Heads up! To view this whole video, sign in with your Courses account or enroll in your free 7-day trial. Sign In Enroll
Preview
Video Player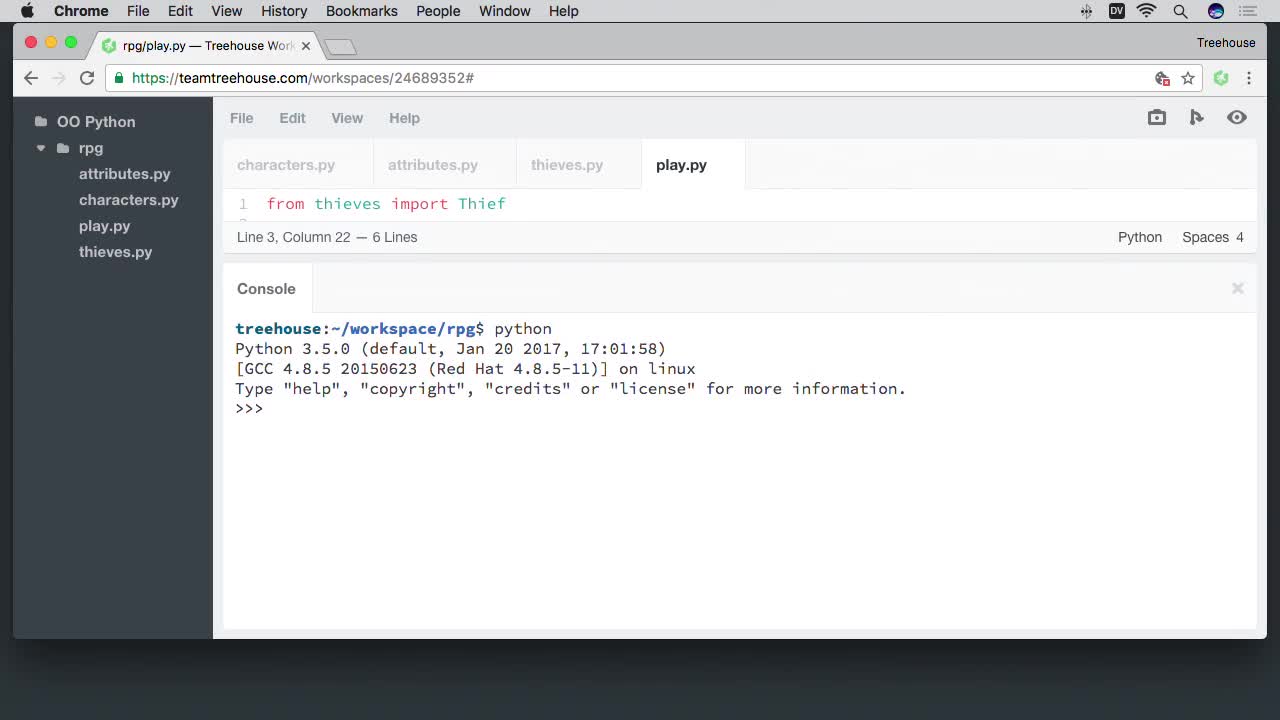
00:00
00:00
00:00
- 2x 2x
- 1.75x 1.75x
- 1.5x 1.5x
- 1.25x 1.25x
- 1.1x 1.1x
- 1x 1x
- 0.75x 0.75x
- 0.5x 0.5x
Being able to identify object classes and types is a really useful ability.
-
isinstance(<object>, <class>)
- This function will tell you whether or not<object>
is an instance of<class>
. If it could be an instance of several classes, you can use a tuple of classes like so:isinstance(<object>, (<class1>, <class2>, <class3>))
. -
issubclass(<class>, <class>)
- This function will tell you whether or not one class is a descendent of another class. Just likeisinstance()
, you can use a tuple of classes to compare against. -
type(<instance>)
will give you the class for an instance, as willinstance.__class__
. Neither of these is particularly useful.
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up-
Alan Azizov
2,066 Points1 Answer
-
Kurobe Kuro^T_T^
5,369 Points1 Answer
View all discussions for this video
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up
So far we've been building new classes and
using classes to add attributes and
0:00
methods to our custom classes.
0:04
Let's take a break from creating
classes though and look at a couple of
0:05
useful functions for identifying what
kind of objects we're working with.
0:08
So, these functions aren't necessarily
something you're going to use every day or
0:12
even something you're gonna
wanna use all the time.
0:16
They are, however, really handy when
you need to act a certain way or
0:19
an object,
depending on what kind of object it is.
0:22
If Python is duck typed,
0:25
consider this to be how you separate
the mallards from the mandarins.
0:27
So I'm gonna hop into Python, and
0:30
I'm just gonna show what these do because
they're really fun and interactive.
0:32
The first is probably the one that
you're going to use the most often.
0:37
This function isinstance
0:39
tells you whether or not something is
an instance of a particular class.
0:42
And the string 'a' does
happen to be a string.
0:47
You can use it with multiple types, too.
0:51
So we can say, isinstance,
and let's get the float 5.2,
0:53
and then inside of a new tuple
we can say int or float, and
0:58
we get back True because it
is either an int or float.
1:03
It's a float.
1:05
We can also do isinstance 5.2,
is that a string, a boolean, or a dict?
1:07
No, it's not.
1:16
This also does expose a pretty fun
little fact from Python's past.
1:18
Cuz you can do isinstance True,
and int, and it is.
1:22
I'll leave it up to you to figure
out why or how that came about.
1:28
The second function is issubclass,
issubclass.
1:32
And this one tells you whether or
1:38
not a particular class is
a subclass of another class.
1:39
Like, isinstance,
this can also take a list of classes, or
1:43
rather a couple of classes
to compare against.
1:46
So, (bool, int) True issubclass(str,
1:48
int) False, okay?
1:55
And we can use that to check
the tree of our own classes, too.
1:58
I'm gonna use Ctrl l here,
to clear the screen.
2:02
And I'm gonna say from
thieves import Thief.
2:04
And then I'm gonna say from
characters import Character.
2:11
And then, I'm gonna say issubclass
is Thief is subclass of Character.
2:16
True, since our Thief class
inherits from the Character class,
2:23
our Thief is a subclass of Character,
of course you already knew that.
2:26
The last two items are a bit less useful
but they're still good to know about.
2:31
Firstly, we have the type function.
2:35
Now, type can be used for
some trickier behavior, but
2:37
let's just focus on using it to
get information about an instance.
2:40
So I'm gonna make a new Thief, and
2:43
remember I have to put in
the name argument now.
2:47
And I'm going to say type for kenneth.
2:51
And I get that kenneth is a Thief.
2:54
Now, we already knew that, right?
2:56
But, it's great that Python can tell us.
2:59
The type function tells you the type
of object that an instance is.
3:01
Generally, you'll want to use isinstance,
if you're wanting to know if I whether or
3:05
not to work with an instance though, since
it will check the full inheritance tree.
3:08
If I was to use this,
to find out whether or
3:12
not kenneth was a Character,
I wouldn't know.
3:14
I only know that kenneth is a Thief.
3:17
Now, we've used the __init__
to control how it was created.
3:19
Python's classes have tons
of these magic methods and
3:25
we're gonna talk about several
of them in the next stage.
3:27
Python classes also have a lot
of magic attributes too, and
3:30
one of those is the class attribute.
3:34
It'll tell you what class an instance is.
3:36
So we can say kenneth.__class__ and
get back our thieves.Thief.
3:40
And we can even go a step further and get
the magic name attribute off of the class.
3:47
Kenneth.__class__.__name__, and
that tells us Thief.
3:53
So if you wanted to write some code,
then inspect at an instance's class name,
3:59
you could do it.
4:03
While Python is heavily slanted toward
duck typing, where if an object quacks
4:06
like a duck and waddles like a duck
should be considered a duck.
4:09
I mean, if you can use a method on
an object and not get an exception,
4:12
you should use it that way.
4:15
It's still really handy to be able to
tell if something is an instance of
4:17
a particular class or not.
4:20
You'll find yourself using these functions
and attributes when you get into code
4:21
that operates based on the type
of arguments it's working with.
4:24
Often referred to metaprograming or
introspective programming.
4:27
Both of those are beyond the scope of
this course, but feel free to look
4:31
into them yourself, they're very
interesting ways of programming.
4:34
All right, take a break if you need it,
get a snack if you're hungry,
4:37
do code challenges and
4:40
quizzes to cement all of this knowledge
in your head, then come back for
4:41
the next stage where we'll get our hands
dirty with Python's built in classes.
4:44
Ever wanted dot notation on a dictionary?
4:48
You need to sign up for Treehouse in order to download course files.
Sign upYou need to sign up for Treehouse in order to set up Workspace
Sign up