Heads up! To view this whole video, sign in with your Courses account or enroll in your free 7-day trial. Sign In Enroll
- Adding jQuery to a Project 2:43
- Spoiler Revealer: Breaking it Down 6:28
- Understanding Unobtrusive JavaScript 4:38
- Adding New Elements to the DOM 5:06
- Progressive Enhancement and Unobtrusive JavaScript 5 questions
- Adding New Elements to the DOM 2 objectives
- Using on() for Event Handling 2:26
- Using Events with Dynamically Added Elements 4:41
- The Event Object 4:44
- Events with jQuery 2 objectives
- What is Traversal? 8:42
- DOM Traversal with jQuery 2 objectives
- jQuery Events and DOM Traversal Review 5 questions
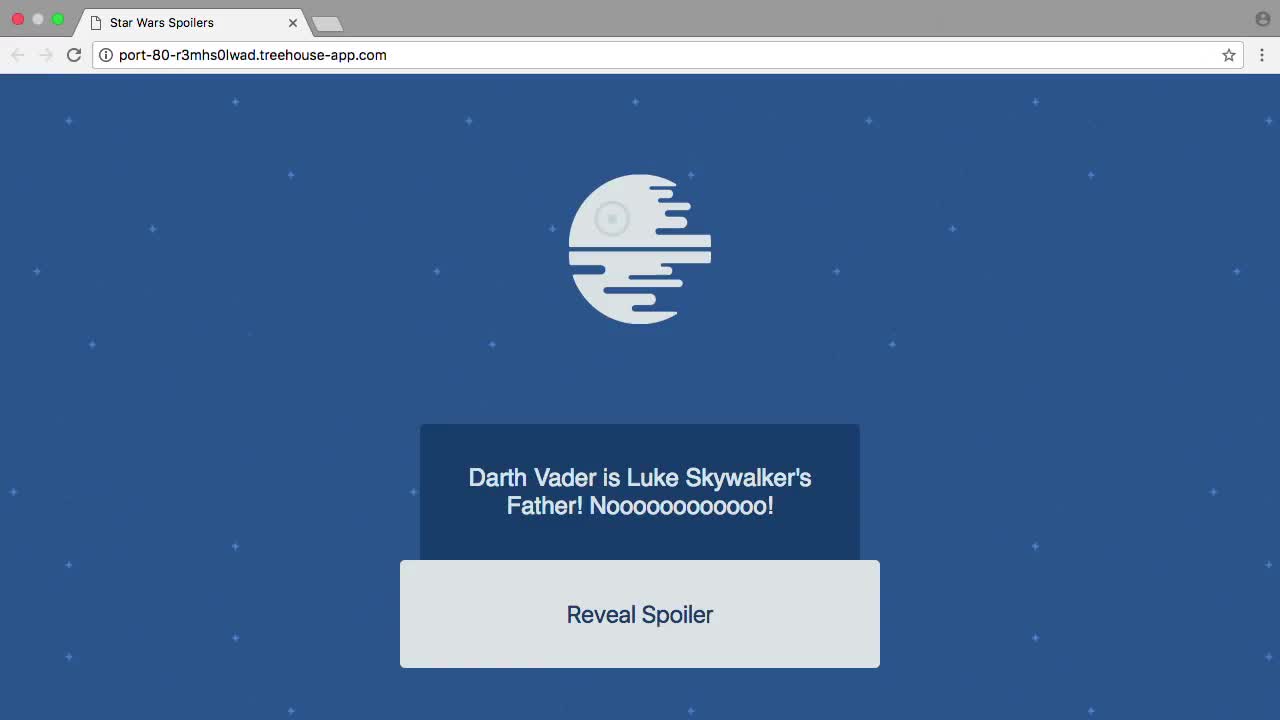
- 2x 2x
- 1.75x 1.75x
- 1.5x 1.5x
- 1.25x 1.25x
- 1.1x 1.1x
- 1x 1x
- 0.75x 0.75x
- 0.5x 0.5x
We start planning our spoiler revealer and learn a bit about using descendant selectors with jQuery.
Click event listener example:
$( "#target" ).click(function() {
alert( "I've been clicked!" );
});
Further Reading
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up-
Riley O'Neil
2,560 Points0 Answers
-
Sam Alcaraz
6,637 Points4 Answers
-
PLUS
africgheorgheaugustin
Courses Plus Student 8,180 Points3 Answers
-
Iver Band
8,389 Points7 Answers
-
David Lacho
20,864 PointsSplit second where spoiler is shown before app.js loaded
Posted by David LachoDavid Lacho
20,864 Points3 Answers
-
Ioana Rusu
9,599 Points2 Answers
-
Ikuyasu Usui
36 Points2 Answers
View all discussions for this video
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up
You need to sign up for Treehouse in order to download course files.
Sign upYou need to sign up for Treehouse in order to set up Workspace
Sign up