Heads up! To view this whole video, sign in with your Courses account or enroll in your free 7-day trial. Sign In Enroll
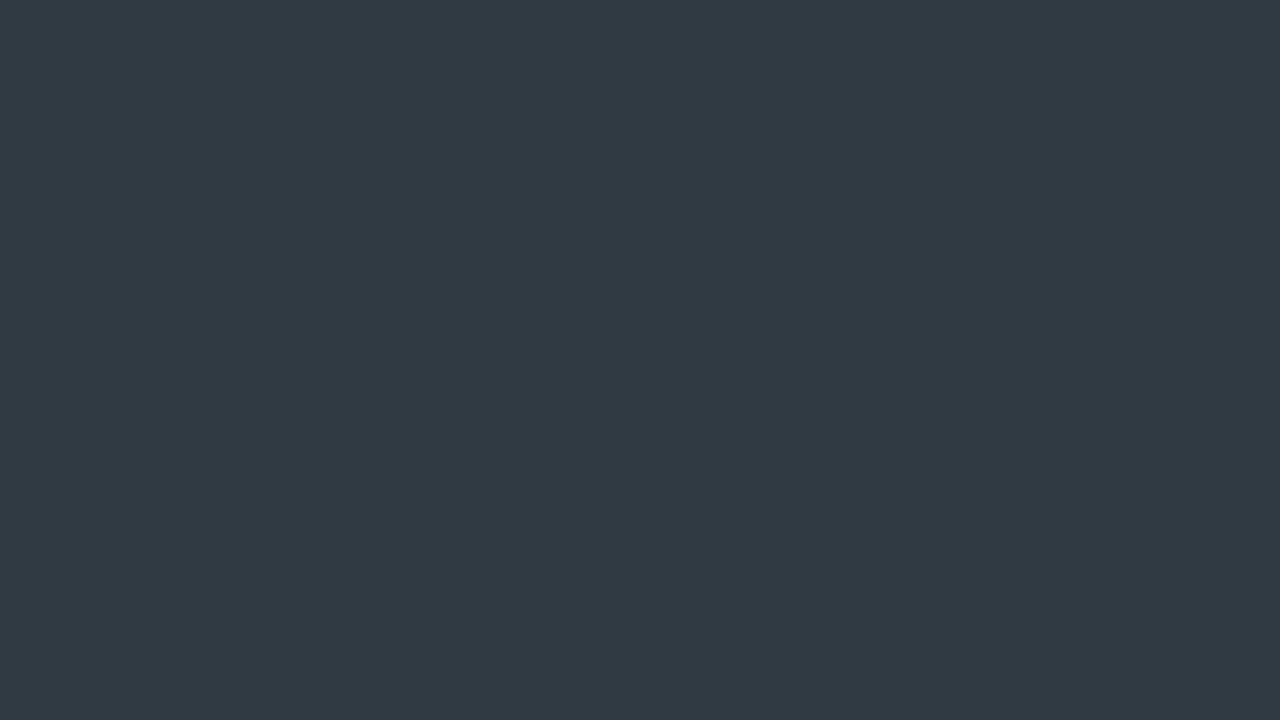
- 2x 2x
- 1.75x 1.75x
- 1.5x 1.5x
- 1.25x 1.25x
- 1.1x 1.1x
- 1x 1x
- 0.75x 0.75x
- 0.5x 0.5x
Review what makes a class object, and what happens when a class is instantiated.
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up-
Carlo Hogeveen
10,584 Points0 Answers
-
nermien barakat
1,623 Points0 Answers
-
Libor Gess
6,880 Points2 Answers
-
PLUS
tal Shnitzer
Courses Plus Student 5,242 Points1 Answer
-
jlampstack
23,932 Points1 Answer
-
jlampstack
23,932 Points3 Answers
-
jlampstack
23,932 Points1 Answer
-
jlampstack
23,932 Points2 Answers
-
M Glasser
10,868 Points1 Answer
-
christian gamboa
10,097 Points1 Answer
-
cyber voyager
8,859 Points2 Answers
-
PLUS
Nick Trabue
Courses Plus Student 12,666 Points1 Answer
-
Brendan Moran
14,052 Points3 Answers
-
Dotun Ogunsakin
13,475 Points1 Answer
-
joseph arias
9,269 Points2 Answers
-
Sergi Oca
7,981 Points3 Answers
View all discussions for this video
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up
You need to sign up for Treehouse in order to download course files.
Sign upYou need to sign up for Treehouse in order to set up Workspace
Sign up