Heads up! To view this whole video, sign in with your Courses account or enroll in your free 7-day trial. Sign In Enroll
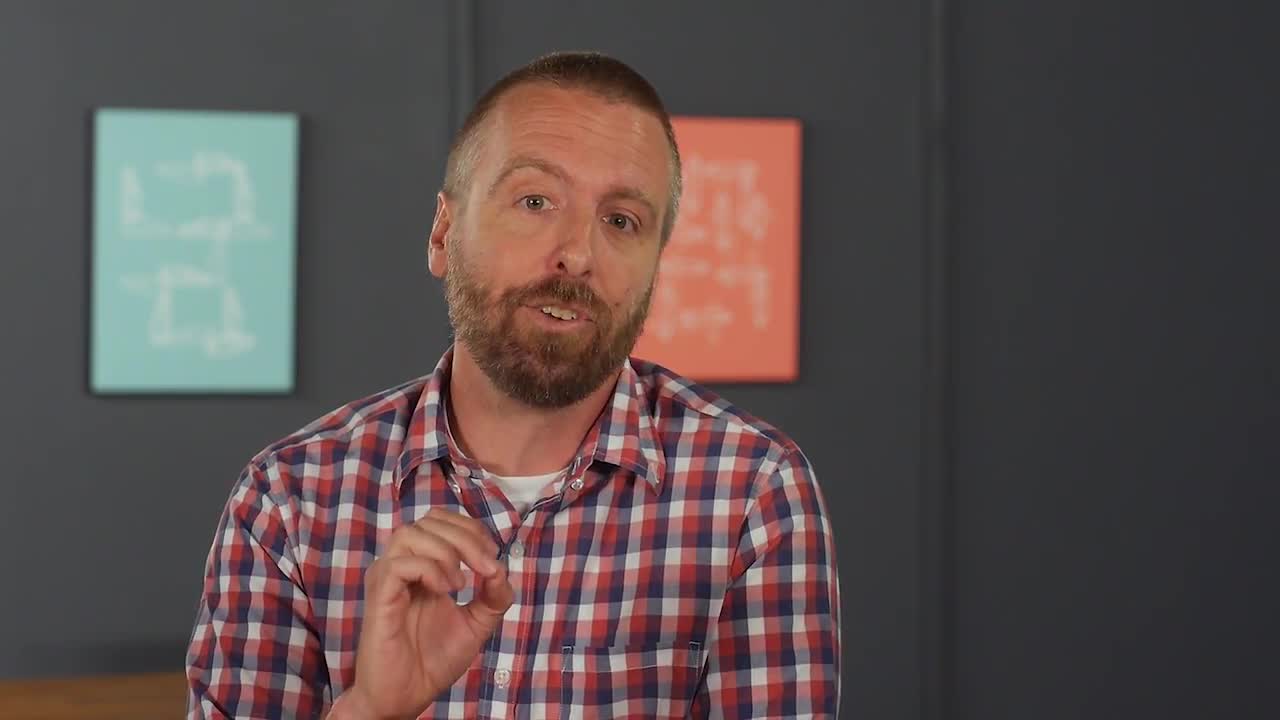
- 2x 2x
- 1.75x 1.75x
- 1.5x 1.5x
- 1.25x 1.25x
- 1.1x 1.1x
- 1x 1x
- 0.75x 0.75x
- 0.5x 0.5x
In this video we will review Single Abstract Methods or SAMs and how things were done prior to Java 8.
Read More
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up-
Chance Edward
4,507 Points0 Answers
-
Travis Sly
3,947 Points0 Answers
-
PLUS
Jaslynn Terry
Courses Plus Student 2,166 Points1 Answer
-
Ronnie N
Full Stack JavaScript Techdegree Student 102 Points1 Answer
-
k doshi
128 Points1 Answer
-
Diwakar Singh
785 Points1 Answer
-
Apurva Trehan
77 Points1 Answer
-
Unsubscribed User
48,988 Points5 Answers
-
Phillip Hurst
4,204 Points1 Answer
View all discussions for this video
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up
You need to sign up for Treehouse in order to download course files.
Sign upYou need to sign up for Treehouse in order to set up Workspace
Sign up