Heads up! To view this whole video, sign in with your Courses account or enroll in your free 7-day trial. Sign In Enroll
- What is jQuery? 3:10
- jQuery: History and Relevance Today 2:51
- jQuery vs. JavaScript 6:02
- Review: What is jQuery? 5 questions
- Animating Elements with jQuery 7:43
- jQuery Syntax and Animation Effects 2 objectives
- Blog Previewer: Changing Content Inside Elements 7:13
- Using text() and html() 3 objectives
- Blog Previewer: Getting Values from Form Fields 6:06
- Getting Values from Form Fields 2 objectives
- Introduction to jQuery Review 5 questions
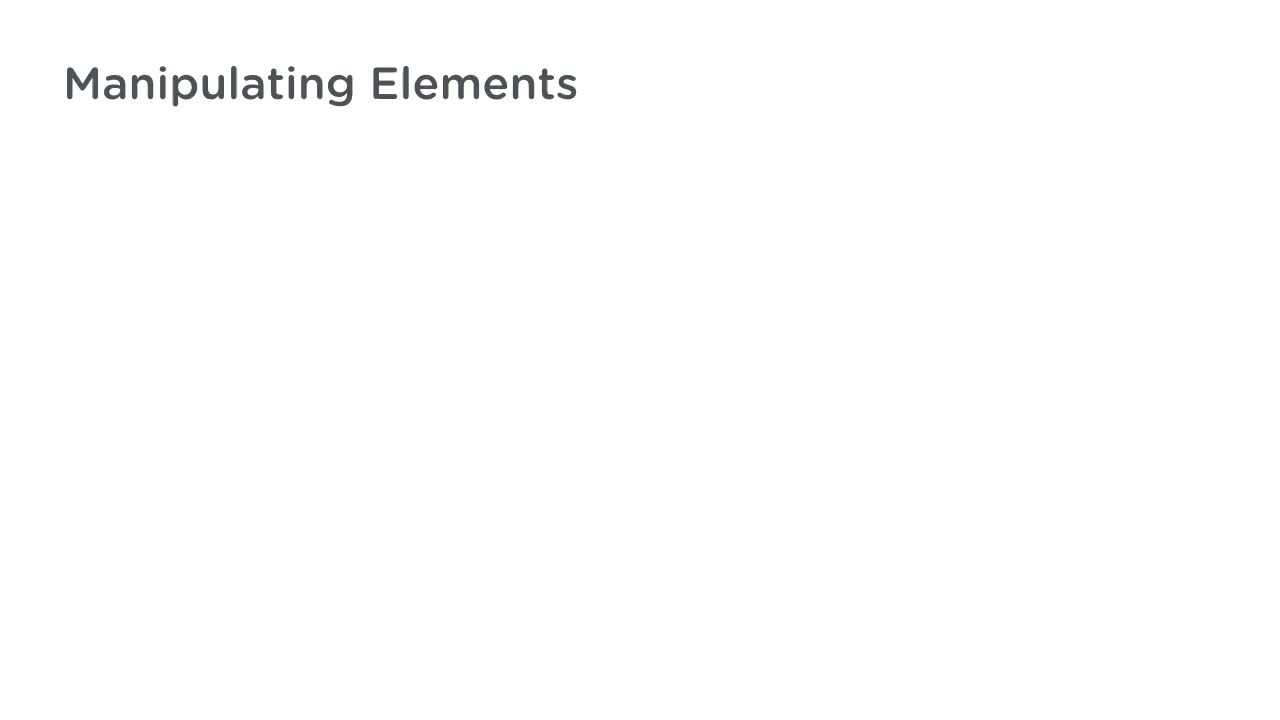
- 2x 2x
- 1.75x 1.75x
- 1.5x 1.5x
- 1.25x 1.25x
- 1.1x 1.1x
- 1x 1x
- 0.75x 0.75x
- 0.5x 0.5x
Create simple, fun animation sequences with jQuery!
Further Reading
- jQuery Docs: Animation Effects
- Method chaining
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up-
Lanai Lewis
10,400 Points2 Answers
-
PLUS
Ian Salmon
Courses Plus Student 10,687 Points1 Answer
-
Aakash Srivastav
Full Stack JavaScript Techdegree Student 11,638 Points2 Answers
-
babasariffodeen
6,475 Points1 Answer
-
Jesse Hunter
12,531 Points4 Answers
View all discussions for this video
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up
You need to sign up for Treehouse in order to download course files.
Sign upYou need to sign up for Treehouse in order to set up Workspace
Sign up