Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial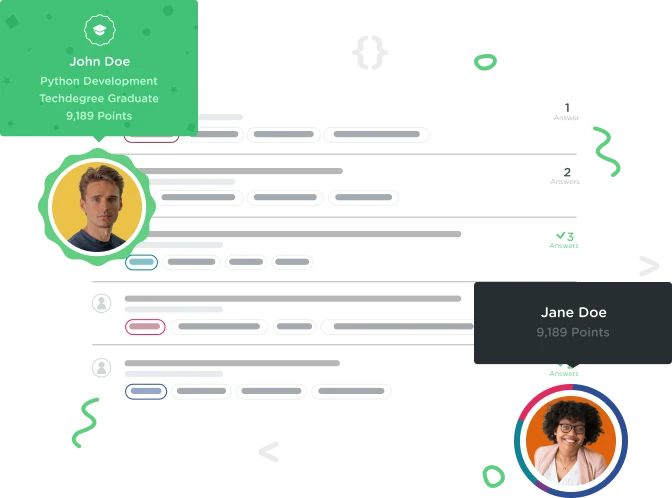
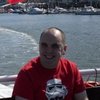
Sean Flanagan
33,236 PointsMy address book doesn't work like Jason's
Hi. Does anyone know why my address book functions differently from Jason's? I've checked the code sample and nothing seems to be wrong. Here's my address_book.rb:
require "./contact"
class AddressBook
attr_reader :contacts
def initialize
@contacts = []
end
def run
loop do
puts "Address Book"
puts "a: Add Contact"
puts "p: Print Address Book"
puts "e: Exit"
print "Enter your choice: "
input = gets.chomp.downcase
case input
when 'a'
add_contact
when 'p'
print_contact_list
when 'e'
break
end
end
end
def add_contact
contact = Contact.new
print "First name: "
contact.first_name = gets.chomp
print "Middle name: "
contact.middle_name = gets.chomp
print "Last name: "
contact.last_name = gets.chomp
loop do
puts "Add phone number or address? "
puts "p: Add phone number"
puts "a: Add address"
puts "(Any other key to go back)"
response = gets.chomp.downcase
case response
when "p"
phone = PhoneNumber.new
print "Phone number kind (Home, Work, etc): "
phone.kind = gets.chomp
print "Number: "
phone.number = gets.chomp
contact.phone_numbers.push(phone)
when "a"
address = Address.new
print "Address Kind (Home, Work, etc): "
address.kind = gets.chomp
print "Address line 1: "
address.street_1 = gets.chomp
print "Address line 2: "
address.street_2 = gets.chomp
print "City: "
address.city = gets.chomp
print "State: "
address.state = gets.chomp
print "Postal Code: "
address.postal_code = gets.chomp
contact.addresses.push(address)
else
print "\n"
break
end
end
contacts.push(contact)
end
def print_results(search, results)
puts search
results.each do |contact|
puts contact.to_s('full_name')
contact.print_phone_numbers
contact.print_addresses
puts "\n"
end
end
def find_by_name(name)
results = []
search = name.downcase
contacts.each do |contact|
if contact.full_name.downcase.include?(search)
results.push(contact)
end
end
print_results("Name search results (#{search})", results)
end
def find_by_phone_number(number)
results = []
search = number.gsub("-", "")
contacts.each do |contact|
contact.phone_numbers.each do |phone_number|
if phone_number.number.gsub("-", "").include?(search)
results.push(contact) unless results.include?(contact)
end
end
end
print_results("Phone search results (#{search})", results)
end
def find_by_address(query)
results = []
search = query.downcase
contacts.each do |contact|
contact.addresses.each do |address|
if address.to_s('long').downcase.include?(search)
results.push(contact) unless results.include?(contact)
end
end
end
print_results("Address search results (#{search})", results)
end
def print_contact_list
puts "Contact List"
contacts.each do |contact|
puts contact.to_s('last_first')
end
end
end
address_book = AddressBook.new
address_book.run
Thanks in advance for any help rendered. :)
3 Answers

Ben Jakuben
Treehouse TeacherHi Sean--what behavior are you seeing? I launched the Workspace for this video and pasted your code in and it looked like it was working the same way Jason demonstrated in the video.
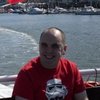
Sean Flanagan
33,236 PointsMy apologies in advance if I've panicked unnecessarily. This is the output I got:
Enter your choice: a
First name: Sean
Middle name: Michael
Last name: Flanagan
Add phone number or address?
p: Add phone number
a: Add address
(Any other key to go back)
p
Phone number kind (Home, Work, etc): 07777 777777
Number: 07777 777777
Add phone number or address?
p: Add phone number
a: Add address
(Any other key to go back)
p
Phone number kind (Home, Work, etc): Mobile
Number: 07777 777777
Add phone number or address?
p: Add phone number
a: Add address
(Any other key to go back)
a
Address Kind (Home, Work, etc): Home
Address line 1: 1 Oak Lane
Address line 2: Friedland
City: Richmond
State: Virginia
Postal Code: 2222
Add phone number or address?
p: Add phone number
a: Add address
(Any other key to go back)
Address Book
a: Add Contact
p: Print Address Book
e: Exit
Enter your choice: p
Contact List
Flanagan, Sean M.
Address Book
a: Add Contact
p: Print Address Book
e: Exit
Enter your choice: p
Contact List
Flanagan, Sean M.
Address Book
a: Add Contact
p: Print Address Book
e: Exit
Enter your choice:

Ben Jakuben
Treehouse TeacherThat's all the code does at this point. :) Keep going in the course and you'll add search, which prints more details. As of right now, entering 'p' causes this code to run, which only prints the contact's name:
def print_contact_list
puts "Contact List"
contacts.each do |contact|
puts contact.to_s('last_first')
end
end
You can modify that to print more details if you'd like! You could add these two lines:
contact.print_phone_numbers
contact.print_addresses
just like in the print_results
method. You could even create a new helper method to format how you print a contact's information and then call it from both print_results
and the loop in print_contact_list
.
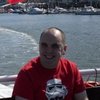
Sean Flanagan
33,236 PointsHi Ben. I've just watched the video again and I'm positive that I get the picture now.
Thank you! :)
Sean Flanagan
33,236 PointsSean Flanagan
33,236 PointsHi Ben. Thanks for your reply. There weren't actually any bugs. I'm trying the program out as I type so please bear with me.
This is what it says now:
Is that supposed to happen?
Ben Jakuben
Treehouse TeacherBen Jakuben
Treehouse TeacherYep! Check out the video around the 3:30 mark to see how it works for Jason, or take a look at your code line by line to try to predict the behavior. So when you enter "a", it matches the
when "a"
line and executes this code: