name += last_name
full_name
end
def to_s(format = 'full_name')
case format
when 'full_name'
full_name
when 'last_first'
last_first
when 'first'
first_name
when 'last'
last_name
else
first_last
end
end
def print_phone_numbers
puts "Phone Numbers"
phone_numbers.each { |phone_number| puts phone_number }
end
end
jason = Contact.new
jason.first_name = "Jason"
jason.last_name = "Seifer"
jason.add_phone_number("Home", "123-456-7890")
jason.add_phone_number("Work", "456-789-0123")
puts jason.to_s('full_name')
jason.print_phone_numbers
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up-
Jonathan Hartmayer
5,626 Points0 Answers
-
MaryAnn Eleanya
8,626 Points1 Answer
-
Muhammad Saif
11,565 Points1 Answer
-
Ben Flores
17,074 Points1 Answer
-
Immo Struchholz
10,515 Points3 Answers
-
arnaud
13,859 Points3 Answers
-
Michael Hulet
47,913 Points2 Answers
-
Nick Stoneman
10,093 Points3 Answers
View all discussions for this video
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up
You need to sign up for Treehouse in order to download course files.
Sign upYou need to sign up for Treehouse in order to set up Workspace
Sign up