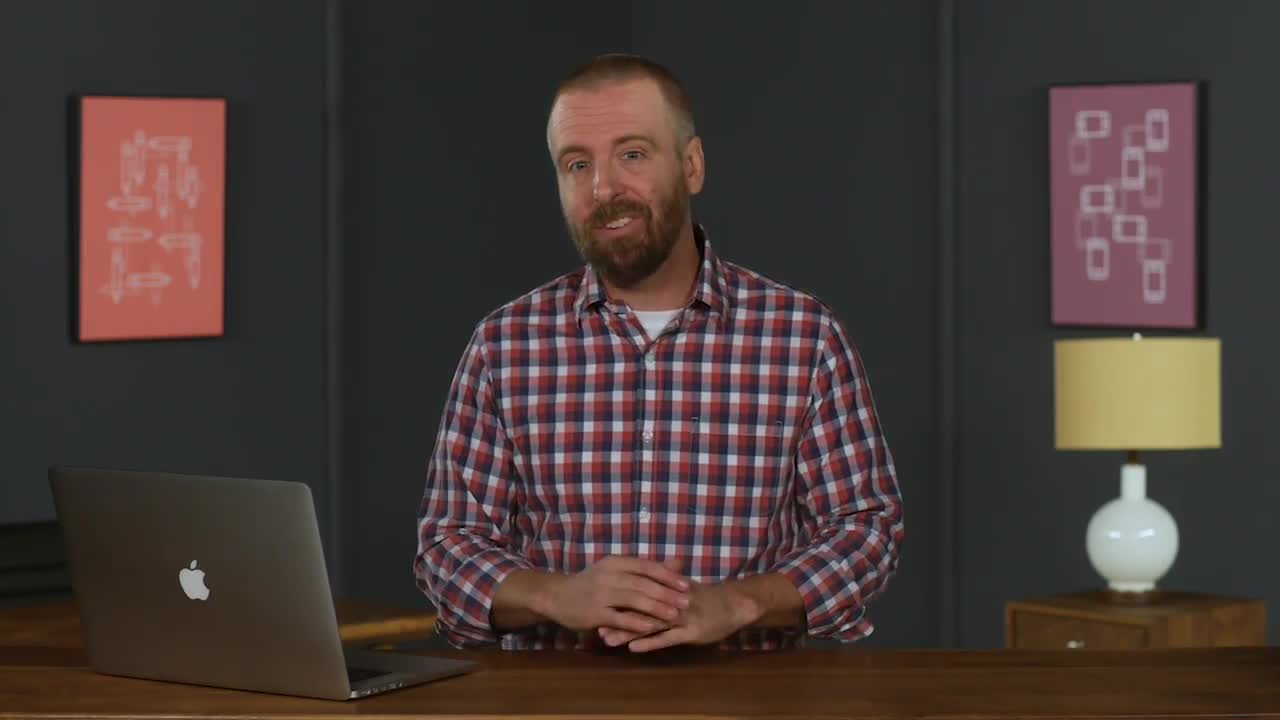
- 2x 2x
- 1.75x 1.75x
- 1.5x 1.5x
- 1.25x 1.25x
- 1.1x 1.1x
- 1x 1x
- 0.75x 0.75x
- 0.5x 0.5x
In this video we will explore the development environment we will be using for the rest of the course, Workspaces. We will write, compile and run a command line program that will introduce ourselves.
It's not an error!
- Not to worry, the
Picked up JAVA_TOOL_OPTIONS: -Xmx128m
is not an error on your part, we recently just tweaked our setup to have more memory allocated. Don't fret that mine looks different!
Additional Content
- Learn more about the command line in the Console Foundations course
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign upRelated Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up[MUSIC] 0:00 Hello and welcome. 0:04 I'm Craig, and I'm a developer. 0:05 For this course, it doesn't matter if you have any programming experience at all. 0:07 I'll be introducing you to some very common tools available in 0:12 almost every programming language that you might encounter. 0:15 We'll be doing our exercises in Java, and I'd like you to approach this 0:19 as if you were attempting to learn a foreign language. 0:23 I'll introduce you to the rules or syntax of the language as you need it, 0:26 we will also discuss the oddities that you might encounter. 0:30 As we go through different parts of this course, 0:33 you'll start to recognize words and concepts in other parts of the language 0:36 much like you would in a language immersion program. 0:40 Just like when you're wandering around a foreign country where you're just 0:43 learning the language, you'll come across words and 0:46 phrases that you aren't familiar with and that's okay. 0:48 Focus on the topics we're covering and don't worry about the other parts. 0:52 As we're cruising along, I might ask you to ignore some things, but 0:56 I promise we'll get back to it when the time is right. 0:59 You might not have noticed it yet but there is speed controls on your video. 1:02 Please feel free to speed me up and make a pretty good chipmunk or 1:06 slow me way down as you feel necessary. 1:09 I won't take any offense. 1:14 I promise. 1:15 Also, I've added helpful links in the teacher's notes 1:16 that will allow you to learn even more, sound good? 1:19 So without further ado, let's get started with our task at hand. 1:23 Just like most foreign language classes, 1:27 the first thing we're going to learn is how to introduce ourselves in code. 1:29 So, what we'll do is we'll write out or print, hello my name is, and 1:34 then your name, to the screen. 1:38 Speaking of introductions, 1:40 allow me to introduce you to where we'll be coding during this course, Workspaces. 1:42 If you click the launch Workspaces button right next to this video, 1:47 you'll see a new browser open with our programing environment. 1:50 I'll head over there with you. 1:53 So this is a Workspace, and it's all yours. 1:56 You'll see up here that there's a tabbed interface of files that you have open. 2:00 And over here is a list of files that you have available to you. 2:04 I've gone and created a file and 2:07 got it all set up with a nice starting point to begin writing our application. 2:08 It's called Introductions.java. 2:12 Let's open it up and take a peek. 2:13 I've left a note or a comment right here. 2:18 Comments in Java start with two forward slashes, 2:22 everything after the slash was ignored by Java. 2:25 So they're used by programmers to explain what their code is trying to accomplish in 2:27 clear English or to leave notes for other programmers. 2:31 Now one goal of programming is for your code to be as clear as possible, so 2:34 that it doesn't require comments. 2:38 However, as we're getting started here feel free to leave comments whenever you 2:39 want to remind yourself of what it is that we're attempting to do. 2:43 Now, remember how I warned you that you'll come across words or 2:46 phrases that you aren't familiar with and that I might ask you to 2:49 ignore some parts of code until later, we're at one of those points. 2:52 Every Java program has some setup that needs to happen and for now, 2:56 I've done that for you. 2:59 But don't worry about understanding most of the pre-written code that's on 3:00 the screen, it's just the setup that we need to do to start writing our own code. 3:03 However, the one bit of code I've written that I want you to take a closer look at 3:07 is this line right here. 3:11 But don't worry exactly about how this code works. 3:14 What you need to know is that it creates a Java object, which we'll talk about later, 3:17 which has a method that allows us to write out 3:21 text to the terminal which is down here. 3:23 I'd like to take a quick break and already acknowledge, as well as remind you that I 3:26 just threw out a mouthful of new terms like objects and methods and like I said, 3:30 don't worry, we'll cover them in much greater detail in the following course. 3:34 But for now, I just want you to continue immersing yourself in this language. 3:38 I'll explain the concepts when they're important to the task at hand. 3:42 So like I said thanks to the setup I've done, we have access to the console object. 3:45 We can use this console object to print text to the screen. 3:50 Objects like console have methods that let them perform actions, and 3:53 the action that we want to do right now is print some text to the screen. 3:57 Console's method for printing text on the screen is called printf, so let's use it. 4:01 First we'll type console, and 4:07 then a period to access its methods, then the word printf. 4:09 We're gonna call the method by doing open parenthesis. 4:14 Now we're gonna do double quotes and write the text out that we wanna write, so 4:17 we're gonna say, hello my name is Craig. 4:21 We're gonna end the double quote, and then we're gonna end the parenthesis and 4:24 then we're gonna put a semicolon to signify that the statement is complete. 4:28 You might have noticed that as I edited the file there's a little dot up here 4:32 signifying that the file has been edited. 4:36 I'm going to go ahead and I'm going to save from the file, 4:38 I mean I'm going to click File, and I'm going to click Save and 4:41 you'll notice that in the future you can use Cmd+S or Ctrl+S on a window. 4:43 Okay, so let's see our code in action. 4:48 Now, Java is a compiled language, 4:50 which means that we have to run a compiler program to turn our human readable Java 4:52 code into computer readable code before we run it. 4:56 So let's compile our introductions program. 4:59 To do that at the console, we type javac and 5:02 then the name of the file which is Introductions.Java. 5:07 Java C is short for Java compiler. 5:14 So this line is telling the Java compiler to convert or compile 5:17 the code in the file Introductions.Java into an executable file. 5:20 So I'm going to press Enter. 5:26 And if we take a look at the files in this directory using the LS command, 5:29 see that there is now a file called Introductions.class. 5:33 By the way, I should mention here that LS stands for list, and 5:39 it allows you to list files in a directory. 5:42 We're gonna use a few terminal commands on this course, and 5:45 I'll teach you everything you need to know for it. 5:48 But if you wanna learn more about using the terminal, check out our 5:50 course called Console Foundations which is linked in the teacher's notes. 5:52 Now to run our program, we simply type java Introductions. 5:56 Now notice the command that I type here is java, not javac. 6:04 Java runs the program it only needs the name of the class, without the extension. 6:09 Javac required that .java, and Java does not. 6:14 So I wanna press Enter. 6:18 And here on the screen we see our output, the introduction. 6:20 Great job. 6:24 We now have our first compiled program. 6:25 You've been learning a ton. 6:28 Before we move on and learn even more, 6:30 let's do an exercise to review some of the things we've learned thus far. 6:32 Before we get there, let me just remind you that there is a community that you can 6:36 and should lean on while going through this course. 6:40 If you have questions, it's likely someone else has them too and 6:44 has maybe even answered them. 6:47 Learning how to ask questions and 6:50 find answers is in my personal opinion the best way to learn anything. 6:51 If you're coming to this course with some Java experience, 6:56 you might have used an IDE or Integrated Development Environment. 6:59 Now those are a set of tools which greatly simplify the development 7:03 process through code completion and many other bells and whistles. 7:06 As handy as IDEs are, 7:11 I'd like to request that you still follow along in the Workspace. 7:13 As I think there's benefit in learning and gaining confidence in doing things 7:17 all by yourself and removing some of the magic of that IDE. 7:21 We'll go over IDEs in a future course when the time is right. 7:24 Okay, now back to that exercise. 7:28
You need to sign up for Treehouse in order to download course files.
Sign upYou need to sign up for Treehouse in order to set up Workspace
Sign up