Heads up! To view this whole video, sign in with your Courses account or enroll in your free 7-day trial. Sign In Enroll
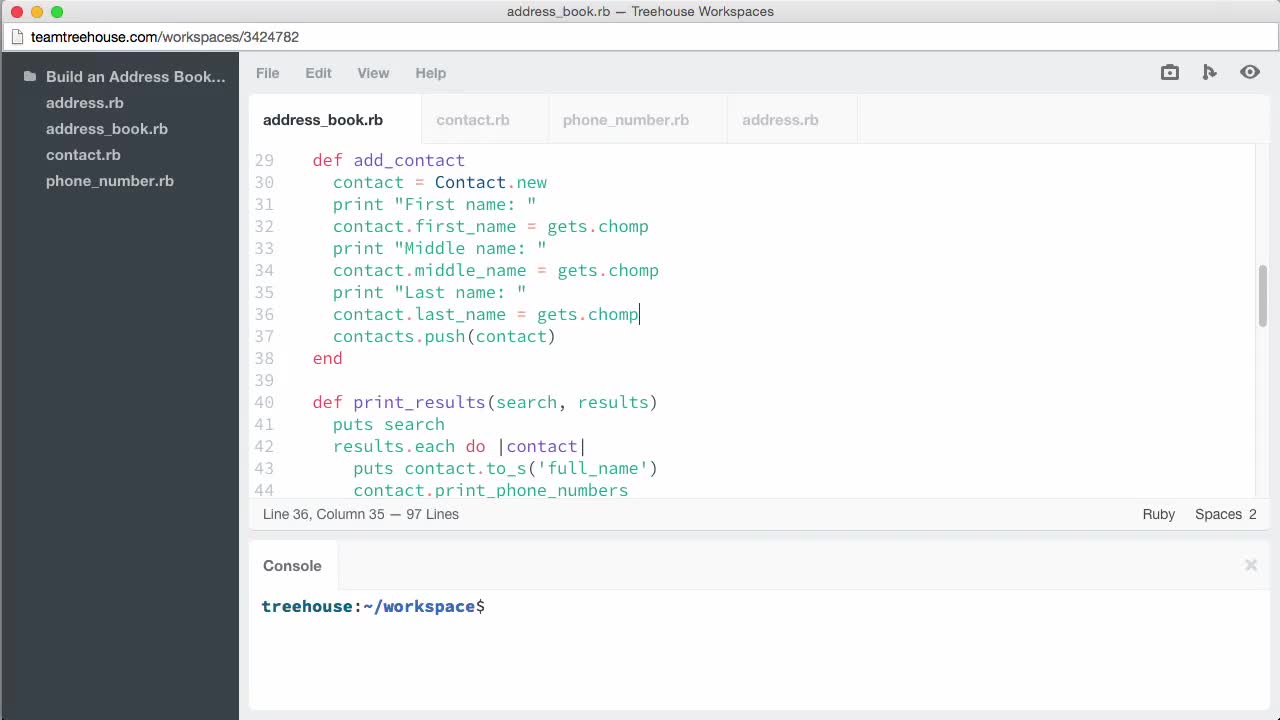
- 2x 2x
- 1.75x 1.75x
- 1.5x 1.5x
- 1.25x 1.25x
- 1.1x 1.1x
- 1x 1x
- 0.75x 0.75x
- 0.5x 0.5x
Our address book functionality is getting there. In this video, we implement the ability to add contacts via the command line.
Code Samples
def add_contact
contact = Contact.new
print "First name: "
contact.first_name = gets.chomp
print "Middle name: "
contact.middle_name = gets.chomp
print "Last name: "
contact.last_name = gets.chomp
loop do
puts "Add phone number or address? "
puts "p: Add phone number"
puts "a: Add address"
puts "(Any other key to go back)"
response = gets.chomp.downcase
case response
when 'p'
phone = PhoneNumber.new
print "Phone number kind (Home, Work, etc): "
phone.kind = gets.chomp
print "Number: "
phone.number = gets.chomp
contact.phone_numbers.push(phone)
when 'a'
address = Address.new
print "Address Kind (Home, Work, etc): "
address.kind = gets.chomp
print "Address line 1: "
address.street_1 = gets.chomp
print "Address line 2: "
address.street_2 = gets.chomp
print "City: "
address.city = gets.chomp
print "State: "
address.state = gets.chomp
print "Postal Code: "
address.postal_code = gets.chomp
contact.addresses.push(address)
else
print "\n"
break
end
end
contacts.push(contact)
end
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up-
Daniel Grieve
6,432 PointsTerminal doesn't register a non-nil value for middle name.
Posted by Daniel GrieveDaniel Grieve
6,432 Points0 Answers
-
Jorge Rodriguez
2,616 Points2 Answers
-
Loi Tran
9,053 Pointsadding phone number or address yields multiple contact adds
Posted by Loi TranLoi Tran
9,053 Points0 Answers
-
Sean Flanagan
33,236 Points3 Answers
View all discussions for this video
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up
You need to sign up for Treehouse in order to download course files.
Sign upYou need to sign up for Treehouse in order to set up Workspace
Sign up