Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial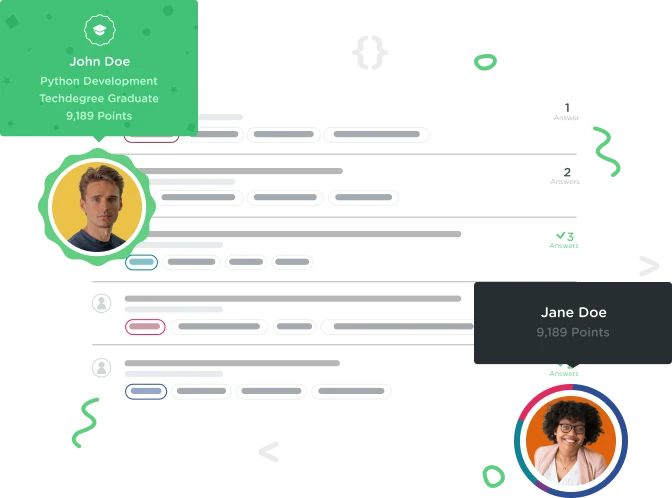

Sean Conolly
19,110 PointsC# Streams and Data Processing - Challenge Task 1 of 3
I am doing the course:
C# Streams and Data Processing
I'm stuck on part four 'Working with Date Time'.
I feel the instructions for the task are a bit too long winded.
"Create a static method named ParseWeatherForecast that takes a string[] parameter named values and returns a WeatherForecast. Instantiate a WeatherForecast variable named weatherForecast and assign the appropriate value in the values array to the WeatherStationId property. Use the sample data shown in WeatherForecast.cs to determine which value in the array is the WeatherStationId. Don't forget to return the weatherForecast in the new method!"
I have the code:
using System; using System.IO;
namespace Treehouse.CodeChallenges { public class Program { public static void Main(string[] arg) {
}
static string ParseWeatherForecast(string[] values) { var weatherForecast = new WeatherForecast(); //string values = line.Split(','); weatherForecast.WeatherStationId = DateTime.Parse(values[0]); DateTime.TryParse(values[0], out weatherForecast)
return weatherForecast; }
}
}
Any advice would be appreciated.
Thanks, Sean
3 Answers
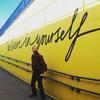
Matthew Hill
7,799 PointsHi Sean,
I can only agree with you that this is a very wordy challenge. There are a number of ways of doing this but many of the code challenges like you to only solve something in a partiuclar way. It took me a few tries to figure out what was wanted and I got this to work:
using System;
using System.IO;
namespace Treehouse.CodeChallenges
{
public class Program
{
public static void Main(string[] arg)
{
}
public static WeatherForecast ParseWeatherForecast(string[] values)
{
WeatherForecast weatherForecast = new WeatherForecast();
weatherForecast.WeatherStationId = values[0];
return weatherForecast;
}
}
}
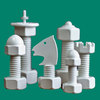
Steven Parker
241,970 PointsYou still did not provide a link to the challenge page.
So this is still a bit of a guess, but just going from the quoted challenge text, I notice where they say to "Create a static method named ParseWeatherForecast that ...returns a WeatherForecast" — and while you have done that inside the method, your declaration indicates that the return value will be a string.
Also, the TryParse line seems redundant since the previous line parsed and assigned the value, but worse than that, it is being passed weatherForecast as the 2nd argument, where it would be expecting a DateTime.
If you wanted to use TryParse instead of Parse, refer to my suggestion in your previous question.

Sean Conolly
19,110 PointsHere is a link to the challenge page:
https://teamtreehouse.com/library/c-streams-and-data-processing/parsing-data/working-with-datetime
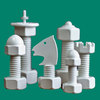
Steven Parker
241,970 PointsLooks like all my guesses above were good.
In addition, now that I've seen the rest of the challenge, WeatherStationId is defined as a string. So it can be assigned directly from the values array without parsing.
And finally, remember to use the public access modifier with the method declaration.

Sean Conolly
19,110 PointsThanks Steven,
So what would the code be then. Still having trouble working it out.
Thanks, Sean
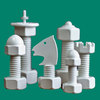
Steven Parker
241,970 PointsChanging your definition so that it returns the correct type and has public access:
public static WeatherForecast ParseWeatherForecast(string[] values)
Assigning WeatherStationId:
weatherForecast.WeatherStationId = values[0];
None of the Parse or TryParse stuff is needed.

Carel Du Plessis
Courses Plus Student 16,356 Pointsusing System;
using System.IO;
namespace Treehouse.CodeChallenges
{
public class Program
{
public static void Main(string[] arg)
{
}
public static WeatherForecast ParseWeatherForecast(string[] values)
{
WeatherForecast weatherForecast = new WeatherForecast();
weatherForecast.WeatherStationId = values[0];
var timeOfDay = new DateTime();
if (DateTime.TryParse(values[1], out timeOfDay))
{
weatherForecast.TimeOfDay = timeOfDay;
}
return weatherForecast;
}
}
}
using System;
/* Sample CSV Data
weather_station_id,time_of_day,condition,temperature,precipitation_chance,precipitation_amount
HGKL8Q,06/11/2016 0:00,Rain,53,0.3,0.03
HGKL8Q,06/11/2016 6:00,Cloudy,56,0.08,0.01
HGKL8Q,06/11/2016 12:00,PartlyCloudy,70,0,0
HGKL8Q,06/11/2016 18:00,Sunny,76,0,0
HGKL8Q,06/11/2016 19:00,Clear,74,0,0
*/
namespace Treehouse.CodeChallenges
{
public class WeatherForecast
{
public string WeatherStationId { get; set; }
public DateTime TimeOfDay { get; set;}
}
}

Carel Du Plessis
Courses Plus Student 16,356 Pointsmy answer to the challenge after looking for the answers around the web and treehouse community.
Sean Conolly
19,110 PointsSean Conolly
19,110 PointsThanks for your help Matthew. That worked great.
Steven Parker
241,970 PointsSteven Parker
241,970 PointsThese are the same changes I had already given you!